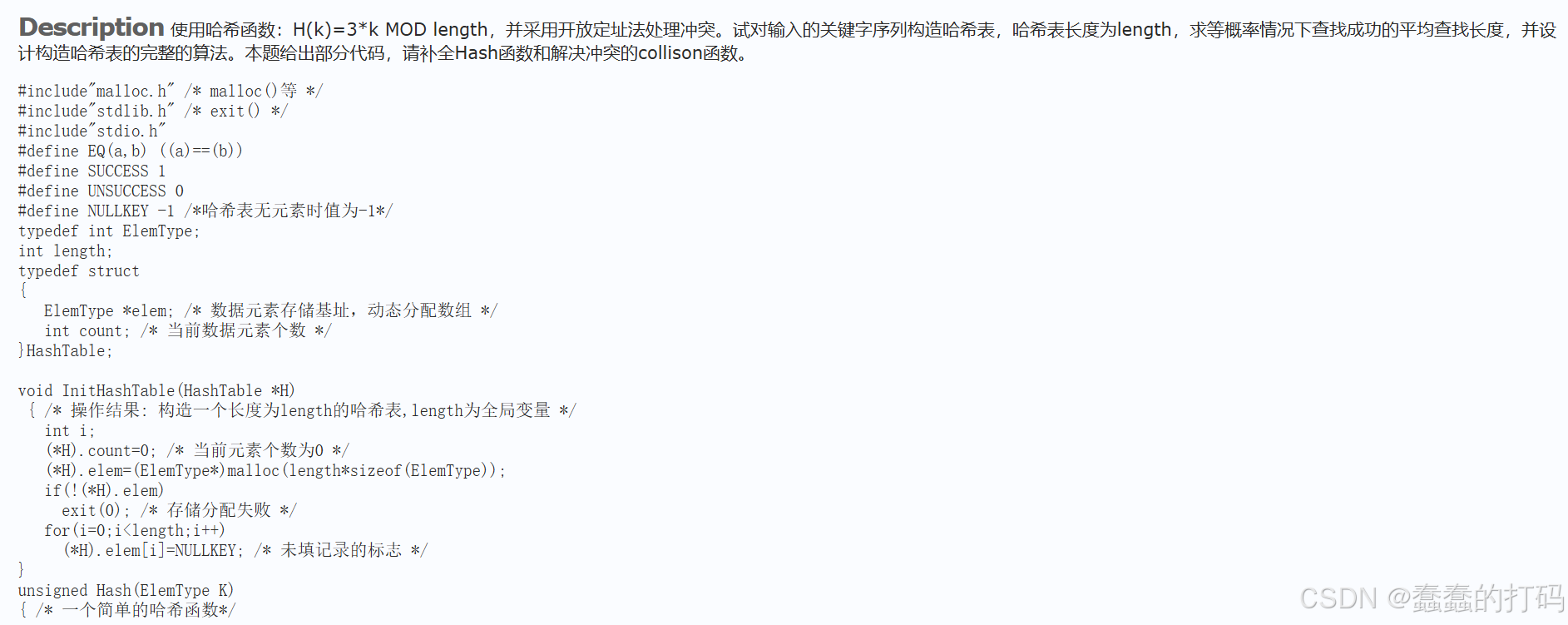
**思路:**
-
初始化哈希表,长度为 `length`。
-
使用哈希函数 `H(k) = 3 * k % length` 计算哈希地址。
-
采用线性探测法处理冲突。
-
插入关键字序列到哈希表中。
-
计算平均查找长度。
**伪代码:**
-
初始化哈希表 `H`,长度为 `length`。
-
定义哈希函数 `Hash`。
-
定义冲突处理函数 `collision`。
-
定义查找函数 `SearchHash`。
-
定义插入函数 `InsertHash`。
-
读取输入的哈希表长度 `length`。
-
读取关键字序列,插入哈希表。
-
输出哈希表内容。
-
计算并输出平均查找长度。
**C++代码:**
#include "malloc.h" /* malloc()等 */
#include "stdlib.h" /* exit() */
#include "stdio.h"
#define EQ(a,b) ((a)==(b))
#define SUCCESS 1
#define UNSUCCESS 0
#define NULLKEY -1 /*哈希表无元素时值为-1*/
typedef int ElemType;
int length;
typedef struct {
ElemType *elem; /* 数据元素存储基址,动态分配数组 */
int count; /* 当前数据元素个数 */
} HashTable;
void InitHashTable(HashTable *H) {
int i;
(*H).count = 0; /* 当前元素个数为0 */
(*H).elem = (ElemType*)malloc(length * sizeof(ElemType));
if (!(*H).elem)
exit(0); /* 存储分配失败 */
for (i = 0; i < length; i++)
(*H).elem[i] = NULLKEY; /* 未填记录的标志 */
}
unsigned Hash(ElemType K) {
return (3 * K) % length;
}
void collision(int *p) {
*p = (*p + 1) % length; /* 线性探测再散列 */
}
int SearchHash(HashTable H, ElemType K, int *p, int *c) {
*p = Hash(K); /* 求得哈希地址 */
while (H.elem[*p] != NULLKEY && !EQ(K, H.elem[*p])) {
(*c)++;
if (*c < length)
collision(p); /* 求得下一探查地址p */
else
break;
}
if EQ(K, H.elem[*p])
return SUCCESS; /* 查找成功,p返回待查数据元素位置 */
else
return UNSUCCESS; /* 查找不成功(H.elem[p].key==NULLKEY),p返回的是插入位置 */
}
int InsertHash(HashTable *H, ElemType e) {
int c, p;
c = 0;
if (SearchHash(*H, e, &p, &c)) /* 表中已有与e有相同关键字的元素 */
printf("哈希表中已有元素%d。\n", e);
else { /* 插入e */
(*H).elem[p] = e;
++(*H).count;
}
return c + 1; /*查找长度为冲突次数加1*/
}
void TraverseHash(HashTable H) {
int i;
for (i = 0; i < length; i++)
if (H.elem[i] == NULLKEY) /* 有数据 */
printf("X ");
else
printf("%d ", H.elem[i]);
printf("\n");
}
int main() {
float i = 0, j = 0;
ElemType e;
HashTable H;
scanf("%d", &length);
InitHashTable(&H);
scanf("%d", &e);
while (e != -1) {
j++; /*j记录输入元素个数*/
i = i + InsertHash(&H, e); /*i记录查找长度的和*/
scanf("%d", &e);
}
TraverseHash(H);
printf("Average search length=%f\n", i / j);
return 0;
}
**总结:**
-
使用哈希函数 `H(k) = 3 * k % length` 计算哈希地址。
-
采用线性探测法处理冲突。
-
插入关键字序列到哈希表中,并计算平均查找长度。