- 操作系统:ubuntu22.04
- OpenCV版本:OpenCV4.9
- IDE:Visual Studio Code
- 编程语言:C++11
算法描述
该函数用于创建一个 Scharr 滤波器(基于 CUDA 加速),用于图像的一阶导数计算。它常用于边缘检测任务中,相比 Sobel 滤波器具有更高的方向精度和更小的误差。
在 GPU 上使用此滤波器可显著提升图像处理速度,特别适合大规模图像或实时视频处理任务。
函数原型
cpp
Ptr<Filter> cv::cuda::createScharrFilter
(
int srcType,
int dstType,
int dx,
int dy,
double scale = 1,
int rowBorderMode = BORDER_DEFAULT,
int columnBorderMode = -1
)
参数
参数名 | 类型 | 描述 |
---|---|---|
srcType |
int |
输入图像类型。例如 CV_8UC1 , CV_32FC1 等。 |
dstType |
int |
输出图像类型。通常使用浮点类型如 CV_32FC1 。 |
dx |
int |
x 方向上的导数阶数,取值为 0 或 1。 |
dy |
int |
y 方向上的导数阶数,取值为 0 或 1,且必须满足 dx + dy == 1 。 |
scale |
double |
可选比例因子,默认为 1。用于对滤波结果进行缩放。 |
rowBorderMode |
int |
行方向滤波时使用的边界填充方式。常用如 BORDER_DEFAULT 、BORDER_REPLICATE 等。 |
columnBorderMode |
int |
列方向滤波时使用的边界填充方式。默认值 -1 表示与 rowBorderMode 相同。 |
代码示例
cpp
#include <opencv2/cudafilters.hpp>
#include <opencv2/cudaimgproc.hpp>
#include <opencv2/opencv.hpp>
int main()
{
// 读取图像并上传到 GPU
cv::Mat h_input = cv::imread( "/media/dingxin/data/study/OpenCV/sources/images/Lenna.png", cv::IMREAD_GRAYSCALE );
cv::cuda::GpuMat d_input, d_output;
d_input.upload( h_input );
// 创建 Scharr 滤波器(检测 x 方向边缘)
auto scharrX = cv::cuda::createScharrFilter( CV_8UC1, CV_32FC1, 1, 0 );
// 应用滤波器
scharrX->apply( d_input, d_output );
// 下载结果并显示
cv::Mat h_output;
d_output.download( h_output );
// 归一化显示
cv::convertScaleAbs( h_output, h_output ); // 转换回 uchar 类型
cv::imshow( "Scharr X Output", h_output );
cv::waitKey( 0 );
return 0;
}
运行结果
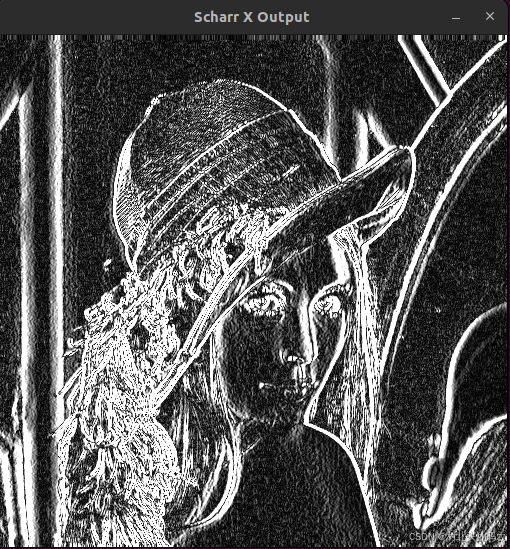