MyBatis-Plus 使用入门指南
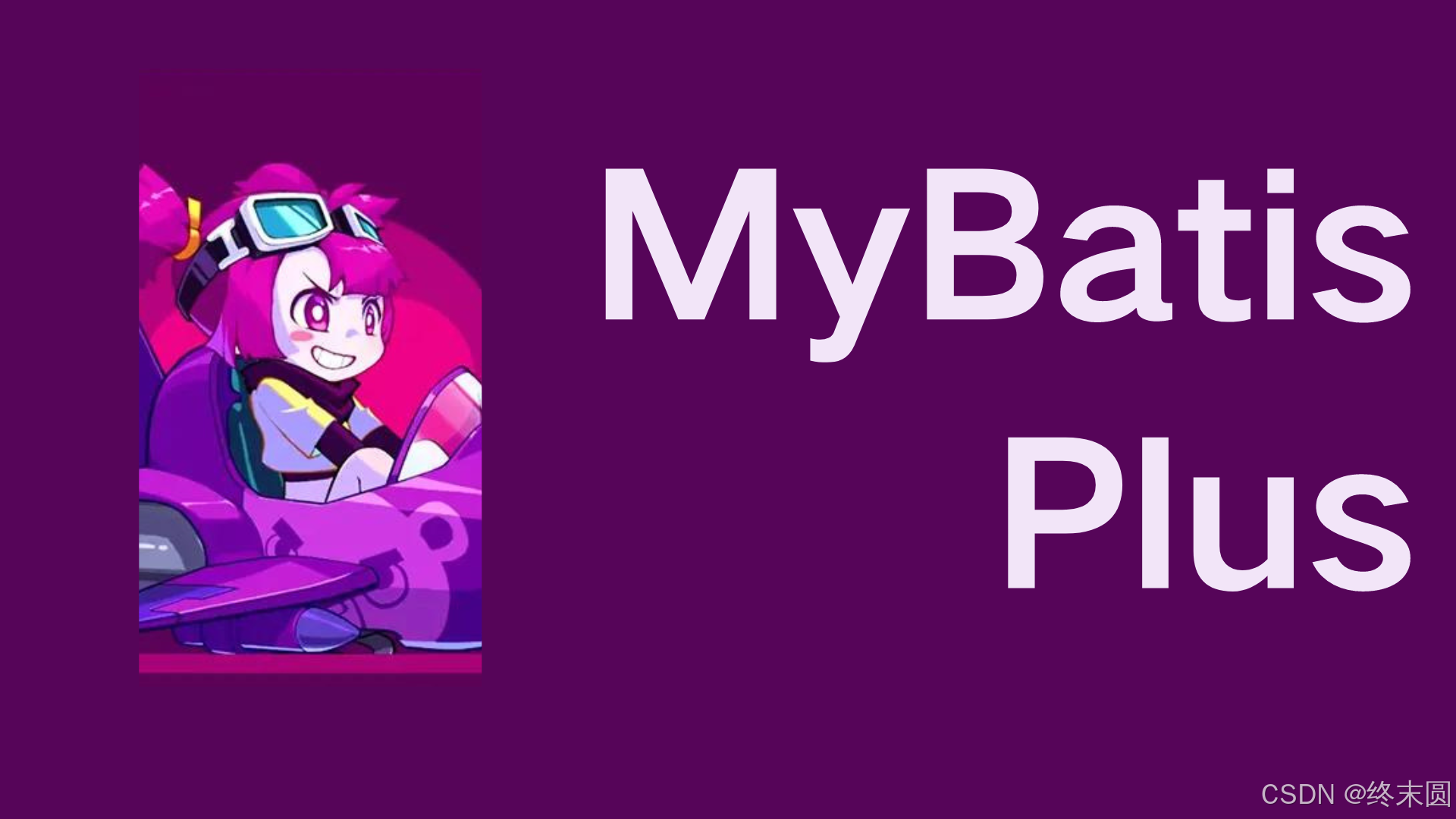
前言
在Java的持久层框架中,MyBatis因其灵活性和易用性而广受欢迎。然而,随着项目规模的扩大,MyBatis的一些重复性工作(如CRUD操作)开始显得繁琐。为了解决这一问题,MyBatis-Plus应运而生。MyBatis-Plus(简称MP)是在MyBatis基础上增强的工具,在MyBatis的基础上只做增强不做改变,为简化开发、提高效率而生。
一、MyBatis-Plus简介
MyBatis-Plus是一个MyBatis的增强工具,在MyBatis的基础上只做增强不做改变,为简化开发、提高效率而生。它内置了强大的CRUD操作,以及条件构造器(Wrapper),使得开发者能够以更少的代码完成数据库操作。
二、MyBatis-Plus的核心特性
- 强大的CRUD操作:无需编写繁琐的Mapper XML文件,MyBatis-Plus提供了内置的CRUD操作。
- 条件构造器:通过Wrapper(如QueryWrapper、UpdateWrapper等)构造复杂的查询条件,无需编写大量的SQL语句。
- 分页插件:内置分页插件,轻松实现分页功能。
- 性能分析插件:自动分析SQL执行性能,帮助开发者优化SQL。
- 乐观锁插件:支持乐观锁,解决并发更新问题。
- 多租户SQL解析器:支持多租户SQL解析,为SaaS应用提供数据隔离支持。
三、MyBatis-Plus使用步骤
1. 添加依赖
首先,你需要在项目的pom.xml
文件中添加MyBatis-Plus的依赖。以下是一个使用Spring Boot和MyBatis-Plus的示例依赖:
xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>你的版本号</version>
</dependency>
请替换你的版本号
为当前MyBatis-Plus的最新稳定版本。
2. 配置数据源
在application.yml
或application.properties
中配置数据源信息,例如:
yaml
spring:
datasource:
url: jdbc:mysql://localhost:3306/your_database?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=UTC
username: root
password: your_password
driver-class-name: com.mysql.cj.jdbc.Driver
3. 实体类
创建实体类,并使用MyBatis-Plus提供的注解来标识主键、表名等。
java
import com.baomidou.mybatisplus.annotation.TableName;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.Id;
@TableName("your_table")
public class YourEntity {
@Id(type = IdType.AUTO)
private Long id;
// 其他字段
// getters and setters
}
4. Mapper接口
创建Mapper接口,继承BaseMapper<YourEntity>
,无需编写任何方法,MyBatis-Plus会自动提供CRUD操作。
java
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
public interface YourEntityMapper extends BaseMapper<YourEntity> {
// 无需编写任何方法
}
5. Service层
创建Service层,调用Mapper接口进行操作。
java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class YourEntityService {
@Autowired
private YourEntityMapper yourEntityMapper;
public void addYourEntity(YourEntity entity) {
yourEntityMapper.insert(entity);
}
// 其他业务方法
}
6. 控制器层
在控制器层调用Service层的方法,处理HTTP请求。
java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class YourEntityController {
@Autowired
private YourEntityService yourEntityService;
@PostMapping("/yourEntity")
public String addYourEntity(@RequestBody YourEntity entity) {
yourEntityService.addYourEntity(entity);
return "添加成功";
}
// 其他请求处理方法
}
四、总结
MyBatis-Plus通过提供强大的CRUD操作、条件构造器等功能,极大地简化了MyBatis的开发过程。通过上述步骤,你可以快速地将MyBatis-Plus集成到你的Spring Boot项目中,并开始享受它带来的便利。随着你对MyBatis-Plus的深入了解,你会发现更多强大的功能和最佳实践,从而进一步提高你的开发效率。