题目:
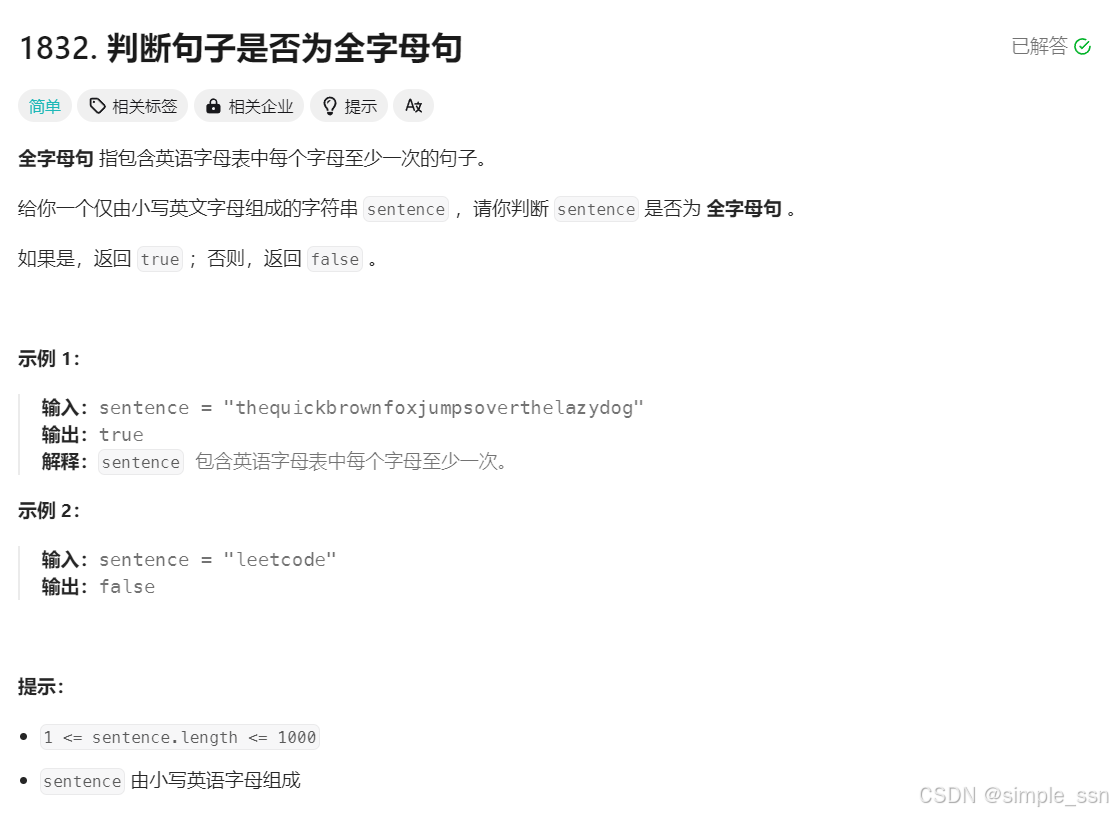
法一
bool checkIfPangram(char* sentence) {
int str[256];
memset(str, 0, sizeof(int));
for (int i = 0; i < strlen(sentence); ++i) {
++str[ sentence[i] ];
}
for (int j = 'a'; j <= 'z'; ++j) {
if (!str[j]) return false;
}
return true;
}
法二 动态分配
typedef struct {
char word;
int count;
UT_hash_handle hh;
} hashEntry;
bool checkIfPangram(char* sentence) {
hashEntry * cnt = NULL;
for (int i = 0; i < strlen(sentence); i++) {
hashEntry* pEntry = NULL;
HASH_FIND(hh, cnt, &sentence[i], sizeof(char), pEntry);
if (pEntry == NULL) {
hashEntry * pEntry = (hashEntry*)malloc(sizeof(hashEntry));
pEntry -> word = sentence[i];
pEntry -> count = 1;
HASH_ADD(hh, cnt, word, sizeof(char), pEntry);
}
else pEntry -> count++;
}
hashEntry *curr = NULL, *next = NULL;
int num = 26;
HASH_ITER(hh, cnt, curr, next)
{
if (curr -> count > 0) {
num--;
}
if (num == 0) return true;
free(curr);
}
return false;
}