Ajax请求
目录
1.ajax请求使用
2.增加任务列表功能(只有查看和新增)
3.代码展示集合
这篇文章, 要开始讲关于ajax请求的内容了。这个和以前文章中写道的Vue框架里面的axios请求, 很相似。后端代码, 会有一些细节点, 跟前几节文章写的有些区别。
一、ajax请求使用
我们先在templates文件夹下面新建ajax_data文件夹, 然后再创建ajax_data.html文件。
我们先讲ajax使用的方法:
js
$.ajax({
//请求的目标地址
url: "/demo/one/",
// 请求类型
type:"post",
// 表单数据
data:{
sign: "45z6x4c65qf4c4c5z",
type: "text"
},
//请求成功后,接受目标函数的数据
success:function (res){
// 这里面需要写请求成功之后, 需要做的事情
console.log(res);
}
})
说完ajax请求后, 我们需要写关于ajax使用的代码:
编辑ajax_data.html:
html
{% extends "index/model_tmp.html" %}
{% block content %}
<div class="container">
<h1>Ajax演示-one</h1>
<input type="button" id="button-one" class="btn btn-success" value="点我">
<table border="1" class="table">
<thead>
<th>分类</th>
<th>名称</th>
<th>最高价</th>
<th>最低价</th>
<th>平均价</th>
<th>产地</th>
</thead>
<tbody id="tBody"></tbody>
</table>
<h1>Ajax演示-two</h1>
<input type="text" id="username" placeholder="请输入账号">
<input type="password" id="password" placeholder="请输入密码">
<input type="button" id="button-two" class="btn btn-success" value="点我">
</div>
{% endblock %}
{% block js %}
<script>
$(function () {
bindBtnOne();
bindBtnTwo();
})
function bindBtnOne() {
$("#button-one").click(function () {
$.ajax({
//请求的目标地址
url: "/demo/one/",
// 请求类型
type:"post",
// 表单数据
data:{
sign: "45z6x4c65qf4c4c5z",
type: "text"
},
//请求成功后,接受目标函数的数据
success:function (res){
//获取dict_data当中list所对于的值
var list = res.list;
var htmlStr = "";
for (var i=0; i<list.length;i++){
// emp相当于dict_data[list]当中的每一个字典
var emp = list[i];
//每次循环的时候,向页面添加一行六列
htmlStr += "<tr>";
htmlStr += "<td>"+emp.prodCat+"</td>";
htmlStr += "<td>"+emp.prodName+"</td>";
htmlStr += "<td>"+emp.highPrice+"</td>";
htmlStr += "<td>"+emp.lowPrice+"</td>";
htmlStr += "<td>"+emp.avgPrice+"</td>";
htmlStr += "<td>"+emp.place+"</td>";
htmlStr += "</tr>";
document.getElementById("tBody").innerHTML = htmlStr;
}
}
})
})
}
function bindBtnTwo() {
$("#button-two").click(function () {
$.ajax({
url:"/demo/two/",
type:"post",
data:{
username:$("#username").val(),
password:$("#password").val(),
},
success:function (res){
//alert(res)
alert(res.status)
}
})
})
}
</script>
{% endblock %}
这里面有两个ajax请求, 第一个是通过点击按钮来获取后端数据完成ajax请求, 最后我们把数据放在tbody标签里面显示, 第二个是通过判断账号密码两个输入框里面的内容, 是否包含在账号数据表里面, 如果有, 那就返回true, 否则就返回false。后端代码的逻辑, 会在后面写道。
我们在views里面创建Ajax_data.py:
Ajax_data.py代码:
python
from django.http import JsonResponse
from django.shortcuts import render, redirect
from django.views.decorators.csrf import csrf_exempt
# Create your views here.
def demo_list(request):
return render(request, "ajax_data/ajax_data.html")
@csrf_exempt
def demo_one(request):
dict_data = {
"current": 1,
"limit": 20,
"count": 129705,
"list": [
{
"id": 1724017,
"prodName": "大白菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "0.4",
"highPrice": "0.55",
"avgPrice": "0.48",
"place": "冀鲁豫鄂",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724016,
"prodName": "娃娃菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "0.65",
"highPrice": "0.75",
"avgPrice": "0.7",
"place": "豫",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724015,
"prodName": "小白菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.0",
"highPrice": "1.2",
"avgPrice": "1.1",
"place": "冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724014,
"prodName": "圆白菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "0.55",
"highPrice": "1.4",
"avgPrice": "0.98",
"place": "冀鄂鲁",
"specInfo": "甘蓝",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724013,
"prodName": "紫甘蓝",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.3",
"highPrice": "2.4",
"avgPrice": "2.35",
"place": "冀苏",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724012,
"prodName": "芹菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.2",
"highPrice": "1.4",
"avgPrice": "1.3",
"place": "鲁",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724011,
"prodName": "西芹",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.6",
"highPrice": "2.0",
"avgPrice": "1.8",
"place": "鲁辽",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724010,
"prodName": "菠菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.2",
"highPrice": "1.5",
"avgPrice": "1.35",
"place": "鲁冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724009,
"prodName": "莴笋",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.5",
"highPrice": "1.9",
"avgPrice": "1.7",
"place": "鲁",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724008,
"prodName": "团生菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.5",
"highPrice": "3.5",
"avgPrice": "3.0",
"place": "云冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724007,
"prodName": "罗马生菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "3.0",
"highPrice": "3.3",
"avgPrice": "3.15",
"place": "云",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724006,
"prodName": "散叶生菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.5",
"avgPrice": "2.25",
"place": "辽冀京",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724005,
"prodName": "油菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.2",
"highPrice": "1.5",
"avgPrice": "1.35",
"place": "皖川京鲁",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724004,
"prodName": "香菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.5",
"avgPrice": "2.25",
"place": "冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724003,
"prodName": "茴香",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.5",
"avgPrice": "2.25",
"place": "冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724002,
"prodName": "韭菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.8",
"avgPrice": "2.4",
"place": "冀粤",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724001,
"prodName": "苦菊",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.6",
"highPrice": "2.2",
"avgPrice": "1.9",
"place": "辽冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724000,
"prodName": "油麦菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.2",
"highPrice": "3.0",
"avgPrice": "2.6",
"place": "京冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1723999,
"prodName": "散菜花",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "3.8",
"avgPrice": "2.9",
"place": "陕云豫",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1723998,
"prodName": "绿菜花",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.3",
"highPrice": "2.8",
"avgPrice": "2.55",
"place": "苏鄂浙",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
}
]
}
return JsonResponse(dict_data)
@csrf_exempt
def demo_two(request):
dict_data = {
"status": True
}
return JsonResponse(dict_data)
demo_one函数里面的dict_data里面的数据, 就是当前端点击按钮的时候, 就会去向后端发起请求, 请求成功以后, 将数据返回给前端, 并展示到前端的页面上。
demo_two函数里面的dict_data里面的数据, 就是把status里面的布尔值传递给前端, 这个布尔值就代表判断账号密码的是否在账户数据库表格里面存在的意思, 这个逻辑, 我们会在下一篇文章, 具体写关于账号密码判断的逻辑。
还有, 这里面用了@csrf_exempt, 是因为在前端, 没有写form表单, 之前我们写前端的时候, 都是有form表单, 然后在里面写上post方法, 里面有csrf_token, 但是这里不一样, 用到了ajax请求, 里面type也是post, 所以我们没有在html里面写csrf_token, 而是把这个验证, 写到了后端代码里面。这就是和之前写的代码, 有小小的区别, 但代码的意思都是差不多的。在用@csrf_exempt,之前, 需要导入包:from django.views.decorators.csrf import csrf_exempt。
这里面有个叫JsonResponse, 意思就是把传进去的参数, 以json格式返回。之后前端请求到的数据, 就是json格式的数据了。
二、 增加任务列表功能(只有查看和新增)
首先, 我们需要先增加任务数据库表格, 这个是毋庸置疑的。
python
class Task(models.Model):
title = models.CharField(verbose_name="标题", max_length=64)
level_choice = {
(1, "临时任务"),
(2, "普通任务"),
(3, "重要任务"),
(4, "紧急任务")
}
level = models.SmallIntegerField(verbose_name="任务级别", choices=level_choice)
detail = models.TextField(verbose_name="任务内容")
user = models.ForeignKey(verbose_name="负责人", to="UserInfo", on_delete=models.CASCADE, null=True, blank=True)
times = models.DateField(verbose_name="开始时间")
code_choices = {
(1, "未完成"),
(2, "正在处理"),
(3, "已完成")
}
code = models.SmallIntegerField(verbose_name="任务状态", choices=code_choices)
然后在项目对应的目录下, 执行python manage.py makemigrations
和python manage.py migrate
这两行代码。
接着, 我们在templates里面创建task_data.html文件。
task_data.html:
html
{% extends "index/model_tmp.html" %}
{% block content %}
<div class="container">
<div class="panel panel-success">
<div class="panel-heading">
<h3 class="panel-title">添加任务</h3>
</div>
<div class="panel-body">
<div class="form-group">
<form id="formAdd">
{% for filed in form %}
<div class="col-xs-6">
<label for="exampleInputEmail1">{{ filed.label }}</label>
{{ filed }}
</div>
{% endfor %}
<div class="col-xs-12">
<button type="button" id="button-add" class="btn btn-success">提交</button>
</div>
</form>
</div>
</div>
</div>
</div>
<div class="container">
<div class="panel panel-success">
<div class="panel-heading">
<h3 class="panel-title">任务展示</h3>
</div>
<div class="panel-body">
<table class="table table-bordered">
<thead>
<tr>
<th>任务ID</th>
<th>任务标题</th>
<th>任务级别</th>
<th>任务内容</th>
<th>任务时间</th>
<th>任务状态</th>
<th>任务负责人</th>
<th>操作</th>
</tr>
</thead>
<tbody>
{% for data in queryset %}
<tr>
<th>{{ data.id }}</th>
<th>{{ data.title }}</th>
<th>{{ data.get_level_display }}</th>
<th>{{ data.detail }}</th>
<th>{{ data.times }}</th>
<th>{{ data.get_code_display }}</th>
<th>{{ data.user.name }}</th>
</tr>
{% endfor %}
</tbody>
</table>
</div>
</div>
</div>
{% endblock %}
{% block js %}
<script>
$(function (){
bindBtnEvent();
})
function bindBtnEvent() {
$("#button-add").click(function () {
$.ajax({
url :"/task/add/",
type:"post",
data:$("#formAdd").serialize(),
dataType:"JSON",
success:function (res){
alert("添加成功")
}
})
})
}
</script>
{% endblock %}
我们这里把添加和展示页面, 都写在同一个页面上面了。
在views里面新建一个task_data.py:
task_data.py代码:
python
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
from django.shortcuts import render
from project_one import models
from django import forms
# Create your views here.
class taskModelForm(forms.ModelForm):
class Meta:
model = models.Task
fields = '__all__'
def __init__(self, *args, **kwargs):
super(taskModelForm, self).__init__(*args, **kwargs)
for item, field in self.fields.items():
field.widget.attrs['class'] = 'form-control'
field.widget.attrs['autocomplete'] = 'off'
@csrf_exempt
def task_list(request):
queryset = models.Task.objects.all()
form = taskModelForm()
content = {'form': form, "queryset": queryset}
return render(request, "task_data/task_list.html", content)
@csrf_exempt
def task_add(request):
form = taskModelForm(request.POST)
if form.is_valid():
form.save()
dict_data = {"status": True}
return JsonResponse(dict_data)
dict_data = {"status": False}
return JsonResponse(dict_data)
task_list是展示任务的内容, task_add是添加任务的内容。
在task_add里面, dict_data的status的值, 是根据数据是否添加成功来决定的, 当数据添加成功的时候, dict_data的status的值为true, 反之就为false。
最后配置下路由:
python
"""project_simple URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/4.1/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: path('', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: path('', Home.as_view(), name='home')
Including another URLconf
1. Import the include() function: from django.urls import include, path
2. Add a URL to urlpatterns: path('blog/', include('blog.urls'))
"""
from django.contrib import admin
from django.urls import path
from project_one.views import depart, user, assets, admin_role, login, Ajax_data, task_data
urlpatterns = [
path("task/list/", task_data.task_list, name="task_list"),
path("task/add/", task_data.task_add, name="task_add"),
]
我们不要忘记在model_tmp.html里面添加ajax请求和任务列表:
model_tmp.html:
html
<li class="dropdown">
{% if request.unicom_role == 3 %}
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true"
aria-expanded="false">平台用户<span class="caret"></span></a>
{% endif %}
<ul class="dropdown-menu">
<li><a href="/admin_list/">登录账号</a></li>
<li><a href="/demo/list/">ajax请求</a></li>
</ul>
</li>
<li class="active"><a href="/task/list/">任务列表</a></li>
运行结果:
点击平台用户里面的ajax请求:
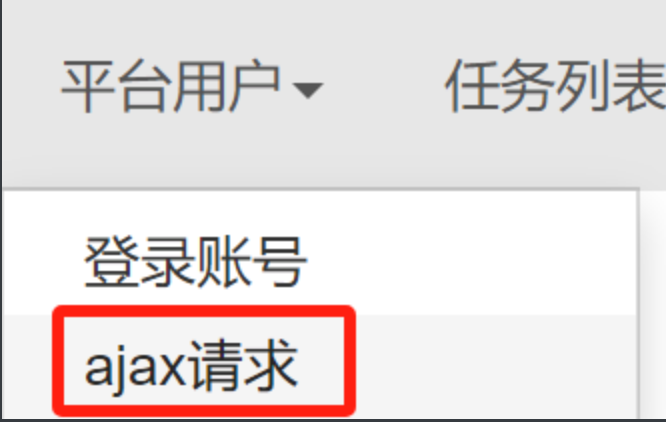
点击按钮:
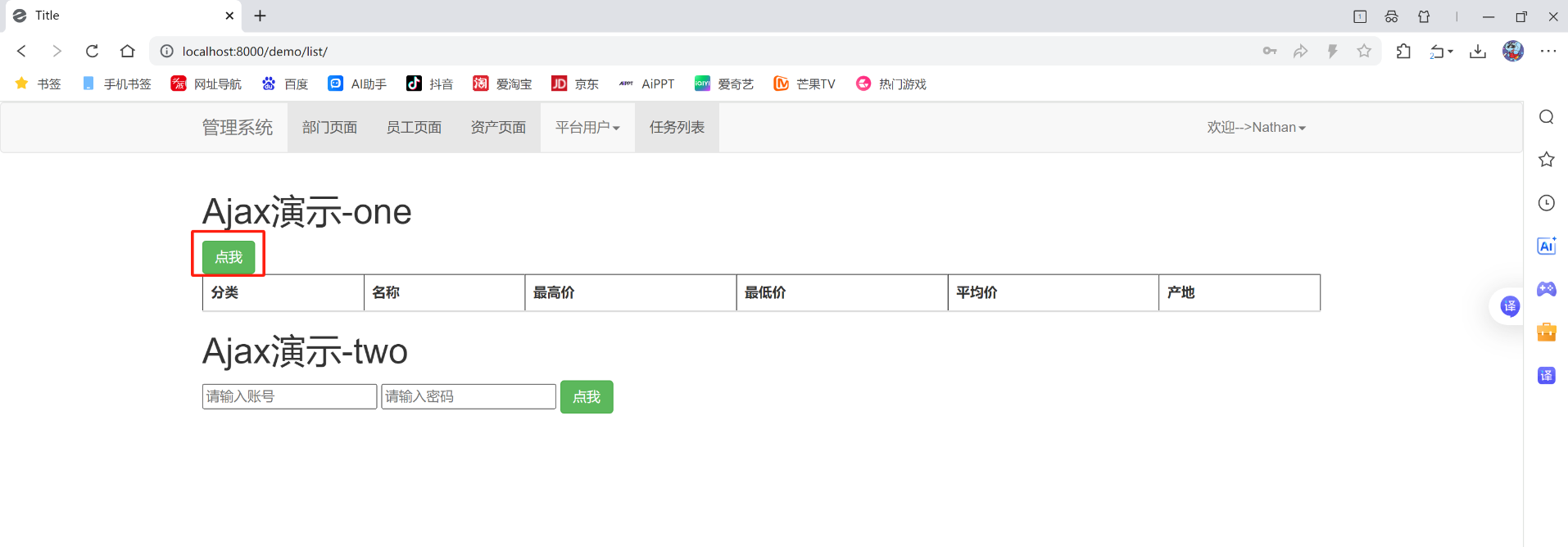
请求成功:
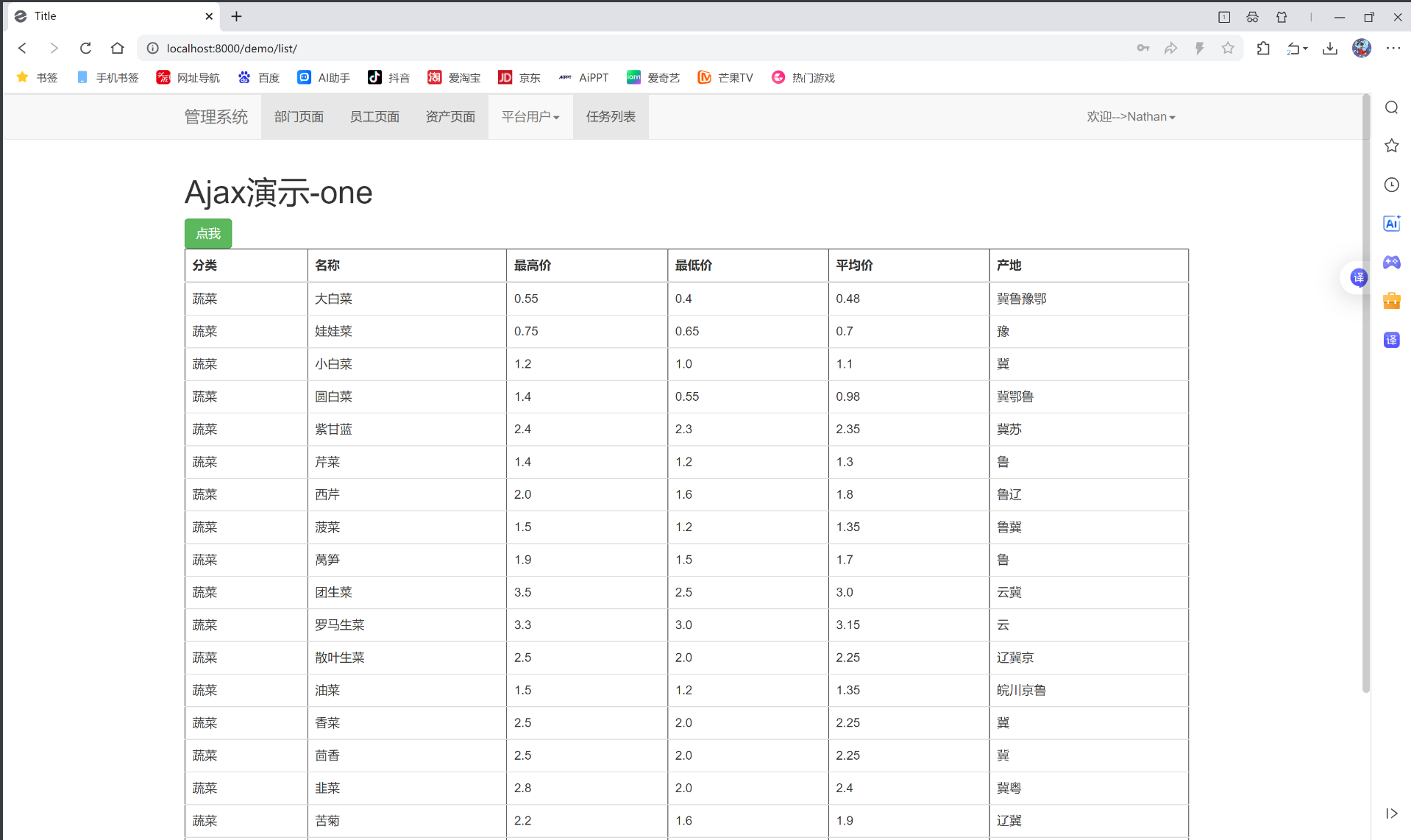
然后在下面的输入框里面随便输入内容:

点击按钮:
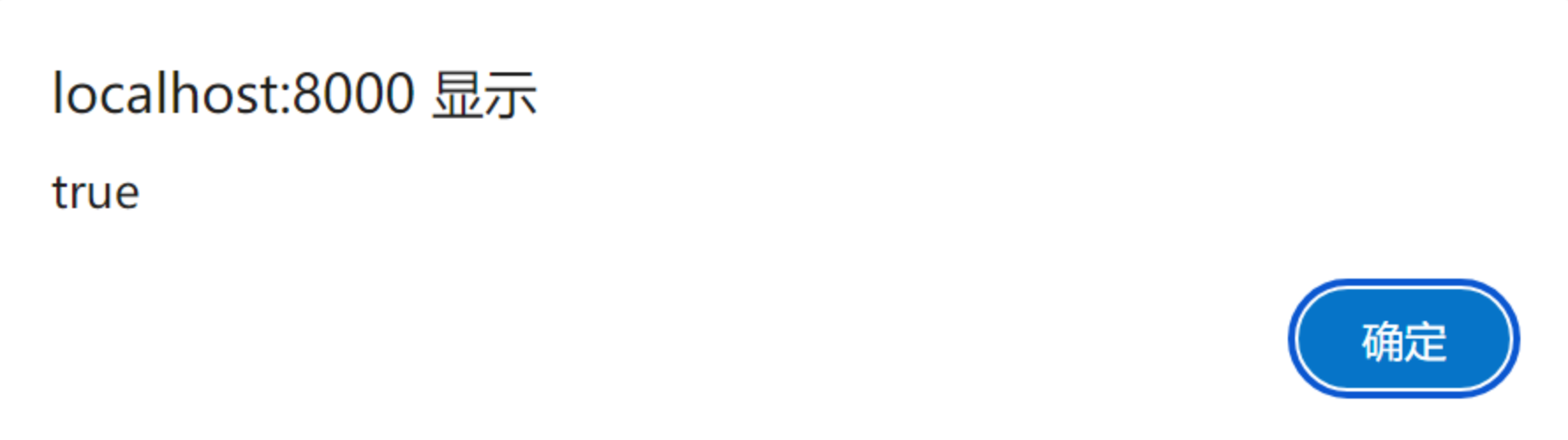
由于我们在后端代码里面没有写到判断账号密码的逻辑, 所以它这里一直返回的是true, 这个问题, 会在下一篇文章, 里面写道。
接着再点击任务列表:
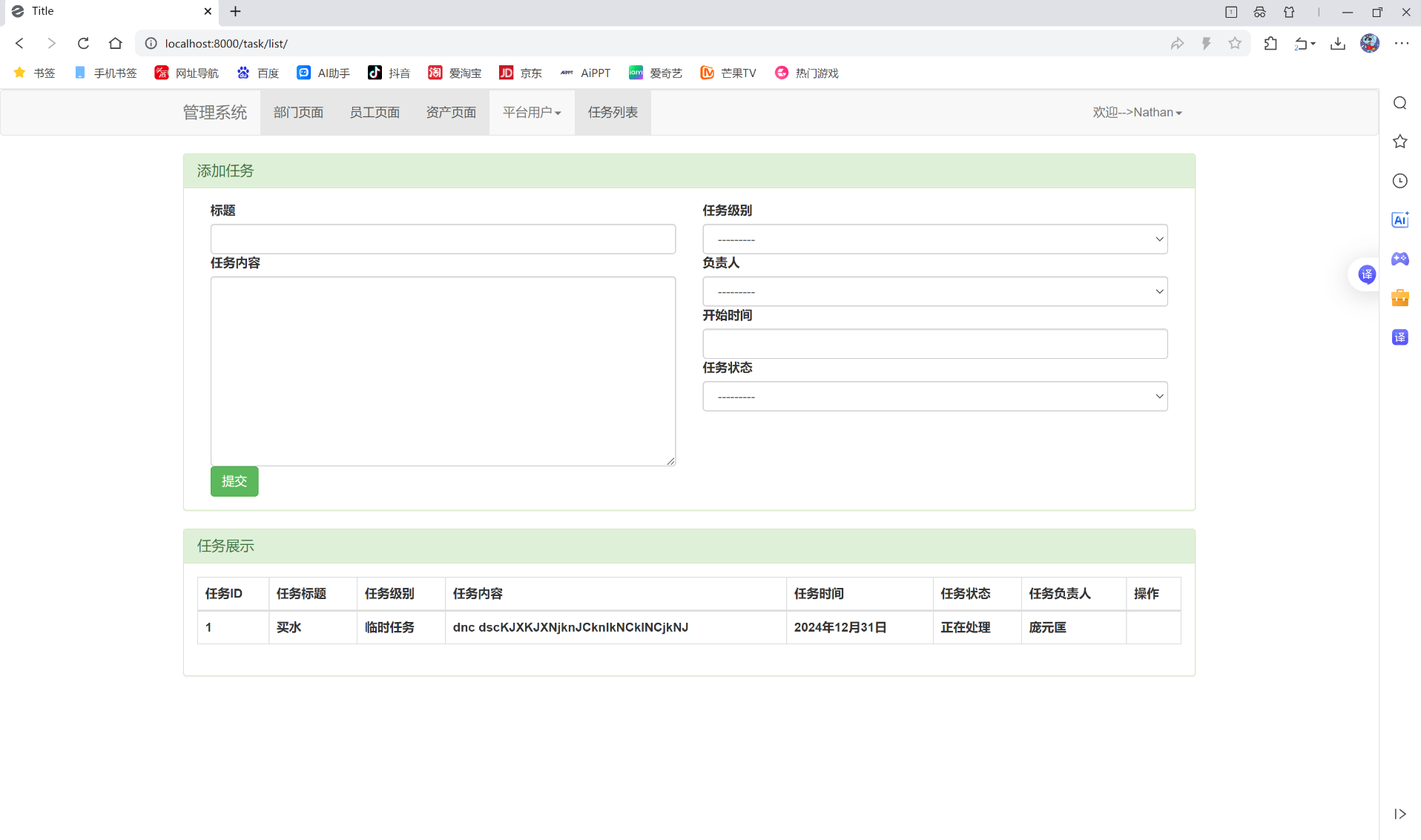
上面填写内容并提交:
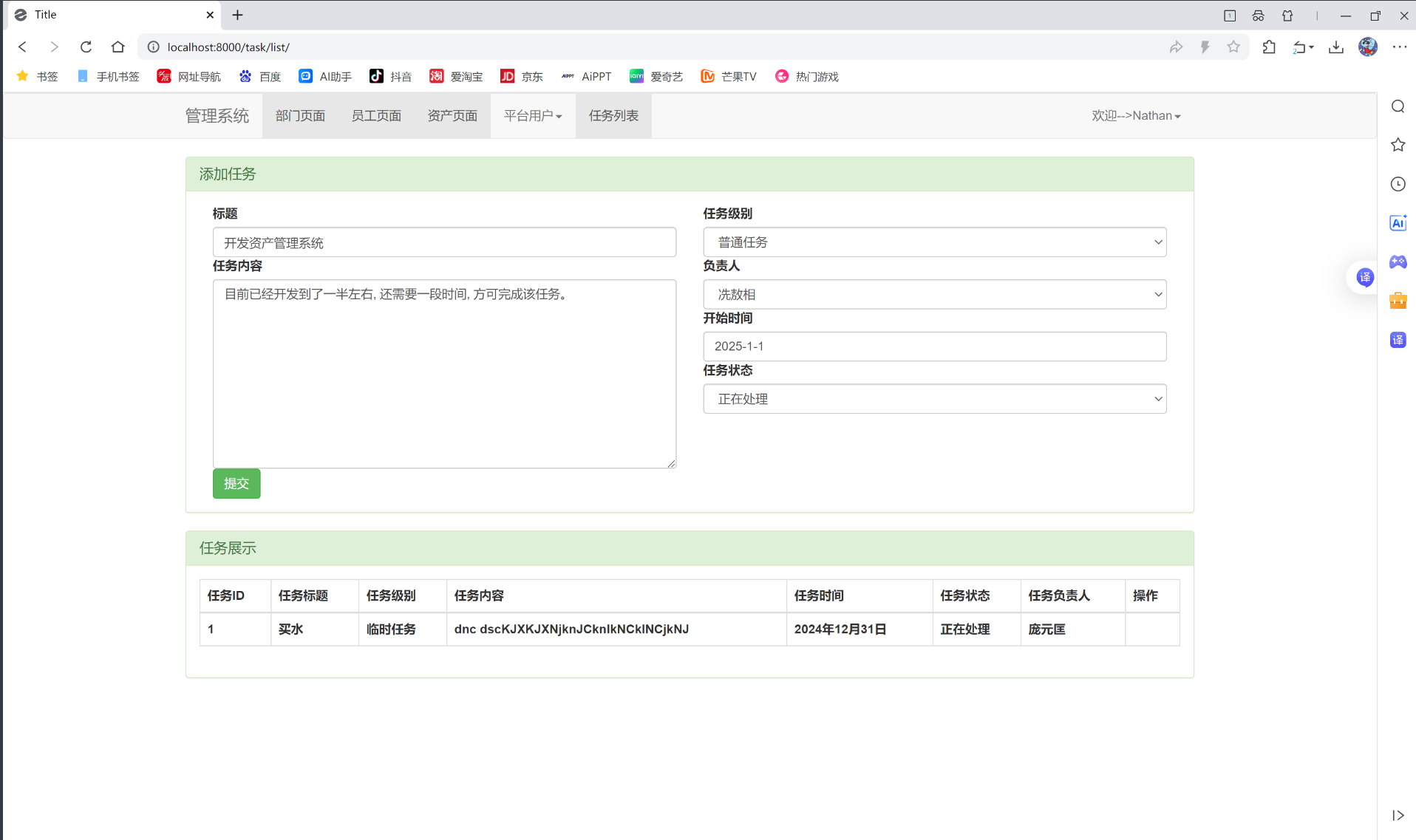
弹窗, 显示添加成功:
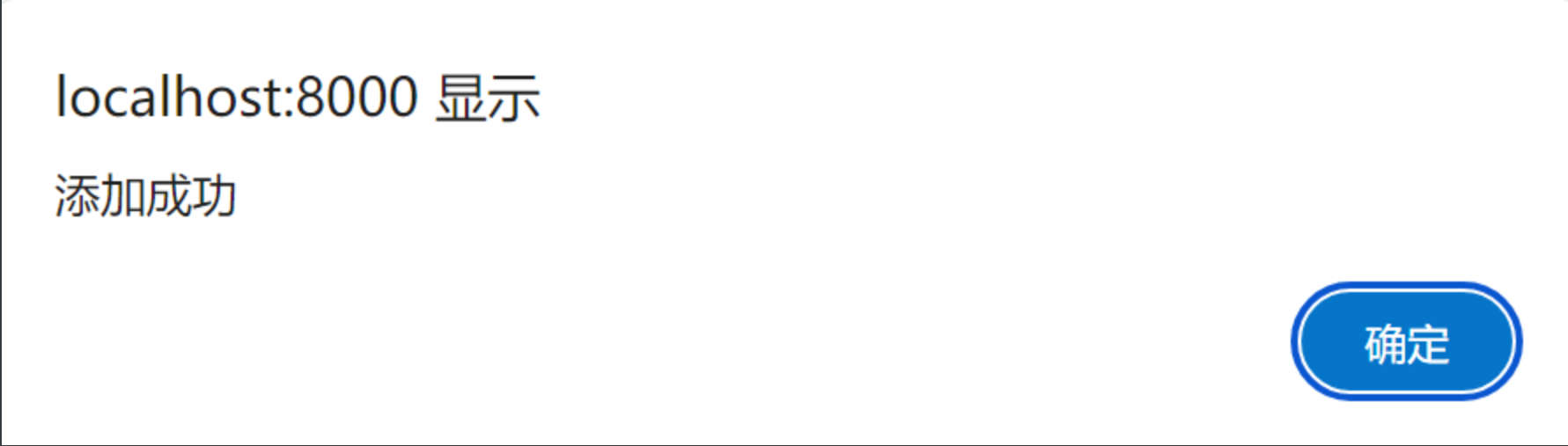
然后再刷新下网页:
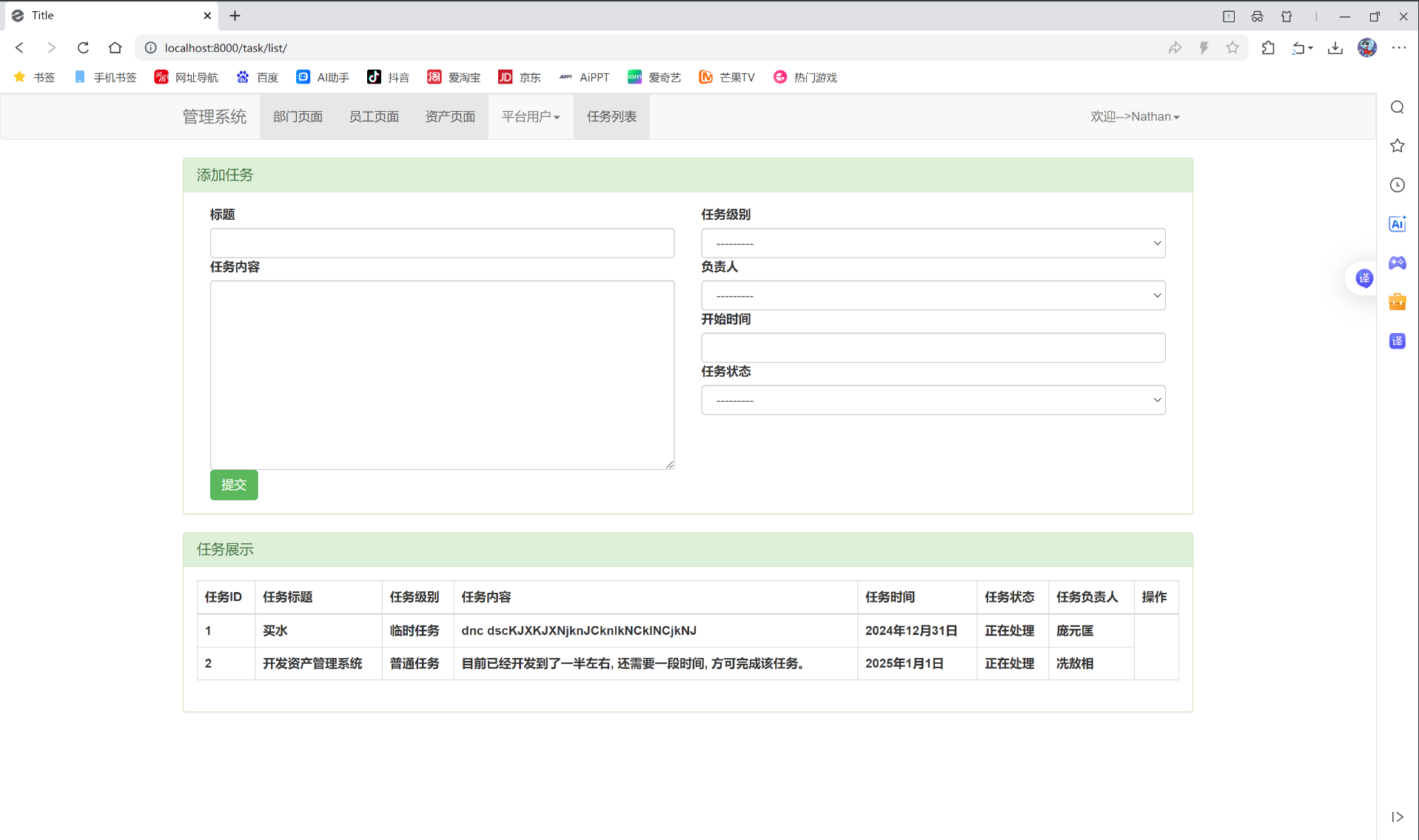
我们发现, 数据添加成功。
三、代码展示集合
前端:
ajax_data.html:
html
{% extends "index/model_tmp.html" %}
{% block content %}
<div class="container">
<h1>Ajax演示-one</h1>
<input type="button" id="button-one" class="btn btn-success" value="点我">
<table border="1" class="table">
<thead>
<th>分类</th>
<th>名称</th>
<th>最高价</th>
<th>最低价</th>
<th>平均价</th>
<th>产地</th>
</thead>
<tbody id="tBody"></tbody>
</table>
<h1>Ajax演示-two</h1>
<input type="text" id="username" placeholder="请输入账号">
<input type="password" id="password" placeholder="请输入密码">
<input type="button" id="button-two" class="btn btn-success" value="点我">
</div>
{% endblock %}
{% block js %}
<script>
$(function () {
bindBtnOne();
bindBtnTwo();
})
function bindBtnOne() {
$("#button-one").click(function () {
$.ajax({
//请求的目标地址
url: "/demo/one/",
// 请求类型
type:"post",
// 表单数据
data:{
sign: "45z6x4c65qf4c4c5z",
type: "text"
},
//请求成功后,接受目标函数的数据
success:function (res){
//获取dict_data当中list所对于的值
var list = res.list;
var htmlStr = "";
for (var i=0; i<list.length;i++){
// emp相当于dict_data[list]当中的每一个字典
var emp = list[i];
//每次循环的时候,向页面添加一行六列
htmlStr += "<tr>";
htmlStr += "<td>"+emp.prodCat+"</td>";
htmlStr += "<td>"+emp.prodName+"</td>";
htmlStr += "<td>"+emp.highPrice+"</td>";
htmlStr += "<td>"+emp.lowPrice+"</td>";
htmlStr += "<td>"+emp.avgPrice+"</td>";
htmlStr += "<td>"+emp.place+"</td>";
htmlStr += "</tr>";
document.getElementById("tBody").innerHTML = htmlStr;
}
}
})
})
}
function bindBtnTwo() {
$("#button-two").click(function () {
$.ajax({
url:"/demo/two/",
type:"post",
data:{
username:$("#username").val(),
password:$("#password").val(),
},
success:function (res){
//alert(res)
alert(res.status)
}
})
})
}
</script>
{% endblock %}
task_data.html:
html
{% extends "index/model_tmp.html" %}
{% block content %}
<div class="container">
<div class="panel panel-success">
<div class="panel-heading">
<h3 class="panel-title">添加任务</h3>
</div>
<div class="panel-body">
<div class="form-group">
<form id="formAdd">
{% for filed in form %}
<div class="col-xs-6">
<label for="exampleInputEmail1">{{ filed.label }}</label>
{{ filed }}
</div>
{% endfor %}
<div class="col-xs-12">
<button type="button" id="button-add" class="btn btn-success">提交</button>
</div>
</form>
</div>
</div>
</div>
</div>
<div class="container">
<div class="panel panel-success">
<div class="panel-heading">
<h3 class="panel-title">任务展示</h3>
</div>
<div class="panel-body">
<table class="table table-bordered">
<thead>
<tr>
<th>任务ID</th>
<th>任务标题</th>
<th>任务级别</th>
<th>任务内容</th>
<th>任务时间</th>
<th>任务状态</th>
<th>任务负责人</th>
<th>操作</th>
</tr>
</thead>
<tbody>
{% for data in queryset %}
<tr>
<th>{{ data.id }}</th>
<th>{{ data.title }}</th>
<th>{{ data.get_level_display }}</th>
<th>{{ data.detail }}</th>
<th>{{ data.times }}</th>
<th>{{ data.get_code_display }}</th>
<th>{{ data.user.name }}</th>
</tr>
{% endfor %}
</tbody>
</table>
</div>
</div>
</div>
{% endblock %}
{% block js %}
<script>
$(function (){
bindBtnEvent();
})
function bindBtnEvent() {
$("#button-add").click(function () {
$.ajax({
url :"/task/add/",
type:"post",
data:$("#formAdd").serialize(),
dataType:"JSON",
success:function (res){
alert("添加成功")
}
})
})
}
</script>
{% endblock %}
model_tmp.html:
html
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="{% static 'css/bootstrap.css' %}">
{% block css %}
{% endblock %}
</head>
<body>
<div class="navbar navbar-default">
<div class="container">
<!-- Brand and toggle get grouped for better mobile display -->
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse"
data-target="#bs-example-navbar-collapse-1" aria-expanded="false">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="#">管理系统</a>
</div>
<!-- Collect the nav links, forms, and other content for toggling -->
<div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1">
<ul class="nav navbar-nav">
{% if request.unicom_role == 1 %}
<li class="active"><a href="/depart/">部门页面</a></li>
<li class="active"><a href="/user/">员工页面</a></li>
{% elif request.unicom_role == 3%}
<li class="active"><a href="/depart/">部门页面</a></li>
<li class="active"><a href="/user/">员工页面</a></li>
<li class="active"><a href="/assets_list/">资产页面</a></li>
{% endif %}
{# <li class="active"><a href="/depart/">部门页面</a></li>#}
{# <li class="active"><a href="/user/">员工页面</a></li>#}
{# <li class="active"><a href="/assets_list/">资产页面</a></li>#}
<li class="dropdown">
{% if request.unicom_role == 3 %}
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true"
aria-expanded="false">平台用户<span class="caret"></span></a>
{% endif %}
<ul class="dropdown-menu">
<li><a href="/admin_list/">登录账号</a></li>
<li><a href="/demo/list/">ajax请求</a></li>
</ul>
</li>
<li class="active"><a href="/task/list/">任务列表</a></li>
</ul>
<ul class="nav navbar-nav navbar-right">
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-haspopup="true"
aria-expanded="false">欢迎-->{{ request.session.info.username }}<span class="caret"></span></a>
<ul class="dropdown-menu">
<li><a href="/logout/">退出登录</a></li>
</ul>
</li>
</ul>
</div><!-- /.navbar-collapse -->
</div><!-- /.container-fluid -->
</div>
{% block content %}
{% endblock %}
<script src="{% static 'js/jquery3.7.1.js' %}"></script>
<script src="{% static 'js/bootstrap.js' %}"></script>
{% block js %}
{% endblock %}
</body>
</html>
后端:
python
from django.db import models
# Create your models here.
class Department(models.Model):
title = models.CharField(verbose_name="部门名称", max_length=255)
def __str__(self):
return self.title
class UserInfo(models.Model):
name = models.CharField(verbose_name="姓名", max_length=255)
gender_choices = (
(1, "男"), (2, "女")
)
gender = models.SmallIntegerField(verbose_name="性别", choices=gender_choices)
salary = models.IntegerField(verbose_name="薪水")
age = models.IntegerField(verbose_name="年龄")
create_time = models.DateField(verbose_name="入职时间")
department = models.ForeignKey(verbose_name="部门", max_length=255, to="Department", to_field="id",
on_delete=models.CASCADE, null=True, blank=True)
def __str__(self):
return self.name
class Assets(models.Model):
mobile = models.CharField(verbose_name="手机号", max_length=11)
status_code_choice = (
(1, "已使用"),
(2, "未使用")
)
status = models.SmallIntegerField(verbose_name="状态", choices=status_code_choice)
create_time = models.DateField(verbose_name="创建时间")
user = models.ForeignKey(to="UserInfo", to_field="id", verbose_name="使用者", on_delete=models.SET_NULL, null=True, blank=True)
price = models.CharField(verbose_name="价格", max_length=10)
class AdminRole(models.Model):
username = models.CharField(verbose_name="用户名", max_length=32)
password = models.CharField(verbose_name="密码", max_length=64)
password_choice = (
(1, "员工"),
(2, "领导"),
(3, "管理员")
)
role = models.SmallIntegerField(verbose_name="角色", choices=password_choice)
class Task(models.Model):
title = models.CharField(verbose_name="标题", max_length=64)
level_choice = {
(1, "临时任务"),
(2, "普通任务"),
(3, "重要任务"),
(4, "紧急任务")
}
level = models.SmallIntegerField(verbose_name="任务级别", choices=level_choice)
detail = models.TextField(verbose_name="任务内容")
user = models.ForeignKey(verbose_name="负责人", to="UserInfo", on_delete=models.CASCADE, null=True, blank=True)
times = models.DateField(verbose_name="开始时间")
code_choices = {
(1, "未完成"),
(2, "正在处理"),
(3, "已完成")
}
code = models.SmallIntegerField(verbose_name="任务状态", choices=code_choices)
Ajax_data.py:
python
from django.http import JsonResponse
from django.shortcuts import render, redirect
from django.views.decorators.csrf import csrf_exempt
# Create your views here.
def demo_list(request):
return render(request, "ajax_data/ajax_data.html")
@csrf_exempt
def demo_one(request):
dict_data = {
"current": 1,
"limit": 20,
"count": 129705,
"list": [
{
"id": 1724017,
"prodName": "大白菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "0.4",
"highPrice": "0.55",
"avgPrice": "0.48",
"place": "冀鲁豫鄂",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724016,
"prodName": "娃娃菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "0.65",
"highPrice": "0.75",
"avgPrice": "0.7",
"place": "豫",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724015,
"prodName": "小白菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.0",
"highPrice": "1.2",
"avgPrice": "1.1",
"place": "冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724014,
"prodName": "圆白菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "0.55",
"highPrice": "1.4",
"avgPrice": "0.98",
"place": "冀鄂鲁",
"specInfo": "甘蓝",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724013,
"prodName": "紫甘蓝",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.3",
"highPrice": "2.4",
"avgPrice": "2.35",
"place": "冀苏",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724012,
"prodName": "芹菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.2",
"highPrice": "1.4",
"avgPrice": "1.3",
"place": "鲁",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724011,
"prodName": "西芹",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.6",
"highPrice": "2.0",
"avgPrice": "1.8",
"place": "鲁辽",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724010,
"prodName": "菠菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.2",
"highPrice": "1.5",
"avgPrice": "1.35",
"place": "鲁冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724009,
"prodName": "莴笋",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.5",
"highPrice": "1.9",
"avgPrice": "1.7",
"place": "鲁",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724008,
"prodName": "团生菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.5",
"highPrice": "3.5",
"avgPrice": "3.0",
"place": "云冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724007,
"prodName": "罗马生菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "3.0",
"highPrice": "3.3",
"avgPrice": "3.15",
"place": "云",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724006,
"prodName": "散叶生菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.5",
"avgPrice": "2.25",
"place": "辽冀京",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724005,
"prodName": "油菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.2",
"highPrice": "1.5",
"avgPrice": "1.35",
"place": "皖川京鲁",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724004,
"prodName": "香菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.5",
"avgPrice": "2.25",
"place": "冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724003,
"prodName": "茴香",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.5",
"avgPrice": "2.25",
"place": "冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724002,
"prodName": "韭菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "2.8",
"avgPrice": "2.4",
"place": "冀粤",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724001,
"prodName": "苦菊",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "1.6",
"highPrice": "2.2",
"avgPrice": "1.9",
"place": "辽冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1724000,
"prodName": "油麦菜",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.2",
"highPrice": "3.0",
"avgPrice": "2.6",
"place": "京冀",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1723999,
"prodName": "散菜花",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.0",
"highPrice": "3.8",
"avgPrice": "2.9",
"place": "陕云豫",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
},
{
"id": 1723998,
"prodName": "绿菜花",
"prodCatid": 1186,
"prodCat": "蔬菜",
"prodPcatid": "null",
"prodPcat": "",
"lowPrice": "2.3",
"highPrice": "2.8",
"avgPrice": "2.55",
"place": "苏鄂浙",
"specInfo": "",
"unitInfo": "斤",
"pubDate": "2024-12-31 00:00:00",
"status": "null",
"userIdCreate": 138,
"userIdModified": "null",
"userCreate": "admin",
"userModified": "null",
"gmtCreate": "null",
"gmtModified": "null"
}
]
}
return JsonResponse(dict_data)
@csrf_exempt
def demo_two(request):
dict_data = {
"status": True
}
return JsonResponse(dict_data)
task_data.py:
python
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
from django.shortcuts import render
from project_one import models
from django import forms
# Create your views here.
class taskModelForm(forms.ModelForm):
class Meta:
model = models.Task
fields = '__all__'
def __init__(self, *args, **kwargs):
super(taskModelForm, self).__init__(*args, **kwargs)
for item, field in self.fields.items():
field.widget.attrs['class'] = 'form-control'
field.widget.attrs['autocomplete'] = 'off'
@csrf_exempt
def task_list(request):
queryset = models.Task.objects.all()
form = taskModelForm()
content = {'form': form, "queryset": queryset}
return render(request, "task_data/task_list.html", content)
@csrf_exempt
def task_add(request):
form = taskModelForm(request.POST)
if form.is_valid():
form.save()
dict_data = {"status": True}
return JsonResponse(dict_data)
dict_data = {"status": False}
return JsonResponse(dict_data)
python
"""project_simple URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/4.1/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: path('', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: path('', Home.as_view(), name='home')
Including another URLconf
1. Import the include() function: from django.urls import include, path
2. Add a URL to urlpatterns: path('blog/', include('blog.urls'))
"""
from django.contrib import admin
from django.urls import path
from project_one.views import depart, user, assets, admin_role, login, Ajax_data, task_data
urlpatterns = [
# path('admin/', admin.site.urls),
path("", depart.index, name="index"),
path("depart/", depart.depart, name="depart"),
path("depart/add/", depart.add_depart, name="add_depart"),
path("depart/<int:nid>/modify/", depart.depart_modify, name="depart_modify"),
path("depart/<int:nid>/del/", depart.del_depart, name="del_depart"),
path("user/", user.user_info, name="user_info"),
path("user/add/", user.user_add, name="user_add"),
path("user/<int:nid>/modify/", user.user_modify, name="user_modify"),
path("user/<int:nid>/del/", user.user_del, name="user_del"),
path("user/add/modelform", user.user_add_modelform, name="user_add_modelform"),
path("user/<int:nid>/modify/modelform", user.user_modify_modelform, name="user_modify_modelform"),
path("assets_list/", assets.assets, name="assets"),
path("assets/add/", assets.assets_add, name="assets_add"),
path("assets/<int:nid>/modify/", assets.assets_modify, name="assets_modify"),
path("assets/<int:nid>/del/", assets.assets_del, name="assets_del"),
path("admin_list/", admin_role.admin, name="admin"),
path("admin/add/", admin_role.admin_add, name="admin_add"),
path("admin/<int:nid>/modify/", admin_role.admin_modify, name="admin_modify"),
path("admin/<int:nid>/reset/pwd/", admin_role.admin_reset_pwd, name="admin_reset_pwd"),
path("admin/<int:nid>/del/", admin_role.admin_del, name="admin_del"),
path("login/", login.login, name="login"),
path("logout/", login.logout, name="logout"),
path("image/code/", login.image_code, name="image_code"),
path("demo/list/", Ajax_data.demo_list, name="demo_list"),
path("demo/one/", Ajax_data.demo_one, name="demo_one"),
path("demo/two/", Ajax_data.demo_two, name="demo_two"),
path("task/list/", task_data.task_list, name="task_list"),
path("task/add/", task_data.task_add, name="task_add"),
]
好了, 关于django框架里面的ajax请求使用的内容, 就到此为止了。这个ajax使用, 和Vue框架里面的axios差不多, 还有一些后端代码, 有些写法和之前的不一样, 可以和前面文章写的后端代码进行对比学习!!!
以上就是Django框架里面的ajax请求使用的所有内容了, 如果有哪里不懂的地方,可以把问题打在评论区, 欢迎大家在评论区交流!!!
如果我有写错的地方, 望大家指正, 也可以联系我, 让我们一起努力, 继续不断的进步.
学习是个漫长的过程, 需要我们不断的去学习并掌握消化知识点, 有不懂或概念模糊不理解的情况下,一定要赶紧的解决问题, 否则问题只会越来越多, 漏洞也就越老越大.
人生路漫漫, 白鹭常相伴!!!