思路
这里n叉树的层序遍历和二叉树的层序遍历非常相似,可以参考博文:二叉树的层序遍历
N叉树对比二叉树就是有多个孩子节点,二叉树是有一个left和一个right节点,n叉树因为有多个子节点,所以通过children
来存放所有的孩子节点,然后在层级遍历的时候,要遍历children中的所有元素入队列
可能一开始看到题目的时候不太明白构造出来的二叉树和N叉树的一个数据结构,下面模拟一下构造逻辑
二叉树的构造
js
class TreeNode {
constructor(val) {
this.val = val;
this.left = null;
this.right = null;
}
}
const root = new TreeNode(5)
root.left = new TreeNode(4)
root.right = new TreeNode(6)
root.left.left = new TreeNode(1)
root.left.right = new TreeNode(2)
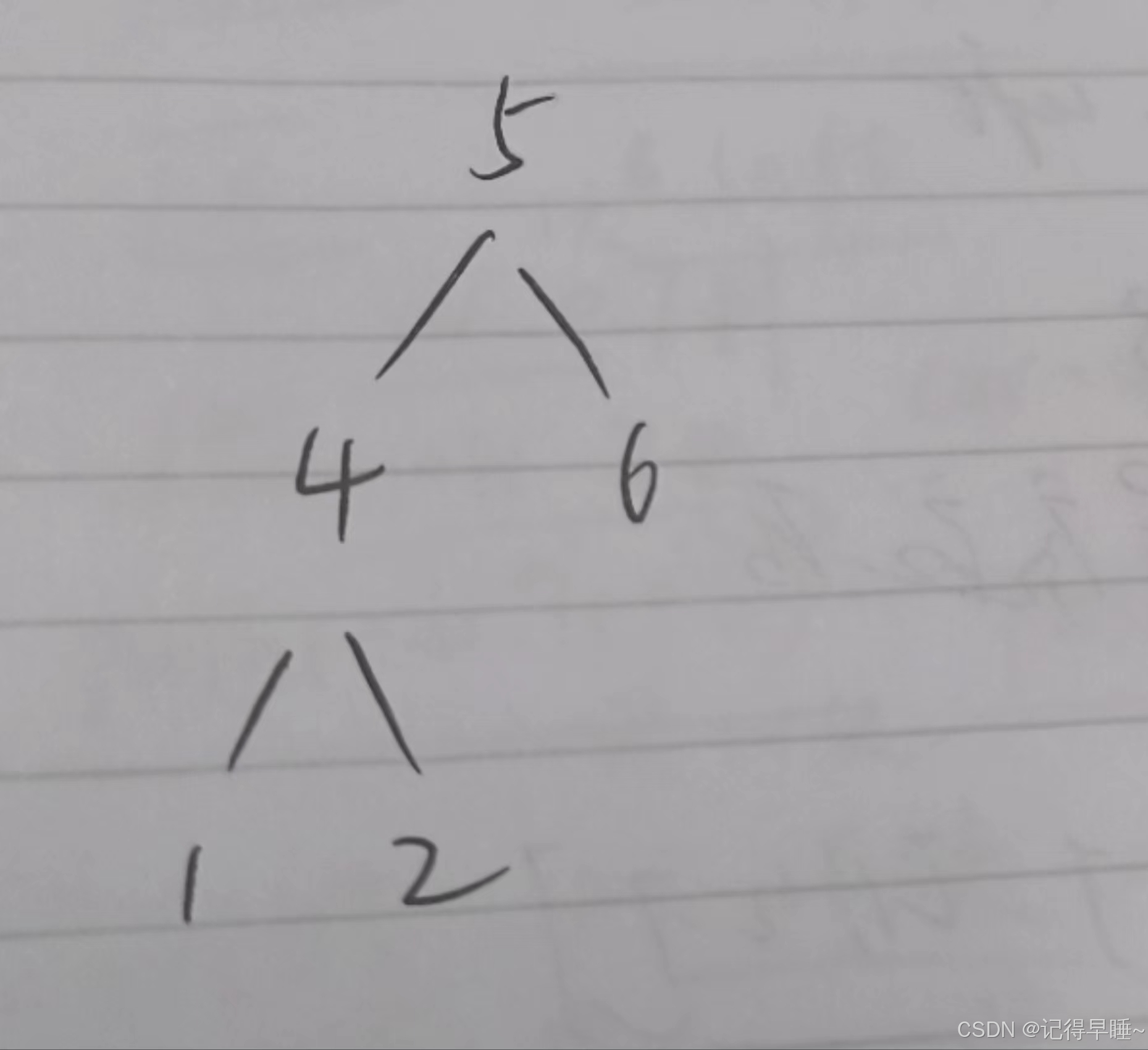
N叉树的构造
js
class TreeNode {
constructor(val) {
this.val = val;
this.children = null
}
}
const root = new TreeNode(1)
root.children = [new TreeNode(3),new TreeNode(2),new TreeNode(4)]
root.children[0].children = [new TreeNode(5),new TreeNode(6)]
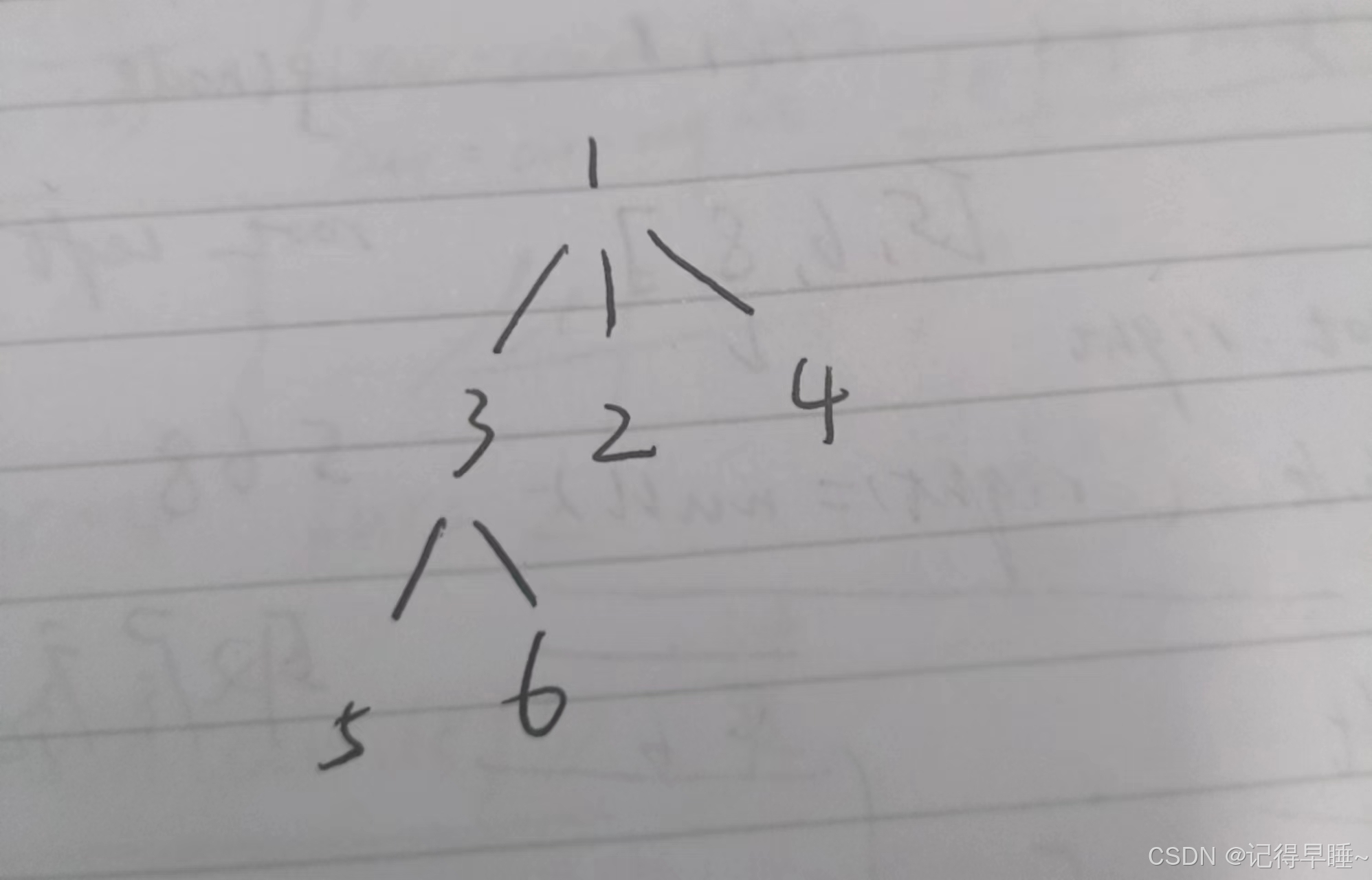
实现
js
var levelOrder = function (root) {
if (!root) return [];
let result = [], queue = [root];
while (queue.length) {
let len = queue.length;
let arr = [];
while (len--) {
let node = queue.shift();
arr.push(node.val);
if (node.children) {
for (const item of node.children) {
queue.push(item)
}
}
}
result.push(arr)
}
return result
};