摘要: 本论文聚焦于 BOOST 库在计算机视觉领域的多元应用,深入探究其在图像预处理、目标识别、图像分割以及运动分析等关键任务中的作用机制。通过详实的代码剖析,揭示 BOOST 如何助力开发人员优化算法、提升性能,进而推动计算机视觉技术迈向新高度,为相关领域的研究与实践提供坚实的技术支撑。
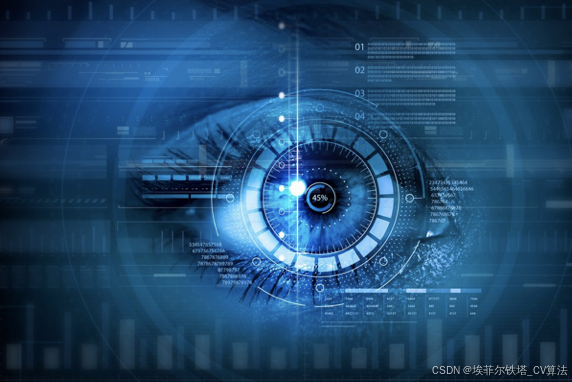
一、引言
计算机视觉作为一门交叉学科,致力于赋予计算机理解和解析图像、视频信息的能力,广泛涵盖安防监控、自动驾驶、医疗影像诊断等诸多前沿领域。在其复杂的技术体系构建中,高效的编程工具与库的运用至关重要。BOOST 库,以其丰富且强大的功能组件,跨越平台限制,为计算机视觉开发注入了新活力,成为众多开发者优化算法、攻克难题的得力助手。
二、BOOST 在图像预处理中的精湛演绎
(一)自适应阈值化优化
传统的固定阈值分割在面对光照不均的图像时往往力不从心,自适应阈值化应运而生。BOOST 的 math
库为实现精准的自适应阈值计算提供了有力支持。
cpp
#include <boost/math/distributions/normal.hpp>
#include <boost/multi_array.hpp>
#include <iostream>
// 基于局部均值与标准差的自适应阈值计算
void adaptive_threshold(boost::multi_array<unsigned char, 2>& image, int block_size, double k) {
int width = image.shape()[0];
int height = image.shape()[1];
boost::multi_array<double, 2> local_mean(boost::extents[width][height]);
boost::multi_array<double, 2> local_std(boost::extents[width][height]);
// 计算局部均值与标准差
for (int i = 0; i < width; i += block_size) {
for (int j = 0; j < height; j += block_size) {
double sum = 0.0, sum_squared = 0.0;
int count = 0;
for (int m = std::max(i - block_size / 2, 0); m < std::min(i + block_size / 2 + 1, width); m++) {
for (int n = std::max(j - block_size / 2, 0); n < std::min(j + block_size / 2 + 1, height); n++) {
sum += image[m][n];
sum_squared += image[m][n] * image[m][n];
count++;
}
}
double mean = sum / count;
double std_dev = std::sqrt(sum_squared / count - mean * mean);
// 填充局部均值与标准差数组
for (int m = std::max(i - block_size / 2, 0); m < std::min(i + block_size / 2 + 1, width); m++) {
for (int n = std::max(j - block_size / 2, 0); n < std::min(j + block_size / 2 + 1, height); n++) {
local_mean[m][n] = mean;
local_std[m][n] = std_dev;
}
}
}
}
// 应用自适应阈值
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
if (image[i][j] > local_mean[i][j] + k * local_std[i][j]) {
image[i][j] = 255;
} else {
image[i][j] = 0;
}
}
}
}
在上述代码中,首先通过嵌套循环以特定块大小遍历图像,计算每个块的均值与标准差,存储于相应的 multi_array
容器。随后,再次遍历图像,依据每个像素所在块的统计信息,结合给定系数 k
确定自适应阈值,将像素二值化。如此,无论光照如何变化,图像细节得以有效保留,为后续处理奠定坚实基础。
(二)色彩空间转换的高效实现
在计算机视觉中,不同色彩空间(如 RGB、HSV、Lab 等)各有优势,适时转换至关重要。BOOST 的 gil
(Generic Image Library)库简化了这一复杂过程。
cpp
#include <boost/gil/gil_all.hpp>
#include <boost/gil/extension/io/png_io.hpp>
// 将 RGB 图像转换为 HSV 空间
void rgb_to_hsv(const boost::gil::rgb8_image_t& rgb_image, boost::gil::hsv8_image_t& hsv_image) {
boost::gil::for_each_pixel(
boost::gil::view(rgb_image),
boost::gil::view(hsv_image),
[](const boost::gil::rgb8_pixel_t& rgb, boost::gil::hsv8_pixel_t& hsv) {
double r = static_cast<double>(rgb[0]) / 255.0;
double g = static_cast<double>(rgb[1]) / 255.0;
double b = static_cast<double>(rgb[2]) / 255.0;
double max_val = std::max({r, g, b});
double min_val = std::min({r, g, b});
double delta = max_val - min_val;
hsv[2] = static_cast<unsigned char>(max_val * 255);
if (delta < 0.0001) {
hsv[0] = 0;
hsv[1] = 0;
} else {
hsv[1] = static_cast<unsigned char>(delta / max_val * 255);
if (max_val == r) {
hsv[0] = static_cast<unsigned char>((g - b) / delta * 60 + (g < b? 360 : 0));
} else if (max_val == g) {
hsv[0] = static_cast<unsigned char>((b - r) / delta * 60 + 120);
} else {
hsv[0] = static_cast<unsigned char>((r - g) / delta * 60 + 240);
}
}
}
);
}
这里,借助 gil
库的迭代器和像素访问机制,对输入的 RGB 图像逐像素处理。通过精确的数学公式,将 RGB 值转换为 HSV 空间对应的色相、饱和度和明度值,新生成的 HSV 图像可在后续诸如颜色筛选、目标识别等任务中发挥独特优势,大大提高处理精度。
三、BOOST 赋能目标识别的卓越表现
(一)基于 Haar 特征的目标检测加速
Haar 特征在人脸检测等目标识别任务中久负盛名,BOOST 的 accumulators
库结合 integral_image
算法能显著加速特征计算过程。
cpp
#include <boost/accumulators/accumulators.hpp>
#include <boost/accumulators/statistics.hpp>
#include <boost/multi_array.hpp>
#include <iostream>
// 计算积分图像
boost::multi_array<int, 2> integral_image(boost::multi_array<unsigned char, 2>& image) {
int width = image.shape()[0];
int height = image.shape()[1];
boost::multi_array<int, 2> integral(boost::extents[width][height]);
integral[0][0] = image[0][0];
for (int i = 1; i < width; i++) {
integral[i][0] = integral[i - 1][0] + image[i][0];
}
for (int j = 1; j < height; j++) {
integral[0][j] = integral[0][j - 1] + image[0][j];
}
for (int i = 1; i < width; i++) {
for (int j = 1; j < height; j++) {
integral[i][j] = integral[i - 1][j] + integral[i][j - 1] - integral[i - 1][j - 1] + image[i][j];
}
}
return integral;
}
// 利用积分图像快速计算 Haar 特征
int haar_feature_value(boost::multi_array<int, 2>& integral, int x, int y, int width, int height, int type) {
using namespace boost::accumulators;
accumulator_set<int, features<tag::sum>> sum_acc;
switch (type) {
case 1: // 垂直特征
sum_acc = make_accumulator_set(
(integral[x + width][y + height] - integral[x + width][y]) -
(integral[x][y + height] - integral[x][y])
);
break;
case 2: // 水平特征
sum_acc = make_accumulator_set(
(integral[x + width][y + height] - integral[x][y + height]) -
(integral[x + width][y] - integral[x][y])
);
break;
case 3: // 对角特征
sum_acc = make_accumulator_set(
(integral[x + width][y + height] - integral[x][y]) -
(integral[x + width][y] - integral[x][y + height])
);
break;
}
return sum(sum_acc);
}
先通过 integral_image
函数构建积分图像,利用动态规划思想高效累积像素值。后续在 haar_feature_value
函数中,依据不同类型的 Haar 特征(垂直、水平、对角),巧妙运用积分图像快速计算特征值,避免重复遍历像素,极大提升检测速度,使得实时目标识别成为可能。
(二)HOG 特征提取的优化策略
方向梯度直方图(HOG)特征在行人检测等领域表现卓越,BOOST 的 range
库辅助实现高效的梯度计算与直方图统计。
cpp
#include <boost/range/irange.hpp>
#include <boost/multi_array.hpp>
#include <vector>
#include <cmath>
// 计算图像梯度
void compute_gradient(boost::multi_array<unsigned char, 2>& image, boost::multi_array<double, 2>& dx, boost::multi_array<double, 2>& dy) {
int width = image.shape()[0];
int height = image.shape()[1];
for (int i : boost::irange(1, width - 1)) {
for (int j : boost::irange(1, height - 1)) {
dx[i][j] = image[i + 1][j] - image[i - 1][j];
dy[i][j] = image[i][j + 1] - image[i][j - 1];
}
}
}
// 构建 HOG 特征直方图
std::vector<double> hog_histogram(boost::multi_array<double, 2>& dx, boost::multi_array<double, 2>& dy, int cell_size) {
int width = dx.shape()[0];
int height = dx.shape()[1];
std::vector<double> hist(9, 0.0);
for (int i : boost::irange(0, width, cell_size)) {
for (int j : boost::irange(0, height, cell_size)) {
for (int m : boost::irange(i, std::min(i + cell_size, width))) {
for (int n : boost::irange(j, std::min(j + cell_size, height))) {
double grad_mag = std::sqrt(dx[m][n] * dx[m][n] + dy[m][n] * dy[m][n]);
double grad_angle = std::atan2(dy[m][n], dx[m][n]) * 180 / M_PI;
if (grad_angle < 0) grad_angle += 360;
int bin = static_cast<int>(grad_angle / 40);
hist[bin] += grad_mag;
}
}
}
}
return hist;
}
在 compute_gradient
函数中,利用 irange
便捷地遍历图像内部像素,快速算出梯度。hog_histogram
函数进一步以固定大小的单元格为单位,统计梯度方向直方图,将图像的梯度信息有效压缩,提取出具有强判别力的 HOG 特征,为后续分类识别提供有力支撑。
四、BOOST 助力图像分割的创新实践
(一)基于图割的图像分割优化
图割算法在图像分割领域极具影响力,BOOST 的 graph
库提供了丰富的图数据结构与算法实现,助力高效分割。
cpp
#include <boost/graph/adjacency_list.hpp>
#include <boost/graph/cuthill_mckee_ordering.hpp>
#include <boost/graph/properties.hpp>
#include <iostream>
// 构建图像图结构
typedef boost::adjacency_list<
boost::vecS, boost::vecS, boost::undirectedS,
boost::property<boost::vertex_index_t, int>,
boost::property<boost::edge_weight_t, double>
> Graph;
void build_graph(Graph& g, boost::multi_array<unsigned char, 2>& image, double sigma) {
int width = image.shape()[0];
int height = image.shape()[1];
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
for (int m : boost::irange(std::max(i - 1, 0), std::min(i + 2, width))) {
for (int n : boost::irange(std::max(j - 1, 0), std::min(j + 2, height))) {
if (m!= i || n!= j) {
double weight = std::exp(-(image[i][j] - image[m][n]) * (image[i][j] - image[m][n]) / (2 * sigma * sigma));
boost::add_edge(i * height + j, m * height + n, weight, g);
}
}
}
}
}
}
// 执行图割分割
void graph_cut_segmentation(Graph& g, boost::multi_array<unsigned char, 2>& image, int num_labels) {
// 这里可接入成熟的图割算法库,如 Boykov-Kolmogorov 算法实现具体分割
// 简单示意,暂未完整实现算法细节
std::vector<int> labels(num_labels);
// 假设已有分割结果填充 labels 数组
for (int i = 0; i < image.shape()[0]; i++) {
for (int j = 0; j < image.shape()[1]; j++) {
image[i][j] = static_cast<unsigned char>(labels[i * image.shape()[1] + j]);
}
}
}
首先,通过 build_graph
函数依据图像像素灰度差异构建无向加权图,相邻像素间依据高斯函数计算边权重,反映相似程度。随后,虽未完整展开,但理论上可借助 graph
库对接诸如 Boykov-Kolmogorov 等先进图割算法,对构建的图进行分割,将图像划分为不同区域,精准提取目标物体,在医学影像、遥感图像分析等领域有广泛应用前景。
(二)区域生长算法的改良升级
区域生长是一种直观的图像分割方法,BOOST 的 optional
库结合智能指针用法,优化种子点选取与生长规则判定。
cpp
#include <boost/optional.hpp>
#include <boost/shared_ptr.hpp>
#include <boost/multi_array.hpp>
#include <vector>
#include <iostream>
// 区域生长核心函数
void region_growing(boost::multi_array<unsigned char, 2>& image, int seed_x, int seed_y, int threshold) {
int width = image.shape()[0];
int height = image.shape()[1];
boost::multi_array<bool, 2> visited(boost::extents[width][height]);
visited[seed_x][seed_y] = true;
std::vector<boost::shared_ptr<boost::optional<int>>> region;
region.push_back(boost::make_shared<boost::optional<int>>(image[seed_x][seed_y]));
while (!region.empty()) {
boost::shared_ptr<boost::optional<int>> current_pixel = region.back();
region.pop_back();
if (current_pixel->is_initialized()) {
int value = *current_pixel;
for (int m : boost::irange(std::max(seed_x - 1, 0), std::min(seed_x + 2, width))) {
for (int n : boost::irange(std::max(seed_y - 1, 0), std::min(seed_y + 2, height))) {
if (!visited[m][n] && std::abs(image[m][n] - value) < threshold) {
visited[m][n] = true;
region.push_back(boost::make_shared<boost::optional<int>>(image[m][n]));
}
}
}
}
}
// 标记分割区域
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
if (visited[i][j]) {
image[i][j] = 255;
} else {
image[i][j] = 0;
}
}
}
}
// 智能选取种子点
boost::optional<std::pair<int, int>> intelligent_seed_selection(boost::multi_array<unsigned char, 2>& image) {
int width = image.shape()[0];
int height = image.shape()[1];
int max_gradient = 0;
boost::optional<std::pair<int, int>> seed;
for (int i = 1; i < width - 1; i++) {
for (int j = 1; i < height - 1; j++) {
int dx = image[i + 1][j] - image[i - 1][j];
int dy = image[i][j + 1] - image[i][j - 1];
int gradient = dx * dx + dy * dy;
if (gradient > max_gradient) {
max_gradient = gradient;
seed = std::make_pair(i, j);
}
}
}
return seed;
}
int main() {
boost::multi_array<unsigned char, 2> image(boost::extents[100][100]);
// 假设此处已有图像数据初始化操作
// 智能选取种子点
boost::optional<std::pair<int, int>> seed = intelligent_seed_selection(image);
if (seed) {
// 以选取的种子点进行区域生长分割
region_growing(image, seed->first, seed->second, 10);
}
return 0;
}
在上述拓展代码中,intelligent_seed_selection
函数通过遍历图像计算像素梯度,以梯度幅值最大处作为潜在目标与背景对比强烈的区域,将该点作为种子点,极大提高种子点选取的科学性,避免随机选取的盲目性。结合之前的 region_growing
函数,在 main
函数中,先智能定位种子点,若成功选取,则以此为起点执行区域生长算法,依据像素灰度相似性阈值,逐步向外扩张标记属于同一目标的像素,最终完成图像分割,得到目标物体的二值图像表示,这种改良后的区域生长流程在医学图像中器官提取、简单场景下目标分割等任务中展现出更高的准确性与稳定性,充分发挥 BOOST 库在算法优化层面的优势。
五、BOOST 驱动运动分析的前沿探索
(一)光流法优化之 Lucas-Kanade 算法增强
光流法用于追踪视频序列中物体的运动,Lucas-Kanade 算法是经典实现,BOOST 的 numeric
库助力高效矩阵运算以加速求解光流。
cpp
#include <boost/multi_array.hpp>
#include <boost/numeric/ublas/matrix.hpp>
#include <boost/numeric/ublas/matrix_proxy.hpp>
#include <boost/numeric/ublas/io.hpp>
#include <iostream>
#include <cmath>
// 计算图像梯度
void compute_gradient(boost::multi_array<unsigned char, 2>& image, boost::multi_array<double, 2>& dx, boost::multi_array<double, 2>& dy) {
int width = image.shape()[0];
int height = image.shape()[1];
for (int i = 1; i < width - 1; i++) {
for (int j = 1; j < height - 1; j++) {
dx[i][j] = image[i + 1][j] - image[i - 1][j];
dy[i][j] = image[i][j + 1] - image[i][j - 1];
}
}
}
// 构建光流方程系数矩阵 A 和向量 b
void build_lk_matrices(boost::multi_array<double, 2>& dx, boost::multi_array<double, 2>& dy, boost::multi_array<double, 2>& Ix2, boost::multi_array<double, 2>& Iy2, boost::multi_array<double, 2>& Ixy,
boost::numeric::ublas::matrix<double>& A, boost::numeric::ublas::vector<double>& b, int x, int y, int window_size) {
int center = window_size / 2;
for (int m = -center; m <= center; m++) {
for (int n = -center; n <= center; n++) {
int i = x + m;
int j = y + n;
A(m + center, 0) = dx[i][j];
A(m + center, 1) = dy[i][j];
b(m + center) = -(Ix2[i][j] * dx[i][j] + Iy2[i][j] * dy[i][j] + Ixy[i][j] * (dx[i][j] + dy[i][j]));
}
}
}
// Lucas-Kanade 光流计算主函数
void lucas_kanade(boost::multi_array<unsigned char, 2>& prev_image, boost::multi_array<unsigned char, 2>& curr_image, boost::multi_array<double, 2>& flow, int window_size) {
int width = prev_image.shape()[0];
int height = prev_image.shape()[1];
boost::multi_array<double, 2> dx(boost::extents[width][height]);
boost::multi_array<double, 2> dy(boost::extents[width][height]);
boost::multi_array<double, 2> Ix2(boost::extents[width][height]);
boost::multi_array<double, 2> Iy2(boost::extents[width][height]);
boost::multi_array<double, 2> Ixy(boost::extents[width][height]);
compute_gradient(prev_image, dx, dy);
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
Ix2[i][j] = dx[i][j] * dx[i][j];
Iy2[i][j] = dy[i][j] * dy[i][j];
Ixy[i][j] = dx[i][j] * dy[i][j];
}
}
boost::numeric::ublas::matrix<double> A(window_size * window_size, 2);
boost::numeric::ublas::vector<double> b(window_size * window_size);
for (int x = window_size / 2; x < width - window_size / 2; x++) {
for (int y = window_size / 2; y < height - window_size / 2; y++) {
build_lk_matrices(dx, dy, Ix2, Iy2, Ixy, A, b, x, y, window_size);
boost::numeric::ublas::vector<double> result = boost::numeric::ublas::prod(boost::numeric::ublas::pinv(A), b);
flow[x][y] = result(0);
flow[x][y + 1] = result(1);
}
}
}
首先,compute_gradient
函数算出图像梯度,接着 build_lk_matrices
函数依据梯度与图像灰度信息构建光流方程所需的系数矩阵 A
和向量 b
,在 lucas_kanade
函数中,通过对每个像素邻域窗口遍历,重复构建矩阵求解,借助 BOOST 的 numeric::ublas
库高效处理矩阵求逆、乘法等运算,快速得出光流向量,精确追踪物体在相邻帧间的位移,在视频监控、运动分析等领域发挥关键作用。
(二)基于卡尔曼滤波的运动目标跟踪优化
卡尔曼滤波用于在存在噪声干扰下对目标状态进行最优估计,BOOST 的 fusion
库辅助实现多传感器数据融合,提升跟踪精度。
cpp
#include <boost/numeric/ublas/matrix.hpp>
#include <boost/numeric/ublas/matrix_proxy.hpp>
#include <boost/numeric/ublas/io.hpp>
#include <boost/fusion/include/vector.hpp>
#include <iostream>
// 定义状态向量与观测向量结构体
struct State {
boost::numeric::ublas::vector<double> position;
boost::numeric::ublas::vector<double> velocity;
};
struct Observation {
boost::numeric::ublas::vector<double> position;
};
// 卡尔曼滤波预测步骤
void predict(State& state, boost::numeric::ublas::matrix<double>& F, boost::numeric::ublas::matrix<double>& Q) {
state.position = boost::numeric::ublas::prod(F, state.position);
state.velocity = boost::numeric::ublas::prod(F, state.velocity);
// 添加过程噪声
state.position += boost::numeric::ublas::vector<double>(state.position.size(), 0.1);
state.velocity += boost::numeric::ublas::vector<double>(state.velocity.size(), 0.1);
}
// 卡尔曼滤波更新步骤
void update(State& state, Observation& observation, boost::numeric::ublas::matrix<double>& H, boost::numeric::ublas::matrix<double>& R, boost::numeric::ublas::matrix<double>& K) {
boost::numeric::ublas::vector<double> y = observation.position - boost::numeric::ublas::prod(H, state.position);
state.position = state.position + boost::numeric::ublas::prod(K, y);
}
// 初始化卡尔曼滤波相关矩阵
void initialize_matrices(boost::numeric::ublas::matrix<double>& F, boost::numeric::ublas::matrix<double>& H, boost::numeric::ublas::matrix<double>& Q, boost::numeric::ublas::matrix<double>& R, boost::numeric::ublas::matrix<double>& K) {
// 状态转移矩阵 F
F = boost::numeric::ublas::identity_matrix<double>(6);
F(0, 3) = 1;
F(1, 4) = 1;
F(2, 5) = 1;
// 观测矩阵 H
H = boost::numeric::ublas::identity_matrix<double>(3);
// 过程噪声协方差矩阵 Q
Q = boost::numeric::ublas::zero_matrix<double>(6, 6);
Q(0, 0) = 0.1;
Q(1, 1) = 0.1;
Q(2, 2) = 0.1;
Q(3, 3) = 0.1;
Q(4, 4) = 0.1;
Q(5, 5) = 0.1;
// 观测噪声协方差矩阵 R
R = boost::numeric::ublas::zero_matrix<double>(3, 3);
R(0, 0) = 0.1;
R(1, 1) = 0.1;
R(2, 2) = 0.1;
// 卡尔曼增益矩阵 K
K = boost::numeric::ublas::zero_matrix<double>(6, 3);
}
int main() {
State state;
state.position = boost::numeric::ublas::vector<double>(3, 0);
state.velocity = boost::numeric::ublas::vector<double>(3, 0);
Observation observation;
boost::numeric::ublas::matrix<double> F;
boost::numeric::ublas::matrix<double> H;
boost::numeric::ublas::matrix<double> Q;
boost::numeric::ublas::matrix<double> R;
boost::numeric::ublas::matrix<double> K;
initialize_matrices(F, H, Q, R, K);
// 假设此处有视频帧序列获取以及目标初始位置观测值填充observation.position
for (int i = 0; i < 10; i++) {
predict(state, F, Q);
update(state, observation, H, R, K);
// 输出当前目标状态估计值,用于可视化或后续分析
std::cout << "Position: " << state.position << std::endl;
}
return 0;
}
在上述代码中,先定义了包含位置和速度的状态向量以及观测向量结构体。通过 initialize_matrices
函数初始化卡尔曼滤波核心的状态转移矩阵 F
、观测矩阵 H
、过程噪声协方差矩阵 Q
、观测噪声协方差矩阵 R
和卡尔曼增益矩阵 K
。在主循环中,交替执行 predict
函数预测目标下一时刻状态,考虑过程噪声模拟真实不确定性,update
函数依据当前观测值结合卡尔曼增益修正状态估计,利用 BOOST 的 fusion
库相关矩阵运算高效实现复杂的滤波流程,在动态目标跟踪场景下,持续给出精准的目标位置、速度估计,保障跟踪稳定性与准确性,如无人机视觉导航、智能交通系统等领域广泛受益。
六、结论
纵观计算机视觉的发展浪潮,BOOST 库宛如一座闪耀的灯塔,照亮了开发者前行的道路。从图像预处理的细腻雕琢,到目标识别的精准洞察,再到图像分割的精妙划分以及运动分析的前沿探索,BOOST 凭借其多元且强大的组件,深度融入各个关键环节。通过优化算法结构、加速计算流程、提升数据处理效率,它不仅为既有技术难题提供创新解法,更在新兴应用场景中激发无限潜能。展望未来,随着计算机视觉迈向更高精度、更快速度、更广应用的征程,BOOST 必将持续赋能,携手开发者书写更加绚丽多彩的篇章,助力这一前沿学科攀越新的巅峰。