最长最短单词
-
-
- C语言实现
- C++实现
- Java实现
- Python实现
-
|-----------------------------|
| 💐The Begin💐点点关注,收藏不迷路💐 |
输入1行句子(不多于200个单词,每个单词长度不超过100),只包含字母、空格和逗号。单词由至少一个连续的字母构成,空格和逗号都是单词间的间隔。
试输出第1个最长的单词和第1个最短单词。
输入
一行句子。
输出
第1行,第一个最长的单词。
第2行,第一个最短的单词。
样例输入
c
I am studying Programming language C in Peking University
样例输出
c
Programming
I
C语言实现
c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define MAX_LENGTH 200 * 100 // 考虑句子最多200个单词,每个单词最长100字符,预留足够空间
int main() {
char sentence[MAX_LENGTH]; // 存储输入的句子
fgets(sentence, MAX_LENGTH, stdin); // 从标准输入读取句子,包含换行符
int len = strlen(sentence);
if (len > 0 && sentence[len - 1] == '\n') { // 去除换行符
sentence[len - 1] = '\0';
}
char longest_word[100]; // 存储最长的单词
char shortest_word[100]; // 存储最短的单词
strcpy(longest_word, ""); // 初始化为空字符串
strcpy(shortest_word, ""); // 初始化为空字符串
int current_word_start = 0; // 当前单词开始的位置
int current_word_length = 0; // 当前单词的长度
int max_length = 0; // 记录最长单词长度
int min_length = 100; // 初始设为一个较大值,方便比较找最短单词
for (int i = 0; i < len; i++) {
if (isalpha(sentence[i])) { // 如果是字母,说明在单词内
current_word_length++;
} else if (sentence[i] =='' || sentence[i] == ',') { // 如果是间隔符号,说明单词结束
if (current_word_length > max_length) { // 判断是否是目前最长的单词
max_length = current_word_length;
strncpy(longest_word, sentence + current_word_start, current_word_length);
longest_word[current_word_length] = '\0';
}
if (current_word_length < min_length && current_word_length > 0) { // 判断是否是目前最短的单词且长度大于0
min_length = current_word_length;
strncpy(shortest_word, sentence + current_word_start, current_word_length);
shortest_word[current_word_length] = '\0';
}
current_word_start = i + 1; // 更新下一个单词开始的位置
current_word_length = 0; // 重置当前单词长度
}
}
// 处理最后一个单词的情况(因为循环结束时没处理最后一个单词结束的情况)
if (current_word_length > max_length) {
max_length = current_word_length;
strncpy(longest_word, sentence + current_word_start, current_word_length);
longest_word[current_word_length] = '\0';
}
if (current_word_length < min_length && current_word_length > 0) {
min_length = current_word_length;
strncpy(shortest_word, sentence + current_word_start, current_word_length);
shortest_word[current_word_length] = '\0';
}
printf("%s\n", longest_word);
printf("%s\n", shortest_word);
return 0;
}
C++实现
cpp
#include <iostream>
#include <string>
#include <sstream>
#include <algorithm>
using namespace std;
int main() {
string sentence;
getline(cin, sentence); // 从标准输入读取句子,自动去除换行符
string longest_word; // 存储最长的单词
string shortest_word; // 存储最短的单词
longest_word = ""; // 初始化为空字符串
shortest_word = ""; // 初始化为空字符串
stringstream ss(sentence); // 创建stringstream对象,用于分割句子
string current_word;
int max_length = 0; // 记录最长单词长度
int min_length = 100; // 初始设为一个较大值,方便比较找最短单词
while (ss >> current_word) { // 从stringstream中按空格或逗号分割读取单词
int length = current_word.length();
if (length > max_length) { // 判断是否是目前最长的单词
max_length = length;
longest_word = current_word;
}
if (length < min_length && length > 0) { // 判断是否是目前最短的单词且长度大于0
min_length = length;
shortest_word = current_word;
}
}
cout << longest_word << endl;
cout << shortest_word << endl;
return 0;
}
Java实现
java
import java.util.Scanner;
public class LongestAndShortestWord {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String sentence = scanner.nextLine(); // 从标准输入读取句子
String longestWord = ""; // 存储最长的单词
String shortestWord = ""; // 存储最短的单词
int maxLength = 0; // 记录最长单词长度
int minLength = 100; // 初始设为一个较大值,方便比较找最短单词
String[] words = sentence.split("[,\\s]+"); // 按空格或逗号分割句子得到单词数组
for (String word : words) {
int length = word.length();
if (length > maxLength) { // 判断是否是目前最长的单词
maxLength = length;
longestWord = word;
}
if (length < minLength && length > 0) { // 判断是否是目前最短的单词且长度大于0
minLength = length;
shortestWord = word;
}
}
System.out.println(longestWord);
System.out.println(shortestWord);
scanner.close();
}
}
Python实现
python
sentence = input() # 从标准输入读取句子
words = sentence.split() # 以空格为分隔符将句子分割成单词列表
longest_word = "" # 存储最长的单词
shortest_word = "" # 存储最短的单词
for word in words:
word = word.strip(",") # 去除单词两端可能存在的逗号
length = len(word)
if length > len(longest_word): # 判断是否是目前最长的单词
longest_word = word
if length < len(shortest_word) or shortest_word == "": # 判断是否是目前最短的单词
shortest_word = word
print(longest_word)
print(shortest_word)
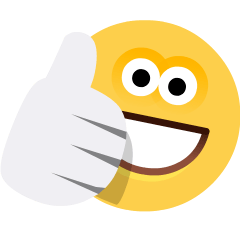
|---------------------------|
| 💐The End💐点点关注,收藏不迷路💐 |