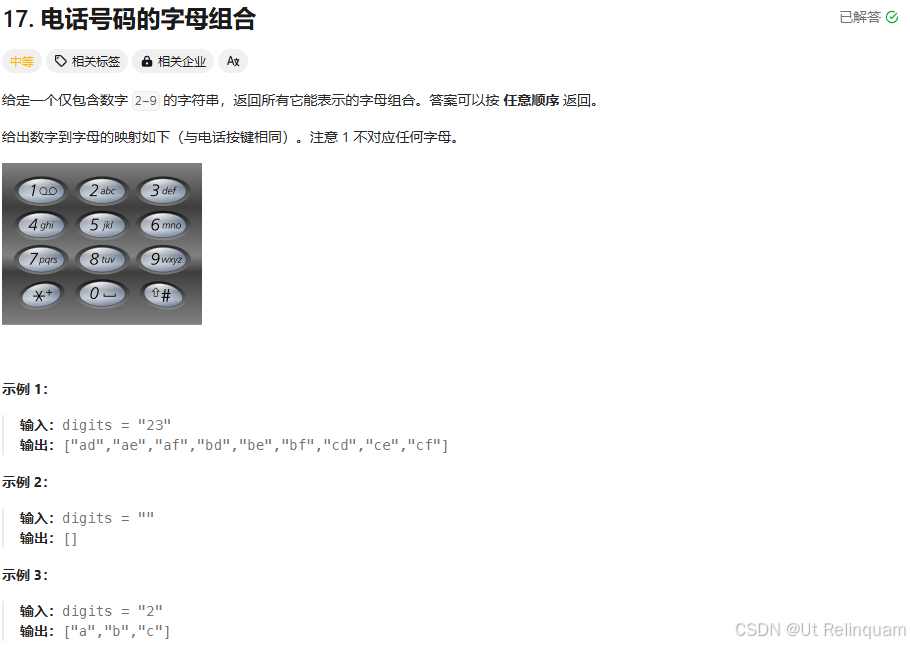
java
class Solution {
HashMap<Character,String> hm=new HashMap<>();
List<String> res=new ArrayList<>();
StringBuilder path=new StringBuilder();
public List<String> letterCombinations(String digits) {
if(digits==null||digits.length()==0){
return res;
}
hm.put('1',"");
hm.put('2',"abc");
hm.put('3',"def");
hm.put('4',"ghi");
hm.put('5',"jkl");
hm.put('6',"mno");
hm.put('7',"pqrs");
hm.put('8',"tuv");
hm.put('9',"wxyz");
hm.put('0',"");
slout(digits,digits.length(),0);
return res;
}
void slout(String digits,int le,int startIndex){
if(path.length()==le){
res.add(path.toString());
return;
}
String st=hm.get(digits.charAt(startIndex));
for(int i=0;i<st.length();i++){
path.append(st.charAt(i));
slout(digits,le,startIndex+1);
path.deleteCharAt(path.length()-1);
}
}
}
python
class Solution(object):
def letterCombinations(self, digits):
hm={
'1':"",
'2':"abc",
'3':"def",
'4':"ghi",
'5':"jkl",
'6':"mno",
'7':"pqrs",
'8':"tuv",
'9':"wxyz",
'0':"",
}
res=[]
path=[]
def slout(digits,le,startIndex):
if len(path)==le:
res.append("".join(path))
return
st=hm[digits[startIndex]]
for i in range(len(st)):
path.append(st[i])
slout(digits,le,startIndex+1)
path.pop()
if digits is None or len(digits)==0:
return res
slout(digits,len(digits),0)
return res