python
git clone https://github.com/PaddlePaddle/PaddleSeg.git
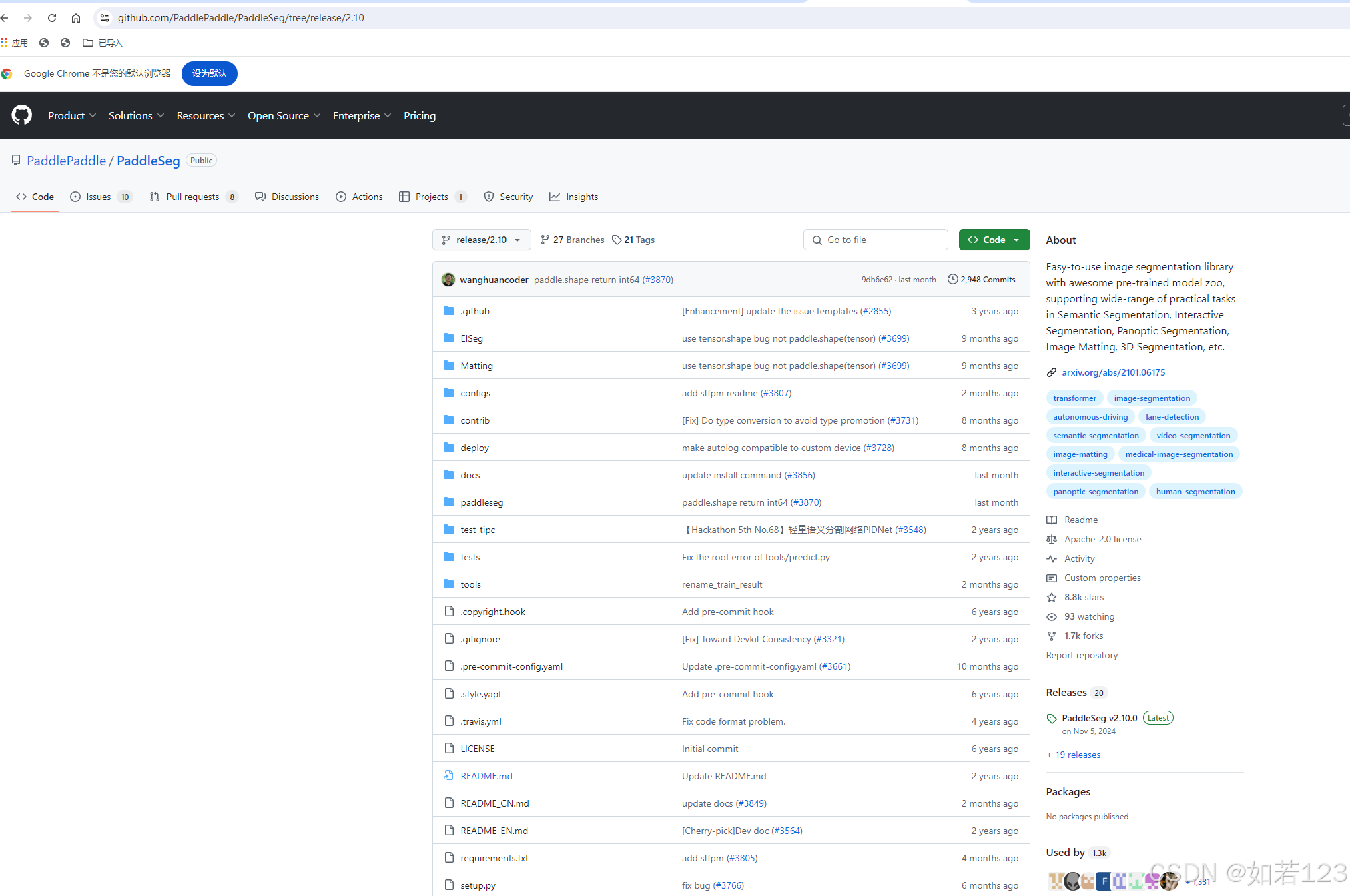
python
# 在ipynb里面运行
cd PaddleSeg
python
import sys
sys.path.append('/home/aistudio/work/PaddleSeg')
python
import os
# 配置文件夹路径
folder_path = "/home/aistudio/work/PaddleSeg/configs"
# 遍历文件夹,寻找所有 .yml 文件并存储到字典中
# key 是文件名(不带扩展名),value 是文件的完整路径
yml_files = {}
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.lower().endswith(".yml"):
file_path = os.path.join(root, file)
file_name_without_extension = os.path.splitext(file)[0] # 获取文件名(不带扩展名)
yml_files[file_name_without_extension] = file_path # 保存文件路径
print(file_path) # 打印找到的配置文件路径
# 读取包含模型 URL 的文件
file_to_read = "/home/aistudio/work/PaddleSeg/voc/pascal_voc12_urls_extracted.txt"
url_lines = {}
if os.path.exists(file_to_read):
with open(file_to_read, 'r') as f:
lines = f.readlines()
for line in lines:
url = line.strip() # 去除换行符和多余空格
parsed_name = url.split("/")[-2] # 提取 URL 中的模型名称部分
url_lines[parsed_name] = url # 保存模型名称与 URL 的映射
print(url) # 打印提取的 URL
else:
print(f"File not found: {file_to_read}") # 如果文件不存在,打印提示信息
# 拼接并运行预测命令
base_command = "python tools/predict.py --config {} --model_path {} --image_path /home/aistudio/data/data117064/voctestimg --save_dir {}"
for model_name, model_path in url_lines.items():
if model_name in yml_files: # 检查模型名称是否有对应的配置文件
config_file = yml_files[model_name] # 获取匹配的配置文件路径
save_dir = f"output/{model_name}" # 保存路径按照模型名称组织
os.makedirs(save_dir, exist_ok=True) # 确保保存目录存在
command = base_command.format(config_file, model_path, save_dir) # 填充命令模板
print(f"Executing: {command}") # 打印正在执行的命令
os.system(command) # 执行命令
else:
print(f"No matching config file found for model: {model_name}") # 如果没有匹配的配置文件,打印提示信息