《博主简介》
小伙伴们好,我是阿旭。专注于人工智能、AIGC、python、计算机视觉相关分享研究。
✌更多学习资源,可关注公-仲-hao:【阿旭算法与机器学习】,共同学习交流~
👍感谢小伙伴们点赞、关注!
《------往期经典推荐------》
二、机器学习实战专栏【链接】 ,已更新31期,欢迎关注,持续更新中~~
三、深度学习【Pytorch】专栏【链接】
四、【Stable Diffusion绘画系列】专栏【链接】
五、YOLOv8改进专栏【链接】,持续更新中~~
六、YOLO性能对比专栏【链接】,持续更新中~
《------正文------》
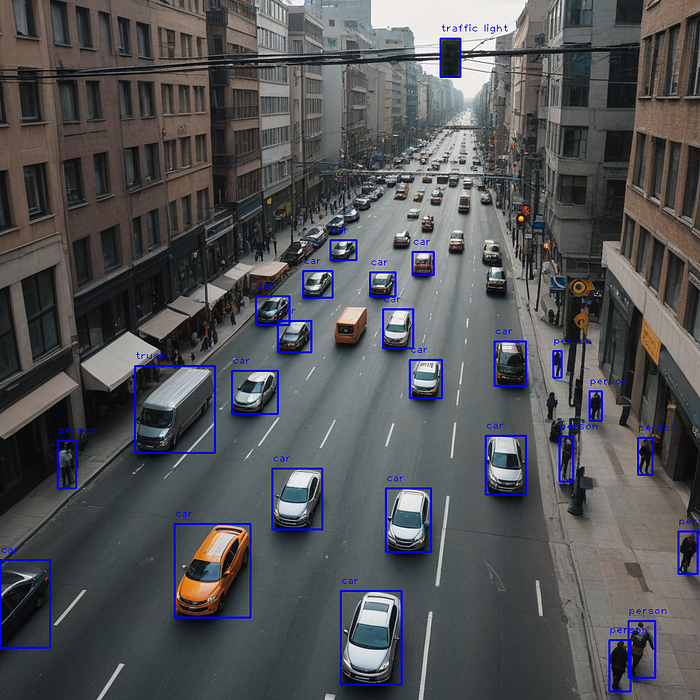
介绍
在之前的博客文章中,我们介绍了如何使用 YOLOv8 进行对象检测。这篇文章则主要介绍如何使用YOLOv9进行图像与视频检测
。
YOLOv9 与其前身一样,专注于识别和精确定位图像和视频中的对象。自动驾驶汽车、安全系统和高级图像搜索等应用在很大程度上依赖于此功能。YOLOv9 引入了比 YOLOv8 更令人印象深刻的创新点。
使用 YOLOv9 处理图像和视频
步骤 1:安装必要的库
pip install opencv-python ultralytics
第 2 步:导入库
python
import cv2
from ultralytics import YOLO
第 3 步:选择模型型号尺寸
model = YOLO("yolov9c.pt")
这里,我们选择了 yolov9c.pt。大家可以选择不同的模型尺寸进行检测,并比较不同的型号并权衡它们各自的优缺点。
第 4 步:编写一个函数来预测和检测图像和视频中的对象
python
def predict(chosen_model, img, classes=[], conf=0.5):
if classes:
results = chosen_model.predict(img, classes=classes, conf=conf)
else:
results = chosen_model.predict(img, conf=conf)
return results
def predict_and_detect(chosen_model, img, classes=[], conf=0.5, rectangle_thickness=2, text_thickness=1):
results = predict(chosen_model, img, classes, conf=conf)
for result in results:
for box in result.boxes:
cv2.rectangle(img, (int(box.xyxy[0][0]), int(box.xyxy[0][1])),
(int(box.xyxy[0][2]), int(box.xyxy[0][3])), (255, 0, 0), rectangle_thickness)
cv2.putText(img, f"{result.names[int(box.cls[0])]}",
(int(box.xyxy[0][0]), int(box.xyxy[0][1]) - 10),
cv2.FONT_HERSHEY_PLAIN, 1, (255, 0, 0), text_thickness)
return img, results
predict()
功能
此函数采用三个参数:
chosen_model
:用于预测的训练模型img
:要进行预测的图像classes
:(可选)要将预测筛选到的类名列表conf
:(可选)要考虑的预测的最小置信度阈值
该函数首先检查是否提供了 classes
参数。如果是,则使用 classes
参数调用该chosen_model.predict()
方法,该参数仅将预测筛选为这些类。否则,将调用该 chosen_model.predict()
方法时不带 classes
参数,该参数将返回所有预测。
该 conf
参数用于筛选出置信度分数低于指定阈值的预测。这对于消除误报很有用。
该函数返回预测结果列表,其中每个结果都包含以下信息:
name
:预测类的名称conf
:预测的置信度分数box
:预测对象的边界框
predict_and_detect()
功能
此函数采用与 predict()
函数相同的参数,但除了预测结果外,它还返回带注释的图像。
该函数首先调用该 predict()
函数以获取预测结果。然后,它循环访问预测结果,并在每个预测对象周围绘制一个边界框。预测类的名称也写在边界框上方。
该函数返回一个包含带注释的图像和预测结果的元组。
以下是这两个函数之间差异的摘要:
- 该
predict()
函数仅返回预测结果,而该predict_and_detect()
函数还返回带注释的图像。 - 该
predict_and_detect()
函数是predict()
函数的包装器,这意味着它在内部调用函数predict()
。
第 5 步:使用 YOLOv9 检测图像
python
# read the image
image = cv2.imread("YourImagePath")
result_img, _ = predict_and_detect(model, image, classes=[], conf=0.5)
如果要检测特定类,只需在类列表classes
中输入对象的 ID 号即可。
第 6 步:保存并绘制结果图像
python
cv2.imshow("Image", result_img)
cv2.imwrite("YourSavePath", result_img)
cv2.waitKey(0)
第 7 步:使用 YOLOv9 检测视频
python
video_path = r"YourVideoPath"
cap = cv2.VideoCapture(video_path)
while True:
success, img = cap.read()
if not success:
break
result_img, _ = predict_and_detect(model, img, classes=[], conf=0.5)
cv2.imshow("Image", result_img)
cv2.waitKey(1)
第 8 步:保存结果视频
python
# 定义保存函数
def create_video_writer(video_cap, output_filename):
# grab the width, height, and fps of the frames in the video stream.
frame_width = int(video_cap.get(cv2.CAP_PROP_FRAME_WIDTH))
frame_height = int(video_cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
fps = int(video_cap.get(cv2.CAP_PROP_FPS))
#初始化
fourcc = cv2.VideoWriter_fourcc(*'MP4V')
writer = cv2.VideoWriter(output_filename, fourcc, fps,
(frame_width, frame_height))
return writer
只需使用上面的函数和代码即可
python
output_filename = "YourFilename"
writer = create_video_writer(cap, output_filename)
video_path = r"YourVideoPath"
cap = cv2.VideoCapture(video_path)
while True:
success, img = cap.read()
if not success:
break
result_img, _ = predict_and_detect(model, img, classes=[], conf=0.5)
writer.write(result_img)
cv2.imshow("Image", result_img)
cv2.waitKey(1)
writer.release()
结论
在本文中,我们学习了如何使用 YOLOv9 检测图像和视频中的对象。如果您觉得此代码有用,感谢点赞关注。
引用
YOLOv9论文:https://arxiv.org/abs/2402.13616
YOLOv9 源码地址:https://github.com/WongKinYiu/yolov9
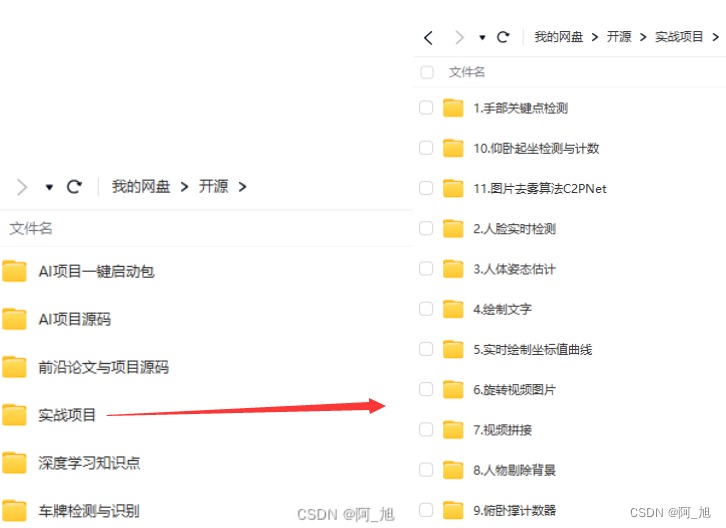
好了,这篇文章就介绍到这里,喜欢的小伙伴感谢给点个赞和关注,更多精彩内容持续更新~~
关于本篇文章大家有任何建议或意见,欢迎在评论区留言交流!