✨个人主页:熬夜学编程的小林
💗系列专栏: 【C语言详解】 【数据结构详解】【C++详解】【Linux系统编程】【MySQL】
目录
[1 表的增删改查](#1 表的增删改查)
[1.1 Create](#1.1 Create)
[1.1.1 单行数据 + 全列插入](#1.1.1 单行数据 + 全列插入)
[1.1.2 多行数据 + 指定列插入](#1.1.2 多行数据 + 指定列插入)
[1.1.3 插入否则更新](#1.1.3 插入否则更新)
[1.1.4 替换](#1.1.4 替换)
[1.2 Retrieve](#1.2 Retrieve)
[1.2.1 SELECT 列](#1.2.1 SELECT 列)
[1.2.1.1 全列查询](#1.2.1.1 全列查询)
[1.2.1.2 指定列查询](#1.2.1.2 指定列查询)
[1.2.1.3 查询字段为表达式](#1.2.1.3 查询字段为表达式)
[1.2.1.4 为查询结果指定别名](#1.2.1.4 为查询结果指定别名)
[1.2.1.5 结果去重](#1.2.1.5 结果去重)
[1.2.2 WHERE 条件](#1.2.2 WHERE 条件)
[1.2.3 结果排序](#1.2.3 结果排序)
[1.2.4 筛选分页结果](#1.2.4 筛选分页结果)
1 表的增删改查
CRUD : Create (创建), Retrieve (读取),Update (更新),Delete(删除)
1.1 Create
语法:
INSERT [INTO] table_name
[(column [, column] ...)]
VALUES (value_list) [, (value_list)] ...
value_list: value, [, value] ...
案例:
创建学生表
mysql> create table student(
id int unsigned primary key auto_increment,
sn int not null unique comment '学号',
name varchar(20) not null,
qq varchar(15) unique);
Query OK, 0 rows affected (0.04 sec)
mysql> desc student;
+-------+------------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------+------------------+------+-----+---------+----------------+
| id | int(10) unsigned | NO | PRI | NULL | auto_increment |
| sn | int(11) | NO | UNI | NULL | |
| name | varchar(20) | NO | | NULL | |
| qq | varchar(15) | YES | UNI | NULL | |
+-------+------------------+------+-----+---------+----------------+
4 rows in set (0.00 sec)
1.1.1 单行数据 + 全列插入
- 插入两条记录,value_list 数量必须和定义表的列的数量及顺序一致。
- 注意,这里在插入的时候,也可以不用指定id (当然,那时候就需要明确插入数据到那些列了),那么mysql会使用默认的值进行自增。
mysql> insert into student values(1,123,'张三',123456);
Query OK, 1 row affected (0.01 sec)
mysql> insert into student values(2,124,'李四',156954);
Query OK, 1 row affected (0.01 sec)
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 123 | 张三 | 123456 |
| 2 | 124 | 李四 | 156954 |
+----+-----+--------+--------+
2 rows in set (0.00 sec)
1.1.2 多行数据 + 指定列插入
mysql> insert into student (sn,name,qq) values(125,'王五',523124); # 不指定id列,默认+1插入
Query OK, 1 row affected (0.01 sec)
mysql> insert into student (sn,name,qq) values(126,'赵六',529124);
Query OK, 1 row affected (0.00 sec)
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 123 | 张三 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 赵六 | 529124 |
+----+-----+--------+--------+
4 rows in set (0.00 sec)
1.1.3 插入否则更新
由于 主键 或者 唯一键 对应的值已经存在而导致插入失败。
# 主键冲突,也有唯一键冲突
mysql> insert into student (id,sn,name,qq) values(4,126,'赵六',529124);
ERROR 1062 (23000): Duplicate entry '4' for key 'PRIMARY'
// 唯一键冲突
mysql> insert into student (sn,name,qq) values(126,'赵六',529124);
ERROR 1062 (23000): Duplicate entry '126' for key 'sn'
可以选择性的进行同步更新操作 语法:
INSERT ... ON DUPLICATE KEY UPDATE
column = value [, column = value] ...
2 row affected
mysql> insert into student values(1,127,'孙权',456543); # 插入重复的主键值不允许插入
ERROR 1062 (23000): Duplicate entry '1' for key 'PRIMARY'
mysql> insert into student values(1,127,'孙权',456543) on duplicate key update sn=127,name='孙权'; # 如果主键值重复就更新主键内容
Query OK, 2 rows affected (0.01 sec)
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 127 | 孙权 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 赵六 | 529124 |
+----+-----+--------+--------+
4 rows in set (0.00 sec)
2 row affected : 表中有冲突数据,并且数据已经被更新
1 row affected
mysql> insert into student values(5,128,'刘备',165463) on duplicate key update sn=128,name='刘备'; # 插入不重复的主键内容,且唯一键也不冲突
Query OK, 1 row affected (0.02 sec)
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 127 | 孙权 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 赵六 | 529124 |
| 5 | 128 | 刘备 | 165463 |
+----+-----+--------+--------+
5 rows in set (0.00 sec)
1 row affected : 表中没有冲突数据,数据被插入。
0 row affected:
mysql> insert into student values(5,128,'刘备',165463) on duplicate key update sn=128,name='刘备'; # 执行上一次插入语句,冲突数值与update的值相同
Query OK, 0 rows affected (0.00 sec)
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 127 | 孙权 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 赵六 | 529124 |
| 5 | 128 | 刘备 | 165463 |
+----+-----+--------+--------+
5 rows in set (0.00 sec)
0 row affected: 表中有冲突数据,但冲突数据的值和 update 的值相等
通过 MySQL 函数 row_count() 获取受到影响的数据行数
mysql> insert into student values(5,128,'刘备',165463) on duplicate key update sn=128,name='刘备';
Query OK, 0 rows affected (0.00 sec)
mysql> select row_count();
+-------------+
| row_count() |
+-------------+
| 0 |
+-------------+
1 row in set (0.00 sec)
1.1.4 替换
主键 或者 唯一键没有冲突 ,则直接插入 ;
主键 或者 唯一键 如果冲突 ,则删除后再插入;
没有冲突
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 127 | 孙权 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 赵六 | 529124 |
| 5 | 128 | 刘备 | 165463 |
+----+-----+--------+--------+
5 rows in set (0.00 sec)
mysql> replace into student values(6,129,'妲己',596241);# 没有冲突情况
Query OK, 1 row affected (0.00 sec)
mysql> select * from student; # 直接插入数据到表中
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 127 | 孙权 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 赵六 | 529124 |
| 5 | 128 | 刘备 | 165463 |
| 6 | 129 | 妲己 | 596241 |
+----+-----+--------+--------+
6 rows in set (0.00 sec)
有冲突
mysql> replace into student values(6,130,'张飞',544562); # 主键id冲突,先删除再插入
Query OK, 2 rows affected (0.00 sec)
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 127 | 孙权 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 赵六 | 529124 |
| 5 | 128 | 刘备 | 165463 |
| 6 | 130 | 张飞 | 544562 |
+----+-----+--------+--------+
6 rows in set (0.00 sec)
1.2 Retrieve
语法:
SELECT
[DISTINCT] {* | {column [, column] ...}
[FROM table_name]
[WHERE ...]
[ORDER BY column [ASC | DESC], ...]
LIMIT ...
案例:
新建表
mysql> CREATE TABLE exam_result (
id INT UNSIGNED PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(20) NOT NULL COMMENT '同学姓名',
chinese float DEFAULT 0.0 COMMENT '语文成绩',
math float DEFAULT 0.0 COMMENT '数学成绩',
english float DEFAULT 0.0 COMMENT '英语成绩' );
Query OK, 0 rows affected (0.05 sec)
mysql> desc exam_result;
+---------+------------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+---------+------------------+------+-----+---------+----------------+
| id | int(10) unsigned | NO | PRI | NULL | auto_increment |
| name | varchar(20) | NO | | NULL | |
| chinese | float | YES | | 0 | |
| math | float | YES | | 0 | |
| english | float | YES | | 0 | |
+---------+------------------+------+-----+---------+----------------+
5 rows in set (0.00 sec)
插入数据
mysql> INSERT INTO exam_result (name, chinese, math, english) VALUES
-> ('唐三藏', 67, 98, 56),
-> ('孙悟空', 87, 78, 77),
-> ('猪悟能', 88, 98, 90),
-> ('曹孟德', 82, 84, 67),
-> ('刘玄德', 55, 85, 45),
-> ('孙权', 70, 73, 78),
-> ('宋公明', 75, 65, 30);
Query OK, 7 rows affected (0.01 sec)
Records: 7 Duplicates: 0 Warnings: 0
mysql> select * from exam_result;
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 1 | 唐三藏 | 67 | 98 | 56 |
| 2 | 孙悟空 | 87 | 78 | 77 |
| 3 | 猪悟能 | 88 | 98 | 90 |
| 4 | 曹孟德 | 82 | 84 | 67 |
| 5 | 刘玄德 | 55 | 85 | 45 |
| 6 | 孙权 | 70 | 73 | 78 |
| 7 | 宋公明 | 75 | 65 | 30 |
+----+-----------+---------+------+---------+
7 rows in set (0.00 sec)
1.2.1 SELECT 列
1.2.1.1 全列查询
-- 通常情况下不建议使用 * 进行全列查询
-- 1. 查询的列越多,意味着需要传输的数据量越大;
-- 2. 可能会影响到索引的使用。
mysql> select * from exam_result; # 使用*(类似通配符)全列查询
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 1 | 唐三藏 | 67 | 98 | 56 |
| 2 | 孙悟空 | 87 | 78 | 77 |
| 3 | 猪悟能 | 88 | 98 | 90 |
| 4 | 曹孟德 | 82 | 84 | 67 |
| 5 | 刘玄德 | 55 | 85 | 45 |
| 6 | 孙权 | 70 | 73 | 78 |
| 7 | 宋公明 | 75 | 65 | 30 |
+----+-----------+---------+------+---------+
7 rows in set (0.00 sec)
1.2.1.2 指定列查询
指定列的顺序不需要按定义表的顺序来。
mysql> select name,id,chinese from exam_result;
+-----------+----+---------+
| name | id | chinese |
+-----------+----+---------+
| 唐三藏 | 1 | 67 |
| 孙悟空 | 2 | 87 |
| 猪悟能 | 3 | 88 |
| 曹孟德 | 4 | 82 |
| 刘玄德 | 5 | 55 |
| 孙权 | 6 | 70 |
| 宋公明 | 7 | 75 |
+-----------+----+---------+
7 rows in set (0.00 sec)
1.2.1.3 查询字段为表达式
表达式不包含字段
mysql> select name,id,10 from exam_result;
+-----------+----+----+
| name | id | 10 |
+-----------+----+----+
| 唐三藏 | 1 | 10 |
| 孙悟空 | 2 | 10 |
| 猪悟能 | 3 | 10 |
| 曹孟德 | 4 | 10 |
| 刘玄德 | 5 | 10 |
| 孙权 | 6 | 10 |
| 宋公明 | 7 | 10 |
+-----------+----+----+
7 rows in set (0.00 sec)
表达式包含一个字段
mysql> select name,chinese,10+chinese from exam_result;
+-----------+---------+------------+
| name | chinese | 10+chinese |
+-----------+---------+------------+
| 唐三藏 | 67 | 77 |
| 孙悟空 | 87 | 97 |
| 猪悟能 | 88 | 98 |
| 曹孟德 | 82 | 92 |
| 刘玄德 | 55 | 65 |
| 孙权 | 70 | 80 |
| 宋公明 | 75 | 85 |
+-----------+---------+------------+
7 rows in set (0.00 sec)
表达式包含多个字段
mysql> select name,chinese,math,chinese+math from exam_result;
+-----------+---------+------+--------------+
| name | chinese | math | chinese+math |
+-----------+---------+------+--------------+
| 唐三藏 | 67 | 98 | 165 |
| 孙悟空 | 87 | 78 | 165 |
| 猪悟能 | 88 | 98 | 186 |
| 曹孟德 | 82 | 84 | 166 |
| 刘玄德 | 55 | 85 | 140 |
| 孙权 | 70 | 73 | 143 |
| 宋公明 | 75 | 65 | 140 |
+-----------+---------+------+--------------+
7 rows in set (0.00 sec)
1.2.1.4 为查询结果指定别名
语法:
sql
SELECT column [AS] alias_name [...] FROM table_name;
as可以省略。
sql
mysql> select name,chinese+math+english 总分 from exam_result;
+-----------+--------+
| name | 总分 |
+-----------+--------+
| 唐三藏 | 221 |
| 孙悟空 | 242 |
| 猪悟能 | 276 |
| 曹孟德 | 233 |
| 刘玄德 | 185 |
| 孙权 | 221 |
| 宋公明 | 170 |
+-----------+--------+
7 rows in set (0.00 sec)
1.2.1.5 结果去重
sql
# 98重复了
mysql> select math from exam_result;
+------+
| math |
+------+
| 98 |
| 78 |
| 98 |
| 84 |
| 85 |
| 73 |
| 65 |
+------+
7 rows in set (0.00 sec)
# distinct去重
mysql> select distinct math from exam_result;
+------+
| math |
+------+
| 98 |
| 78 |
| 84 |
| 85 |
| 73 |
| 65 |
+------+
6 rows in set (0.00 sec)
1.2.2 WHERE 条件
比较运算符:
运算符 | 说明 |
---|---|
>, >=, <, <= | 大于,大于等于,小于,小于等于 |
= | 等于,NULL 不安全,例如 NULL = NULL 的结果是 NULL |
<=> | 等于,NULL 安全,例如 NULL <=> NULL 的结果是 TRUE(1) |
!=, <> | 不等于 |
BETWEEN a0 AND a1 | 范围匹配,[a0, a1],如果 a0 <= value <= a1,返回 TRUE(1) |
IN (option, ...) | 如果是 option 中的任意一个,返回 TRUE(1) |
IS NULL | 是 NULL |
IS NOT NULL | 不是 NULL |
LIKE | 模糊匹配。% 表示任意多个(包括 0 个)任意字符;_ 表示任意一个字符 |
逻辑运算符:
运算符 | 说明 |
---|---|
AND | 多个条件必须都为 TRUE(1),结果才是 TRUE(1) |
OR | 任意一个条件为 TRUE(1), 结果为 TRUE(1) |
NOT | 条件为 TRUE(1),结果为 FALSE(0) |
英语不及格的同学及英语成绩 ( < 60 )
mysql> select name,english from exam_result where english < 60;
+-----------+---------+
| name | english |
+-----------+---------+
| 唐三藏 | 56 |
| 刘玄德 | 45 |
| 宋公明 | 30 |
+-----------+---------+
3 rows in set (0.00 sec)
语文成绩在 [80, 90] 分的同学及语文成绩
使用 AND 进行条件连接
mysql> select name,chinese from exam_result where chinese >= 80 and chinese <= 90;
+-----------+---------+
| name | chinese |
+-----------+---------+
| 孙悟空 | 87 |
| 猪悟能 | 88 |
| 曹孟德 | 82 |
+-----------+---------+
3 rows in set (0.00 sec)
使用 BETWEEN ... AND ... 条件
mysql> select name,chinese from exam_result where chinese between 80 and 90;
+-----------+---------+
| name | chinese |
+-----------+---------+
| 孙悟空 | 87 |
| 猪悟能 | 88 |
| 曹孟德 | 82 |
+-----------+---------+
3 rows in set (0.00 sec)
数学成绩是 58 或者 59 或者 98 或者 99 分的同学及数学成绩
使用 OR 进行条件连接
mysql> select name,math from exam_result where math=58 or math=59 or math=98 or math=99;
+-----------+------+
| name | math |
+-----------+------+
| 唐三藏 | 98 |
| 猪悟能 | 98 |
+-----------+------+
2 rows in set (0.00 sec)
使用 IN 条件
mysql> select name,math from exam_result where math in(58,59,98,99);
+-----------+------+
| name | math |
+-----------+------+
| 唐三藏 | 98 |
| 猪悟能 | 98 |
+-----------+------+
2 rows in set (0.00 sec)
姓孙的同学 及 孙某同学
姓孙表示只有第一个字是孙即可,孙某表示名字只有两个字且第一个字为孙。
% 匹配任意多个(包括 0 个)任意字符
mysql> select name from exam_result where name like '孙%';
+-----------+
| name |
+-----------+
| 孙悟空 |
| 孙权 |
+-----------+
2 rows in set (0.00 sec)
_ 匹配严格的一个任意字符
mysql> select name from exam_result where name like '孙_';
+--------+
| name |
+--------+
| 孙权 |
+--------+
1 row in set (0.00 sec)
语文成绩好于英语成绩的同学
mysql> select name,chinese,english from exam_result where chinese > english;
+-----------+---------+---------+
| name | chinese | english |
+-----------+---------+---------+
| 唐三藏 | 67 | 56 |
| 孙悟空 | 87 | 77 |
| 曹孟德 | 82 | 67 |
| 刘玄德 | 55 | 45 |
| 宋公明 | 75 | 30 |
+-----------+---------+---------+
5 rows in set (0.00 sec)
总分在 200 分以下的同学
1、查询总分和名字,并将总分起别名
mysql> select name,chinese+math+english total from exam_result;
+-----------+-------+
| name | total |
+-----------+-------+
| 唐三藏 | 221 |
| 孙悟空 | 242 |
| 猪悟能 | 276 |
| 曹孟德 | 233 |
| 刘玄德 | 185 |
| 孙权 | 221 |
| 宋公明 | 170 |
+-----------+-------+
7 rows in set (0.00 sec)
2、查询总分小于200的同学
mysql> select name,chinese+math+english total from exam_result where total<200;
ERROR 1054 (42S22): Unknown column 'total' in 'where clause'
直接使用别名来进行条件比较报错,为什么呢?
因为select 后面的列是显示到屏幕上的,也就是进行筛选之后才打印到屏幕上的,一般情况都是最后才执行,因此我们可以得出结论:先执行from ,再执行where ,最后执行select,因此使用别名会报错。

正确做法(别名不能用在 WHERE 条件中):
mysql> select name,chinese+math+english total from exam_result where chinese+math+english<200;
+-----------+-------+
| name | total |
+-----------+-------+
| 刘玄德 | 185 |
| 宋公明 | 170 |
+-----------+-------+
2 rows in set (0.00 sec)
语文成绩 > 80 并且不姓孙的同学
AND 与 NOT 的使用
mysql> select name,chinese from exam_result where chinese>80 and name not like '孙%';
+-----------+---------+
| name | chinese |
+-----------+---------+
| 猪悟能 | 88 |
| 曹孟德 | 82 |
+-----------+---------+
2 rows in set (0.00 sec)
孙某同学,否则要求总成绩 > 200 并且 语文成绩 < 数学成绩 并且 英语成绩 > 80
综合性查询
mysql> select name,chinese,math,english from exam_result where name like '孙_' or (chinese+math+english>200 and chinese<math and english >80);
+-----------+---------+------+---------+
| name | chinese | math | english |
+-----------+---------+------+---------+
| 猪悟能 | 88 | 98 | 90 |
| 孙权 | 70 | 73 | 78 |
+-----------+---------+------+---------+
2 rows in set (0.00 sec)
NULL 的查询
创建一个表
mysql> create table student(
id int unsigned primary key auto_increment,
sn int not null unique comment '学号',
name varchar(20) not null,
qq varchar(15) unique);
Query OK, 0 rows affected (0.03 sec)
mysql> desc student;
+-------+------------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------+------------------+------+-----+---------+----------------+
| id | int(10) unsigned | NO | PRI | NULL | auto_increment |
| sn | int(11) | NO | UNI | NULL | |
| name | varchar(20) | NO | | NULL | |
| qq | varchar(15) | YES | UNI | NULL | |
+-------+------------------+------+-----+---------+----------------+
4 rows in set (0.00 sec)
插入数据
mysql> insert into student values(1,123,'张三',123456);
Query OK, 1 row affected (0.00 sec)
mysql> insert into student values(2,124,'李四',156954);
Query OK, 1 row affected (0.00 sec)
mysql> insert into student (sn,name,qq) values(125,'王五',523124);
Query OK, 1 row affected (0.01 sec)
mysql> insert into student (sn,name,qq) values(126,'孙权',NULL);
Query OK, 1 row affected (0.00 sec)
mysql> insert into student (sn,name,qq) values(127,'妲己',NULL);
Query OK, 1 row affected (0.00 sec)
mysql> select * from student;
+----+-----+--------+--------+
| id | sn | name | qq |
+----+-----+--------+--------+
| 1 | 123 | 张三 | 123456 |
| 2 | 124 | 李四 | 156954 |
| 3 | 125 | 王五 | 523124 |
| 4 | 126 | 孙权 | NULL |
| 5 | 127 | 妲己 | NULL |
+----+-----+--------+--------+
5 rows in set (0.00 sec)
查询 qq 号已知(不为空)的同学姓名
mysql> select name,qq from student where qq is not null;
+--------+--------+
| name | qq |
+--------+--------+
| 张三 | 123456 |
| 李四 | 156954 |
| 王五 | 523124 |
+--------+--------+
3 rows in set (0.00 sec)
NULL 和 NULL 的比较,= 和 <=> 的区别
mysql> select null=null,null=0,null=1;
+-----------+--------+--------+
| null=null | null=0 | null=1 |
+-----------+--------+--------+
| NULL | NULL | NULL |
+-----------+--------+--------+
1 row in set (0.00 sec)
mysql> select null<=>null,null<=>0,null<=>1;
+-------------+----------+----------+
| null<=>null | null<=>0 | null<=>1 |
+-------------+----------+----------+
| 1 | 0 | 0 |
+-------------+----------+----------+
1 row in set (0.00 sec)
null使用=进行比较结果永远为空,null使用<=>比较返回值为0或者1,0表示假,1表示真。
1.2.3 结果排序
-- ASC (ascending order) 为升序 (从小到大)
-- DESC (descending)为降序 (从大到小)
-- 默认为 ASC(升序)
语法:
SELECT ... FROM table_name [WHERE ...]
ORDER BY column [ASC|DESC], [...];
注意:没有 ORDER BY 子句的查询,返回的顺序是未定义的,永远不要依赖这个顺序。
案例:
同学及数学成绩,按数学成绩升序显示
mysql> select name,math from exam_result order by math asc;
+-----------+------+
| name | math |
+-----------+------+
| 宋公明 | 65 |
| 孙权 | 73 |
| 孙悟空 | 78 |
| 曹孟德 | 84 |
| 刘玄德 | 85 |
| 唐三藏 | 98 |
| 猪悟能 | 98 |
+-----------+------+
7 rows in set (0.00 sec)
默认升序,也可以不写asc。
同学及 qq 号,按 qq 号排序显示
NULL 视为比任何值都小,升序出现在最上面
mysql> select name,qq from student order by qq;
+--------+--------+
| name | qq |
+--------+--------+
| 孙权 | NULL |
| 妲己 | NULL |
| 张三 | 123456 |
| 李四 | 156954 |
| 王五 | 523124 |
+--------+--------+
5 rows in set (0.00 sec)
NULL 视为比任何值都小,降序出现在最下面
mysql> select name,qq from student order by qq desc;
+--------+--------+
| name | qq |
+--------+--------+
| 王五 | 523124 |
| 李四 | 156954 |
| 张三 | 123456 |
| 孙权 | NULL |
| 妲己 | NULL |
+--------+--------+
5 rows in set (0.00 sec)
查询同学各门成绩,依次按 数学降序,英语升序,语文升序的方式显示
先按照数学降序,如果数学成绩相同则按照英语升序,如果英语成绩相同则按照语文升序。
mysql> select name,chinese,math,english from exam_result order by math desc,english,chinese;
+-----------+---------+------+---------+
| name | chinese | math | english |
+-----------+---------+------+---------+
| 唐三藏 | 67 | 98 | 56 |
| 猪悟能 | 88 | 98 | 90 |
| 刘玄德 | 55 | 85 | 45 |
| 曹孟德 | 82 | 84 | 67 |
| 孙悟空 | 87 | 78 | 77 |
| 孙权 | 70 | 73 | 78 |
| 宋公明 | 75 | 65 | 30 |
+-----------+---------+------+---------+
7 rows in set (0.00 sec)
多字段排序,排序优先级随书写顺序。
查询同学及总分,由高到低
mysql> select name,chinese+math+english total from exam_result order by total desc;
+-----------+-------+
| name | total |
+-----------+-------+
| 猪悟能 | 276 |
| 孙悟空 | 242 |
| 曹孟德 | 233 |
| 唐三藏 | 221 |
| 孙权 | 221 |
| 刘玄德 | 185 |
| 宋公明 | 170 |
+-----------+-------+
7 rows in set (0.00 sec)
为什么这里可以使用别名排序,而不能使用别用条件查询?
因为得先需要有数据才能进行排序,得通过条件查询出数据之后,再对该数据进行排序,排序之后再显示到屏幕上。
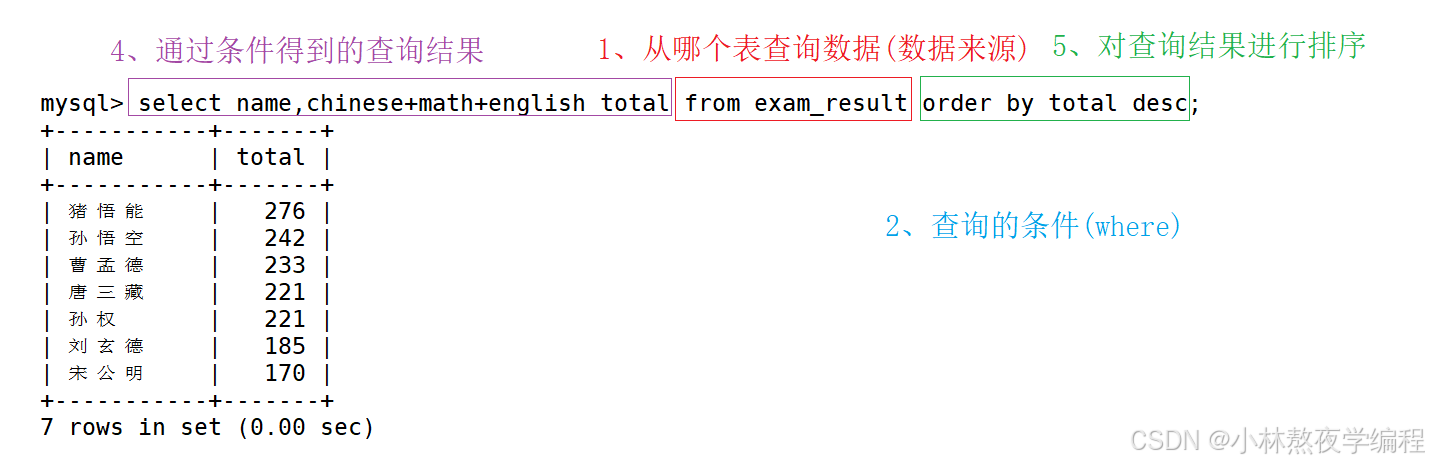
查询姓孙的同学或者姓曹的同学数学成绩,结果按数学成绩由高到低显示
结合 WHERE 子句 和 ORDER BY 子句
mysql> select name,math from exam_result where name like '孙%' or name like '曹%' order by math desc;
+-----------+------+
| name | math |
+-----------+------+
| 曹孟德 | 84 |
| 孙悟空 | 78 |
| 孙权 | 73 |
+-----------+------+
3 rows in set (0.00 sec)
1.2.4 筛选分页结果
语法:
-- 起始下标为 0
-- 从 s 开始,筛选 n 条结果
SELECT ... FROM table_name [WHERE ...] [ORDER BY ...] LIMIT s, n;
-- 从 0 开始,筛选 n 条结果
SELECT ... FROM table_name [WHERE ...] [ORDER BY ...] LIMIT n;
-- 从 s 开始,筛选 n 条结果,比第二种用法更明确,建议使用
SELECT ... FROM table_name [WHERE ...] [ORDER BY ...] LIMIT n OFFSET s;
建议:对未知表进行查询时,最好加一条 LIMIT 1,避免因为表中数据过大,查询全表数据导致数据库卡死
按 id 进行分页,每页 3 条记录,分别显示 第 1、2、3 页
先将id排升序,然后对数据进行分页。
方式一:
第一页
mysql> select id,name,chinese,math,english from exam_result order by id limit 3 offset 0;
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 1 | 唐三藏 | 67 | 98 | 56 |
| 2 | 孙悟空 | 87 | 78 | 77 |
| 3 | 猪悟能 | 88 | 98 | 90 |
+----+-----------+---------+------+---------+
3 rows in set (0.00 sec)
第二页
mysql> select id,name,chinese,math,english from exam_result order by id limit 3 offset 3;
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 4 | 曹孟德 | 82 | 84 | 67 |
| 5 | 刘玄德 | 55 | 85 | 45 |
| 6 | 孙权 | 70 | 73 | 78 |
+----+-----------+---------+------+---------+
3 rows in set (0.00 sec)
第三页
如果结果不足 3 个,不会有影响。
mysql> select id,name,chinese,math,english from exam_result order by id limit 3 offset 6;
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 7 | 宋公明 | 75 | 65 | 30 |
+----+-----------+---------+------+---------+
1 row in set (0.00 sec)
方式二:
limit后面只有一个数字,默认从0开始查询3条数据。
第一页
mysql> select id,name,chinese,math,english from exam_result order by id limit 3;
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 1 | 唐三藏 | 67 | 98 | 56 |
| 2 | 孙悟空 | 87 | 78 | 77 |
| 3 | 猪悟能 | 88 | 98 | 90 |
+----+-----------+---------+------+---------+
3 rows in set (0.00 sec)
第二页
mysql> select id,name,chinese,math,english from exam_result order by id limit 3,3;
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 4 | 曹孟德 | 82 | 84 | 67 |
| 5 | 刘玄德 | 55 | 85 | 45 |
| 6 | 孙权 | 70 | 73 | 78 |
+----+-----------+---------+------+---------+
3 rows in set (0.00 sec)
第三页
mysql> select id,name,chinese,math,english from exam_result order by id limit 6,3;
+----+-----------+---------+------+---------+
| id | name | chinese | math | english |
+----+-----------+---------+------+---------+
| 7 | 宋公明 | 75 | 65 | 30 |
+----+-----------+---------+------+---------+
1 row in set (0.00 sec)