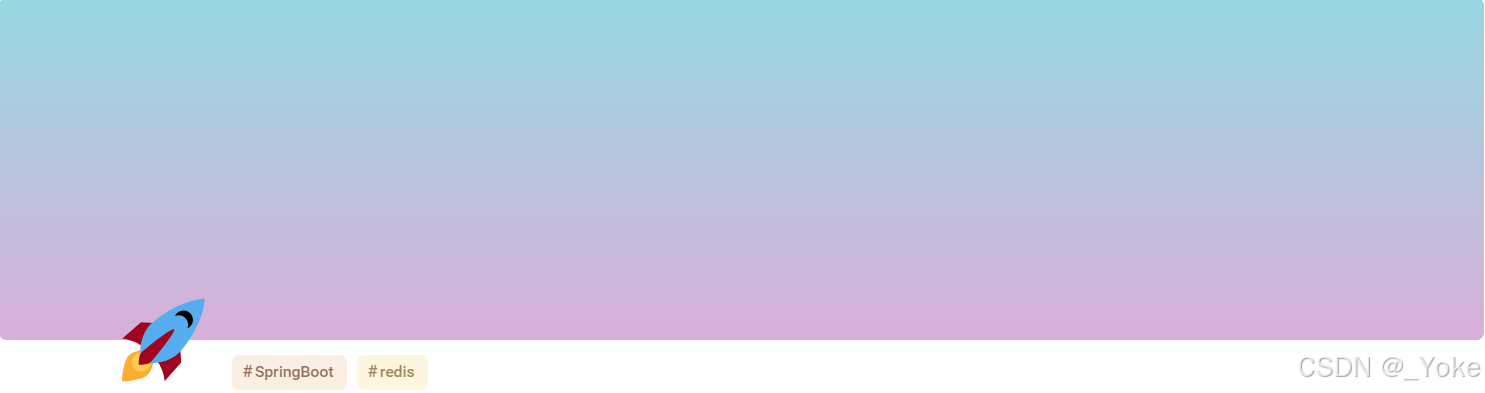
Spring Boot 缓存最佳实践:从基础到生产的完整指南
引言
在现代分布式系统中,缓存是提升系统性能的银弹。Spring Boot 通过 spring-boot-starter-cache
模块提供了开箱即用的缓存抽象,但如何根据业务需求实现灵活、可靠的缓存方案?本文将带您从零开始,逐步构建符合生产要求的缓存系统。
一、基础篇:5分钟快速接入
1.1 最小化配置
pom.xml 依赖
xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
Nacos 配置(application.yml)
yaml
spring:
cache:
type: simple # 默认内存缓存
启动类注解
java
@SpringBootApplication
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
业务层使用
java
@Service
public class ProductService {
@Cacheable("products")
public Product getProduct(Long id) {
// 数据库查询逻辑
}
}
二、进阶篇:多缓存引擎支持
2.1 缓存类型切换
配置选项对比
类型 | 依赖 | 适用场景 | 特点 |
---|---|---|---|
simple |
内置 | 开发测试环境 | 无过期策略 |
caffeine |
com.github.ben-manes.caffeine | 高性能本地缓存 | 支持多种过期策略 |
redis |
spring-boot-starter-data-redis | 分布式生产环境 | 支持持久化、集群 |
Nacos 配置示例
yaml
spring:
cache:
type: redis # 切换缓存引擎
# Redis 连接配置
redis:
host: redis.prod.cluster
port: 6379
password: ${REDIS_PASSWORD}
三、生产级特性实现
3.1 方法级 TTL 控制
实现方式1:语法约定
语法约定
java
@Cacheable("热点数据#600") // 600秒过期
public HotData getHotData(String key) {
// 业务逻辑
}
TTL 解析实现
java
public class CacheConfig {
@Bean
public CacheManagerCustomizer<RedisCacheManager> redisCacheCustomizer() {
return manager -> manager.setCacheDecorator((name, config) -> {
String[] parts = name.split("#");
if (parts.length > 1) {
Duration ttl = Duration.ofSeconds(Long.parseLong(parts[1]));
return new RedisCacheWrapper(parts[0], config.entryTtl(ttl));
}
return new RedisCacheWrapper(name, config);
});
}
}
实现方式2:自定义注解+AOP切面
- 定义自定义注解
java
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface CacheCustomTtl {
long value(); // 缓存时间(秒)
long jitter() default 10; // 抖动范围(秒)
//....自定义其他逻辑
}
- aop切面逻辑
java
@Aspect
@Component
public class CacheTtlAspect {
@Around("@annotation(cacheCustomTtl)")
public Object applyCustomTtl(ProceedingJoinPoint joinPoint, CacheCustomTtl cacheCustomTtl) throws Throwable {
// 获取原始缓存配置
Method method = ((MethodSignature) joinPoint.getSignature()).getMethod();
Cacheable cacheable = method.getAnnotation(Cacheable.class);
String[] cacheNames = cacheable.value();
// 生成带自定义时间的缓存名称(例如: user#3600)
String newCacheName = cacheNames[0] + "#" + cacheCustomTtl.value();
String[] modifiedCacheNames = {newCacheName};
// 动态修改缓存名称
Cacheable modifiedCacheable = new CacheableWrapper(cacheable, modifiedCacheNames);
((MethodSignature) joinPoint.getSignature()).getMethod().getAnnotation(Cacheable.class).value();
// 通过反射调用原方法(需使用动态代理或工具类)
return joinPoint.proceed();
}
// 包装类用于动态修改注解属性
private static class CacheableWrapper implements Cacheable {
private final Cacheable delegate;
private final String[] cacheNames;
public CacheableWrapper(Cacheable delegate, String[] cacheNames) {
this.delegate = delegate;
this.cacheNames = cacheNames;
}
@Override
public String[] value() { return cacheNames; }
// 其他方法委托给原注解...
}
}
3.2 随机抖动(Jitter)
防雪崩配置
yaml
spring:
cache:
jitter-range: 60s # 最大抖动时间范围
抖动值生成逻辑
java
private Duration applyJitter(Duration ttl) {
long jitter = ThreadLocalRandom.current().nextLong(
spring.cache.jitter-range.getSeconds() + 1
);
return ttl.plusSeconds(jitter);
}
四、高级优化方案
4.1 多级缓存架构
命中 未命中 未命中 业务层 本地缓存 Redis集群 数据库
实现要点
- 使用 Caffeine 作为一级缓存
- Redis 作为二级缓存
- 自定义 CacheManager 实现分级策略
4.2 监控与治理
Spring Boot Actuator 集成
yaml
management:
endpoints:
web:
exposure:
include: caches,health,metrics
关键监控指标
cache.gets
:缓存查询次数cache.puts
:缓存写入次数cache.removals
:缓存清除次数cache.evictions
:缓存淘汰次数
五、最佳实践总结
5.1 配置推荐
yaml
# 生产环境推荐配置
spring:
cache:
type: redis
jitter-range: 30s
key-separator: "::"
redis:
lettuce:
pool:
max-active: 20
max-idle: 10
min-idle: 5
5.2 避坑指南
-
键设计原则
- 使用业务语义明确的键命名(如
user:profile:{userId}
) - 避免使用可变对象作为键
- 使用业务语义明确的键命名(如
-
缓存穿透防护
java@Cacheable(value = "users", unless = "#result == null") public User getUser(Long id) { // 返回null时自动跳过缓存 }
-
版本兼容策略
java@CachePut(value = "products#3600", key = "#product.id") public Product updateProduct(Product product) { // 更新后自动刷新缓存 }
最后
根据业务场景灵活选择适合的缓存策略,从简单的内存缓存到复杂的分布式缓存体系,Spring Boot 的缓存抽象层始终提供一致的使用体验。记住:没有完美的缓存方案,只有最适合业务场景的缓存策略。