目录
C# OpenCvSharp 部署3D人脸重建3DDFA-V3
说明
地址:https://github.com/wang-zidu/3DDFA-V3
3DDFA_V3 uses the geometric guidance of facial part segmentation for face reconstruction, improving the alignment of reconstructed facial features with the original image and excelling at capturing extreme expressions. The key idea is to transform the target and prediction into semantic point sets, optimizing the distribution of point sets to ensure that the reconstructed regions and the target share the same geometry.
效果
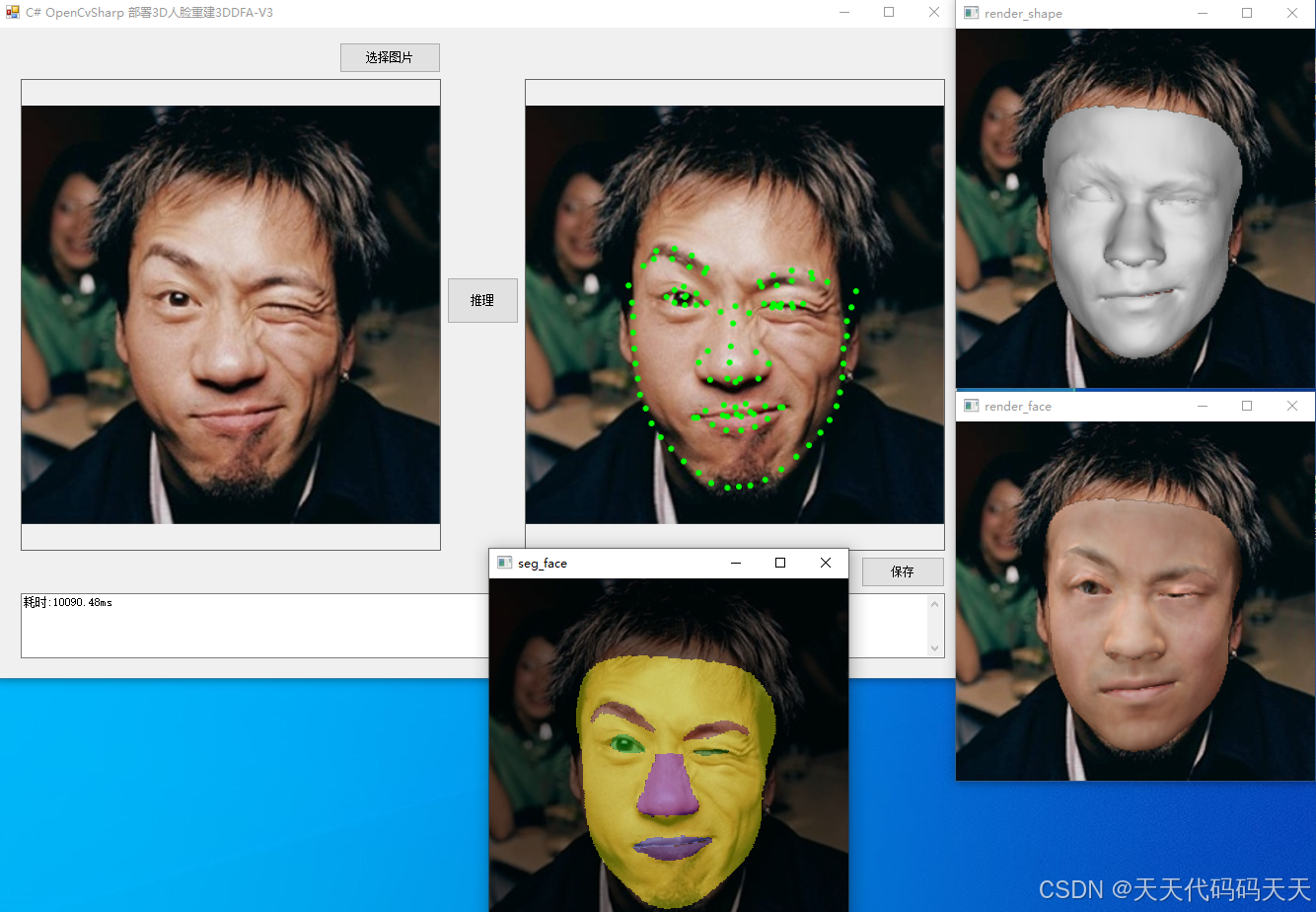
模型信息
landmark.onnx
Model Properties
Inputs
name:input
tensor:Float[1, 3, 224, 224]
Outputs
name:output
tensor:Float[1, 212]
net_recon.onnx
Model Properties
Inputs
name:input
tensor:Float[1, 3, 224, 224]
Outputs
name:output
tensor:Float[1, 257]
net_recon_mbnet.onnx
Model Properties
Inputs
name:input
tensor:Float[1, 3, 224, 224]
Outputs
name:output
tensor:Float[1, 257]
retinaface_resnet50.onnx
Model Properties
Inputs
name:input
tensor:Float[1, 3, 512, 512]
Outputs
name:loc
tensor:Float[1, 10752, 4]
name:conf
tensor:Float[1, 10752, 2]
name:land
tensor:Float[1, 10752, 10]
项目
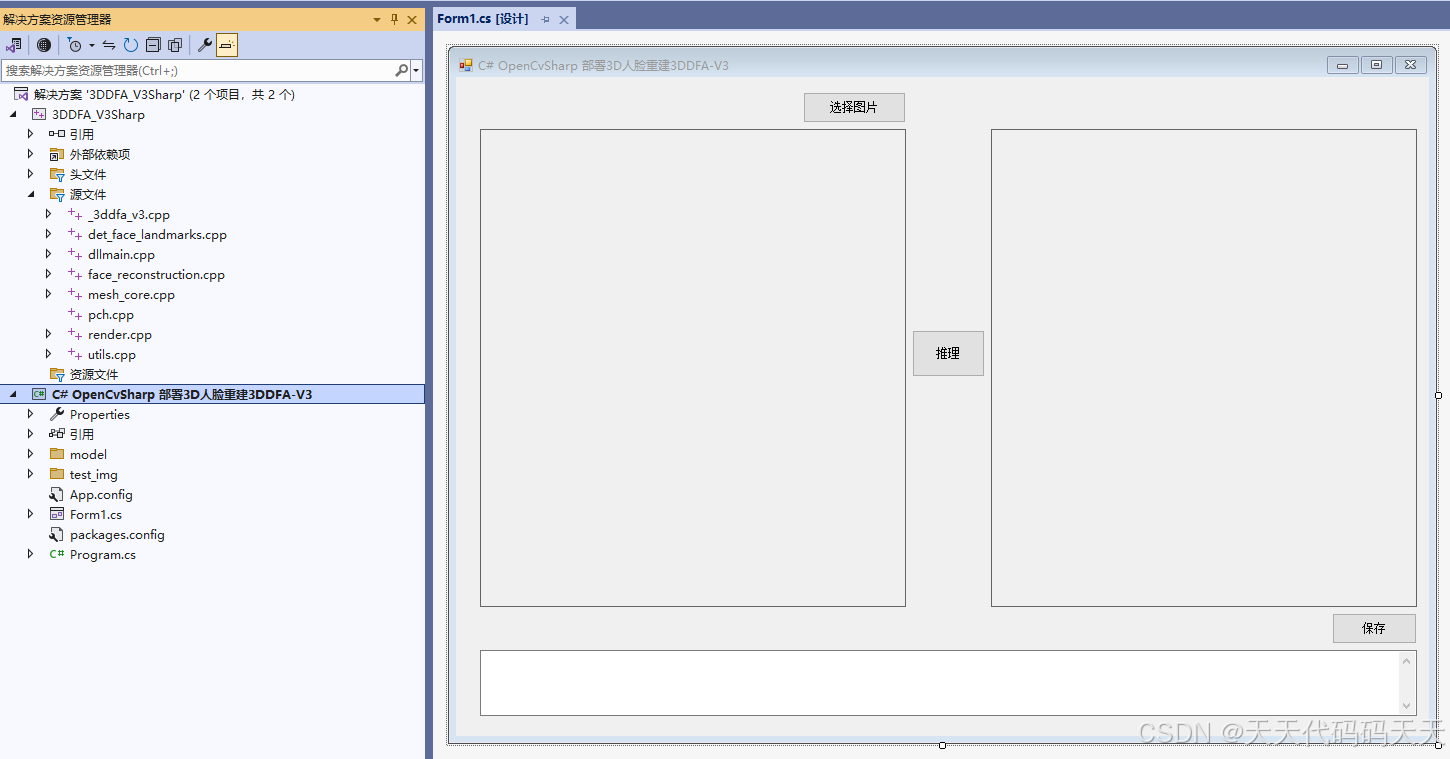
代码
using OpenCvSharp;
using System;
using System.Diagnostics;
using System.Drawing;
using System.Runtime.InteropServices;
using System.Text;
using System.Windows.Forms;
namespace C__OpenCvSharp_部署3D人脸重建3DDFA_V3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
Stopwatch stopwatch = new Stopwatch();
Mat image;
string image_path;
string startupPath;
string model_path;
string fileFilter = "*.*|*.bmp;*.jpg;*.jpeg;*.tiff;*.tiff;*.png";
const string DllName = "3DDFA_V3Sharp.dll";
IntPtr engine;
/*
//初始化
extern "C" _declspec(dllexport) int __cdecl init(void** engine, char* model_path, char* msg);
//forward
extern "C" _declspec(dllexport) int __cdecl forward(void* engine, Mat* srcimg, char* msg, Mat* render_shape, Mat* render_face, Mat* seg_face, Mat* landmarks);
//释放
extern "C" _declspec(dllexport) void __cdecl destroy(void* engine);
*/
[DllImport(DllName, EntryPoint = "init", CallingConvention = CallingConvention.Cdecl)]
internal extern static int init(ref IntPtr engine, string model_path, StringBuilder msg);
[DllImport(DllName, EntryPoint = "forward", CallingConvention = CallingConvention.Cdecl)]
internal extern static int forward(IntPtr engine, IntPtr image, StringBuilder msg, IntPtr render_shape, IntPtr render_face, IntPtr seg_face, IntPtr landmarks);
[DllImport(DllName, EntryPoint = "destroy", CallingConvention = CallingConvention.Cdecl)]
internal extern static int destroy(IntPtr engine);
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd = new OpenFileDialog();
ofd.Filter = fileFilter;
if (ofd.ShowDialog() != DialogResult.OK) return;
pictureBox1.Image = null;
pictureBox2.Image = null;
textBox1.Text = "";
image_path = ofd.FileName;
pictureBox1.Image = new Bitmap(image_path);
image = new Mat(image_path);
}
Mat render_shape;
Mat render_face;
Mat seg_face;
Mat landmarks;
private void button2_Click(object sender, EventArgs e)
{
if (image_path == "")
{
return;
}
button2.Enabled = false;
Application.DoEvents();
Cv2.DestroyAllWindows();
if (image != null) image.Dispose();
if (render_shape != null) render_shape.Dispose();
if (render_face != null) render_face.Dispose();
if (seg_face != null) seg_face.Dispose();
if (landmarks != null) landmarks.Dispose();
if (pictureBox1.Image != null) pictureBox1.Image.Dispose();
StringBuilder msg = new StringBuilder(512);
int out_imgs_size = 0;
image = new Mat(image_path);
render_shape = new Mat();
render_face = new Mat();
seg_face = new Mat();
landmarks = new Mat();
stopwatch.Restart();
int res = forward(engine, image.CvPtr, msg, render_shape.CvPtr, render_face.CvPtr, seg_face.CvPtr, landmarks.CvPtr);
if (res == 0)
{
stopwatch.Stop();
double costTime = stopwatch.Elapsed.TotalMilliseconds;
pictureBox2.Image = new Bitmap(landmarks.ToMemoryStream());
Cv2.ImShow("render_shape", render_shape);
Cv2.ImShow("render_face", render_face);
Cv2.ImShow("seg_face", seg_face);
textBox1.Text = $"耗时:{costTime:F2}ms";
}
else
{
textBox1.Text = "识别失败";
}
button2.Enabled = true;
}
private void Form1_Load(object sender, EventArgs e)
{
startupPath = Application.StartupPath;
model_path = startupPath + "\\model\\";
StringBuilder msg = new StringBuilder(512);
int res = init(ref engine, model_path, msg);
if (res == -1)
{
MessageBox.Show(msg.ToString());
return;
}
else
{
Console.WriteLine(msg.ToString());
}
image_path = startupPath + "\\test_img\\1.jpg";
pictureBox1.Image = new Bitmap(image_path);
image = new Mat(image_path);
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
destroy(engine);
}
}
}
using OpenCvSharp;
using System;
using System.Diagnostics;
using System.Drawing;
using System.Runtime.InteropServices;
using System.Text;
using System.Windows.Forms;
namespace C__OpenCvSharp_部署3D人脸重建3DDFA_V3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
Stopwatch stopwatch = new Stopwatch();
Mat image;
string image_path;
string startupPath;
string model_path;
string fileFilter = "*.*|*.bmp;*.jpg;*.jpeg;*.tiff;*.tiff;*.png";
const string DllName = "3DDFA_V3Sharp.dll";
IntPtr engine;
/*
//初始化
extern "C" _declspec(dllexport) int __cdecl init(void** engine, char* model_path, char* msg);
//forward
extern "C" _declspec(dllexport) int __cdecl forward(void* engine, Mat* srcimg, char* msg, Mat* render_shape, Mat* render_face, Mat* seg_face, Mat* landmarks);
//释放
extern "C" _declspec(dllexport) void __cdecl destroy(void* engine);
*/
[DllImport(DllName, EntryPoint = "init", CallingConvention = CallingConvention.Cdecl)]
internal extern static int init(ref IntPtr engine, string model_path, StringBuilder msg);
[DllImport(DllName, EntryPoint = "forward", CallingConvention = CallingConvention.Cdecl)]
internal extern static int forward(IntPtr engine, IntPtr image, StringBuilder msg, IntPtr render_shape, IntPtr render_face, IntPtr seg_face, IntPtr landmarks);
[DllImport(DllName, EntryPoint = "destroy", CallingConvention = CallingConvention.Cdecl)]
internal extern static int destroy(IntPtr engine);
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd = new OpenFileDialog();
ofd.Filter = fileFilter;
if (ofd.ShowDialog() != DialogResult.OK) return;
pictureBox1.Image = null;
pictureBox2.Image = null;
textBox1.Text = "";
image_path = ofd.FileName;
pictureBox1.Image = new Bitmap(image_path);
image = new Mat(image_path);
}
Mat render_shape;
Mat render_face;
Mat seg_face;
Mat landmarks;
private void button2_Click(object sender, EventArgs e)
{
if (image_path == "")
{
return;
}
button2.Enabled = false;
Application.DoEvents();
Cv2.DestroyAllWindows();
if (image != null) image.Dispose();
if (render_shape != null) render_shape.Dispose();
if (render_face != null) render_face.Dispose();
if (seg_face != null) seg_face.Dispose();
if (landmarks != null) landmarks.Dispose();
if (pictureBox1.Image != null) pictureBox1.Image.Dispose();
StringBuilder msg = new StringBuilder(512);
int out_imgs_size = 0;
image = new Mat(image_path);
render_shape = new Mat();
render_face = new Mat();
seg_face = new Mat();
landmarks = new Mat();
stopwatch.Restart();
int res = forward(engine, image.CvPtr, msg, render_shape.CvPtr, render_face.CvPtr, seg_face.CvPtr, landmarks.CvPtr);
if (res == 0)
{
stopwatch.Stop();
double costTime = stopwatch.Elapsed.TotalMilliseconds;
pictureBox2.Image = new Bitmap(landmarks.ToMemoryStream());
Cv2.ImShow("render_shape", render_shape);
Cv2.ImShow("render_face", render_face);
Cv2.ImShow("seg_face", seg_face);
textBox1.Text = $"耗时:{costTime:F2}ms";
}
else
{
textBox1.Text = "识别失败";
}
button2.Enabled = true;
}
private void Form1_Load(object sender, EventArgs e)
{
startupPath = Application.StartupPath;
model_path = startupPath + "\\model\\";
StringBuilder msg = new StringBuilder(512);
int res = init(ref engine, model_path, msg);
if (res == -1)
{
MessageBox.Show(msg.ToString());
return;
}
else
{
Console.WriteLine(msg.ToString());
}
image_path = startupPath + "\\test_img\\1.jpg";
pictureBox1.Image = new Bitmap(image_path);
image = new Mat(image_path);
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
destroy(engine);
}
}
}