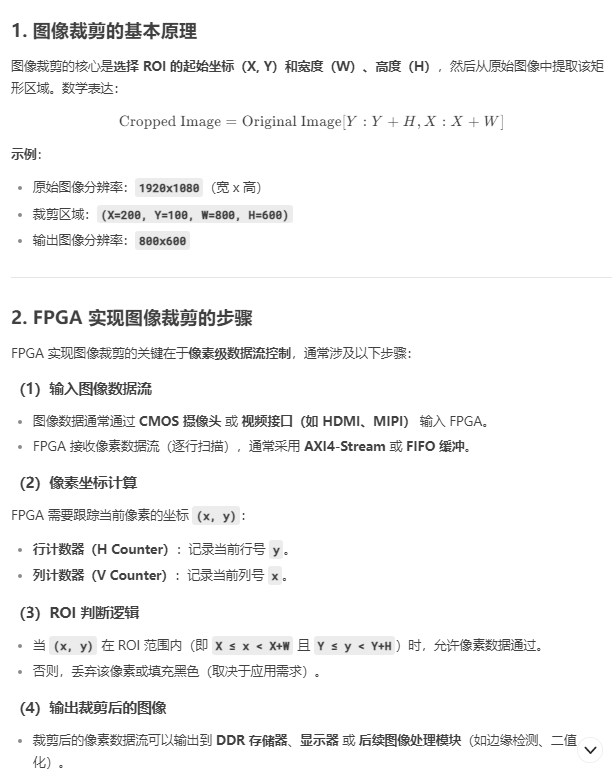
`timescale 1ns / 1ps
//
// Description: 图像裁剪算法
//
module image_crop(
input wire clk,
input wire reset,
input wire [10:0] img_width,
input wire [10:0] img_height,
input wire [10:0] img_x_start,
input wire [10:0] img_x_end,
input wire [10:0] img_y_start,
input wire [10:0] img_y_end,
input wire valid_i,
input wire [23:0] img_data_i,
output reg valid_o,
output reg [23:0] img_data_o
);
//参数声明
reg [10:0] x_cnt_r; //行计数器
reg [10:0] y_cnt_r; //列计数器
//计数
always@(posedge clk or posedge reset) begin
if(reset) begin
x_cnt_r <= 'b0;
y_cnt_r <= 'b0;
end else begin
x_cnt_r <= valid_i ? ((x_cnt_r == img_width - 1) ? 0 : x_cnt_r + 1) : x_cnt_r;
y_cnt_r <= valid_i&&(x_cnt_r == img_width - 1) ? ((y_cnt_r == img_height - 1) ? 0 : y_cnt_r + 1) : y_cnt_r;
end
end
always@(posedge clk or posedge reset) begin
if(reset) begin
valid_o <= 'b0;
img_data_o <= 'b0;
end else begin
valid_o <= valid_i&&(x_cnt_r >= img_x_start)&&(x_cnt_r < img_x_end)&&(y_cnt_r >= img_y_start)&&(y_cnt_r < img_y_end) ? 1'b1 : 1'b0;
img_data_o <= img_data_i;
end
end
endmodule
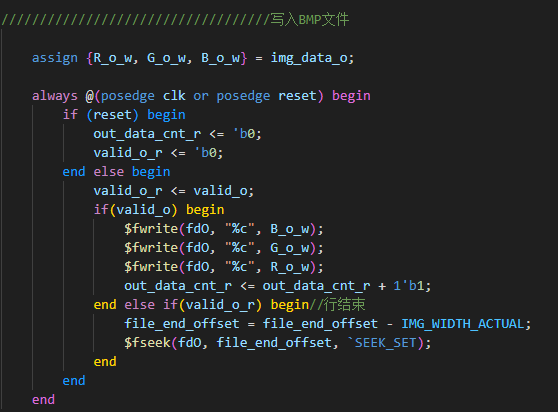