16.1、if语句
当C++程序必须决定是否执行某个操作时,通常使用if语句来实现选择。if有两种格式:if和if else。
if语句的语法与while相似:
if(text-condition)
statement
如果text-condition(测试条件)为true,则程序执行statement(语句)。后者可以是一条语句,或者语句块。如果测试条件为false,则程序将跳过语句。与循环测试一样,if测试条件也将被强制转换为bool值,因此0将被转换为false,非零为true。
#include<iostream>
int main()
{
using namespace std;
char ch;
int spaces = 0;
int total = 0;
cin.get(ch);
while (ch != '.')
{
if (ch == ' ')
++spaces;
++total;
cin.get(ch);
}
cout << spaces << " spaces, " << total;
cout << " characters total in sentence ";
return 0;
}

仅当ch为空格时,语句++spaces;才会被执行。因为语句++total;位于if语句的外面,因此在每轮循环中都将被执行。注意:字符总数中包括按回车键生成的换行符。
16.2、if else语句
if语句让程序决定是否执行特定的语句或语句块,而if else语句则让程序决定执行两条语句或语句块中的哪一条,这种语句对于选择其中一种操作很有用。if else语句的通用格式如下:
if (text-condition)
statement1
else
statement2
如果测试条件为true或非0,则程序将执行statement1,跳过statement2;如果测试条件为false或0,则程序跳过statement1,执行statement2。
#include <iostream>
int main()
{
using namespace std;
char ch;
cout << "Type,and I shall repeart." << endl;
cin.get(ch);
while (ch != '.')
{
if (ch == '\n')
cout << ch;
else
cout << ++ch;
cin.get(ch);
}
cout << endl << "Please excuse the slight confusion." << endl;
return 0;
}
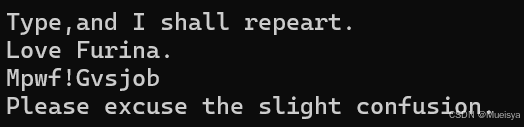
16.3、格式化if else语句
如果需要多条语句,需要用大括号将它们括起来,因为C++不会将它们视为一个代码块。如:
if(ch=='Z')
zerro++;
cout<<"Another Zorro candidate"<<endl;
else
dull++;
cout<<"Not a Zorro candidate"<<endl;
上面这段代码程序执行zerro++;后,视为一段简单的if语句,而后执行cout语句,但之后识别到else将会出错。如果像这样,就不会出错:
if(ch=='Z')
{
zerro++;
cout<<"Another Zorro candidate"<<endl;
}
else
{ dull++;
cout<<"Not a Zorro candidate"<<endl;
}
16.4、if else if else语句
其实if else语句不仅仅只有两个选择,可以将if else语句进行拓展来满足这种需求。例如:
if(ch=='A')
a_grade++;
else if(ch=='B')
b_grade++;
else
soso++;
因为else后面可以是一条语句或者语句块,因此可以在else语句后加上if else进行嵌套:
#include <iostream>
const int Fave = 32;
int main()
{
using namespace std;
int n;
cout << "Enter a number in the range 1-100 to find";
cout << " my favourite number : ";
do {
cin >> n;
if (n < Fave)
cout << "Too low -- guess again: ";
else if (n > Fave)
cout << "Too high -- gusee again: ";
else
cout << Fave << " is right!" << endl;
} while (n != Fave);
return 0;
}

上面的程序让用户输入一个值,不同输入有不同的结果。
16.5、逻辑表达式
假设你需要一个字符无论输入大写或小写都可以,你需要测试多种条件。而C++提供三种运算符来组合和修改已有表达式:逻辑OR(||)、逻辑AND(&&)和逻辑NOT(!)。
16.5.1、逻辑OR运算符(||):
C++采用逻辑OR运算符,将两个表达式组合在一起。如果原来表达式中的任何一个或者全部都为true,则得到的表达式为true,否则为false。下面是一些例子:
5==5||5==9
5>3||5>10
5>8||5<2
上面的表达式里前面两个结果为true,最后为false。由于||的优先级比关系运算符低,因此不需要在这些表达式中使用括号。
**C++规定,||运算符是个顺序点。也就是说先修改左侧的值,再对右侧的值进行判定。**例如:
i++<6||i==j 假设i的值原来是10,则在i和j进行比较时,i的值为11。另外如果左侧的表达式为true,则C++将不会去判断右侧的表达式。
#include <iostream>
int main()
{
using namespace std;
cout << "This program may reformat your hard disk" << endl
<< "and destory all your data." << endl
<<"Do you wish to continue?<y/n>";
char ch;
cin >> ch;
if (ch == 'y' || ch == 'Y')
cout << "You were warned!\a\a\n";
else if (ch == 'n' || ch == 'N')
cout << "A wish choice ... bye" << endl;
else
cout << "That wasn't a y or n!Apparently you "
"can't follow\ninstructions,so "
"I'll trash your disk anyway.\a\a\a\n";
return 0;
}
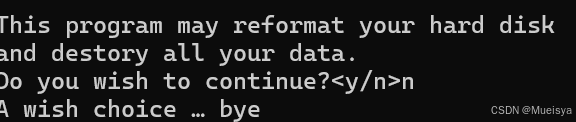
16.5.2、逻辑AND运算符:&&
&&与||运算符很多相同之处,唯一不同的是&&仅当两个表达式都为true得到的表达式的结果才为true。下面给一段有亿点长的程序(不是你也知道啊):
#include <iostream>
const int ArSize = 6;
int main()
{
using namespace std;
float naaq[ArSize];
cout << "Enter the NAQQS (New Age Awereness Quotients)"
<< "of\nyour neighbors.Program terminates "
<< "when you make\n" << ArSize << " entries "
<< "or enter a negative value." << endl;
int i = 0;
float temp;
cout << "First value: ";
cin >> temp;
while (i < ArSize && temp>0)
{
naaq[i] = temp;
++i;
if (i < ArSize)
{
cout << "Next value: ";
cin >> temp;
}
}
if (i == 0)
cout << "No data --bye" << endl;
else
{
cout << "Enter your NAAQ: ";
float you;
cin >> you;
int count = 0;
for (int j = 0; j < i; j++)
if (naaq[j] > you)
++count;
cout << count;
cout << " of your neighbors have greater awareness of" << endl
<< "the New Age than you do." << endl;
}
return 0;
}
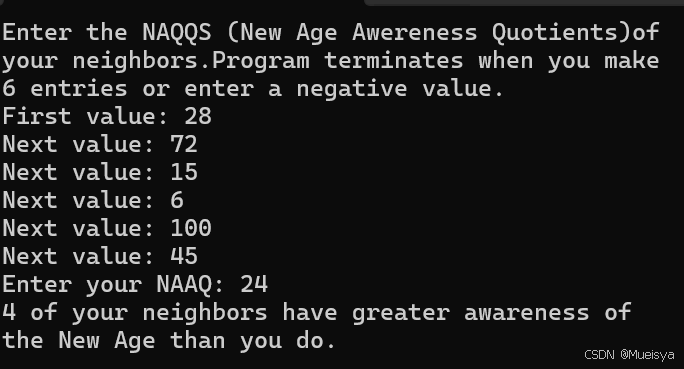
我们来看一下程序的输入部分:
cin >> temp;
while (i < ArSize && temp>0)
{
naaq[i] = temp;
++i;
if (i < ArSize)
{
cout << "Next value: ";
cin >> temp;
}
}
该程序将第一个输入值读入到临时变量temp中,然后while测试条件查看数组是否还有空间(i<ArSize)以及输入值是否为负值。如果满足条件,则将temp的值复制到数组中。
16.5.3、用&&来设置取值范围
&&运算符还允许建立一系列的if else if else语句,其中每种选择都对应一个特定的取值范围。
#include <iostream>
const char* qualify[4] =
{ "10000-meter race.\n",
"mud tug - of - war.\n",
"masters canoe jousting.\n",
"pie-throwing festival.\n"
};
int main()
{
using namespace std;
int age;
cout << "Enter your age in years: ";
cin >> age;
int index;
if (age > 17 && age < 35)
index = 0;
else if (age >= 35 && age < 50)
index = 1;
else if (age >= 50 && age < 65)
index = 2;
else
index = 3;
cout << "You qualify for the " << qualify[index];
return 0;
}

表达式age>17&&age<35测试年龄是否在这两个值之间,我输入了24,则省略后面的测试。使用时应确保取值范围之间既没有缝隙,也没有重复。
16.5.4、逻辑NOT表达式:!
!运算符将它后面的表达式的真值取反。也就是说,如果expression为true或非0,则!expression是false。其实就像下面表达式:
if(!(x>5))==if(x<=5)
下面给一段程序:
#include<iostream>
#include<climits>
bool is_int(double);
int main()
{
using namespace std;
double num;
cout << "Yo,dude!Enter an integer value: ";
cin >> num;
while (!is_int(num))
{
cout << "Out of range -- please try again: ";
cin >> num;
}
int val = int(num);
cout << "You've entered the integer " << val << "\nBye\n";
return 0;
}
bool is_int(double x)
{
if (x <= INT_MAX && x >= INT_MIN)
return true;
else return false;
}

这个程序来筛选可赋给int变量的数字输入。
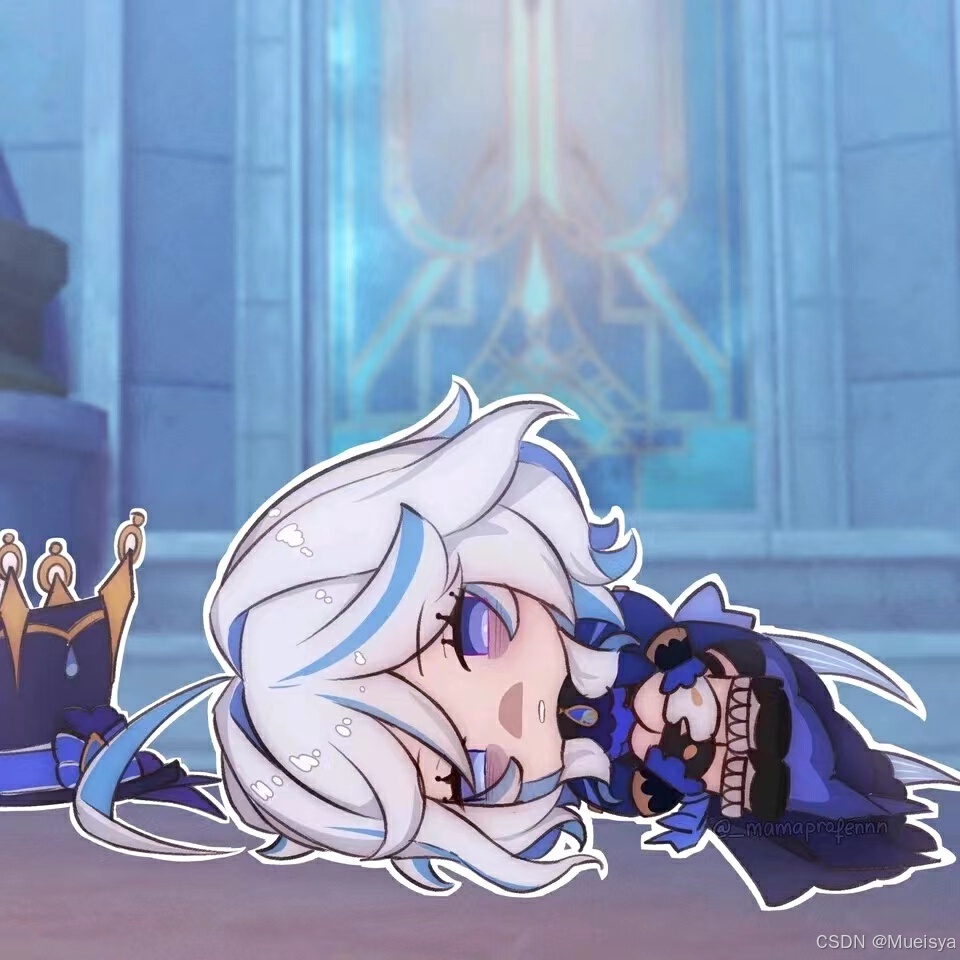