记录自己所学,无详细讲解
带头循环双链表实现
1.项目目录文件
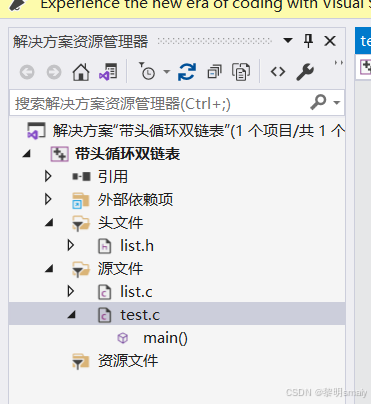
2.头文件 List.h
#include <stdlib.h>
#include <assert.h>
#include <stdio.h>
typedef struct List
{
int data;
struct List* prev;
struct List* next;
}List;
void ListInit(List** phead);//初始化
List* Buynewnode(List* phead,int n);//创建新节点
void ListPushfront(List* phead, int n);//头插
void ListPushback(List* phead, int n);//尾插
void ListPopfront(List* phead);//头删
void ListPopback(List* phead);//尾删
List* ListIsFind(List* phead, int n);//查找节点并返回地址
void ListInsertfront(List* phead, List* pos, int n);//节点前插入
void ListInsertback(List* phead, List* pos, int n);//节点后插入
void ListDel(List* phead, List* pos);//删除节点
void ListModify(List* pos, int n);//修改节点
void ListDestory(List* phead);//销毁节点
void ListPrint(List* phead);//打印输出
3.函数定义源文件 list.c
#include "list.h"
void ListInit(List** phead)
{
*phead = (List*)malloc(sizeof(List));
(*phead)->next = *phead;
(*phead)->prev = *phead;
(*phead)->data = 0;
}
List* Buynewnode(int n)
{
List* newnode = (List*)malloc(sizeof(List));
assert(newnode);
newnode->next = NULL;
newnode->prev = NULL;
newnode->data = n;
return newnode;
}
void ListPushfront(List* phead, int n)
{
List* newnode = Buynewnode(n);
(phead->next)->prev = newnode;
newnode->next = phead->next;
newnode->prev = phead;
phead->next = newnode;
}
void ListPushback(List* phead, int n)
{
List* newnode = Buynewnode(n);
List* cur = phead;
while (cur->next != phead)
{
cur = cur->next;
}
cur->next = newnode;
newnode->prev = cur;
newnode->next = phead;
phead->prev = newnode;
}
void ListPopfront(List* phead)
{
if (phead->next == phead)
{
printf("没东西无需删除\n");
}
else
{
List* node = phead->next;
(node->next)->prev = phead;
phead->next = node->next;
free(node);
node = NULL;
}
}
void ListPopback(List* phead)
{
if (phead->next == phead)
{
printf("无需删除\n");
}
else
{
List* node = phead->prev;
(node->prev)->next = phead;
phead->prev = node->prev;
free(node);
node = NULL;
}
}
List* ListIsFind(List* phead, int n)
{
List* cur = phead->next;
while (cur != phead)
{
if (cur->data == n)
{
return cur;
}
cur = cur->next;
}
return NULL;
}
void ListInsertfront(List* phead, List* pos, int n)
{
assert(pos);
List* newnode = Buynewnode(n);
newnode->next = pos;
(pos->prev)->next = newnode;
newnode->prev = pos->prev;
pos->prev = newnode;
}
void ListInsertback(List* phead, List* pos, int n)
{
assert(pos);
List* newnode = Buynewnode(n);
newnode->next = (pos->next);
(pos->next)->prev = newnode;
pos->next = newnode;
newnode->prev = pos;
}
void ListDel(List* phead,List* pos)
{
assert(pos);
List* node = pos;
(node->prev)->next = node->next;
(node->next)->prev = (node->prev)->next;
}
void ListModify(List* pos, int n)
{
assert(pos);
pos->data = n;
}
void ListDestory(List* phead)
{
List* cur = phead->next;
List* cur1 = cur;
while (cur != phead)
{
cur1 = cur;
cur = cur->next;
free(cur1);
cur1 = NULL;
}
phead->next = phead;
phead->prev = phead;
}
void ListPrint(List* phead)
{
List* cur = phead->next;
printf("NULL->");
while (cur != phead)
{
printf("%d->", cur->data);
cur = cur->next;
}
}
4.函数调用测试源文件test.c
#include "list.h"
int main()
{
List * phead = NULL;
ListInit(&phead);
ListPushback(phead, 5);
ListPushback(phead, 4);
ListPushback(phead, 3);
ListPushback(phead, 2);
ListPushback(phead, 1);
ListPushfront(phead, 6);
//ListPopfront(phead);
//ListPopfront(phead);
//ListPopfront(phead);
//ListPopfront(phead);
//ListPopback(phead);
//ListDestory(phead);
//ListInsertback(phead, ListIsFind(phead, 5), 9);
//ListModify(ListIsFind(phead, 9),10);
//ListDel(phead,ListIsFind(phead, 10));
ListPrint(phead);
}