1. 项目源代码
为了方便演示,以及大家上手实验,我搭建了一个简单的注册登录的后台,在不加 Redis 的情况下的源代码如下
通过网盘分享的文件:study-无redis.zip
链接: https://pan.baidu.com/s/1etc29in_6q8CDOFm-Gb7Nw?pwd=yuer 提取码: yuer
2. 数据库结构
数据库结构如下所示:
/*
Navicat Premium Data Transfer
Source Server : localhost_3306
Source Server Type : MySQL
Source Server Version : 80016
Source Host : localhost:3306
Source Schema : redis
Target Server Type : MySQL
Target Server Version : 80016
File Encoding : 65001
Date: 07/04/2025 10:55:27
*/
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
-- ----------------------------
-- Table structure for usr
-- ----------------------------
DROP TABLE IF EXISTS `usr`;
CREATE TABLE `usr` (
`uid` int(11) NOT NULL,
`username` varchar(255) CHARACTER SET utf8 COLLATE utf8_bin NULL DEFAULT NULL,
`password` varchar(255) CHARACTER SET utf8 COLLATE utf8_bin NULL DEFAULT NULL,
PRIMARY KEY (`uid`) USING BTREE
) ENGINE = InnoDB CHARACTER SET = utf8 COLLATE = utf8_bin ROW_FORMAT = Dynamic;
-- ----------------------------
-- Records of usr
-- ----------------------------
INSERT INTO `usr` VALUES (1, 'yuer', 'yuerpass');
INSERT INTO `usr` VALUES (686, '123', '456');
SET FOREIGN_KEY_CHECKS = 1;
3. Redis集成
接下来我们添加 Redis 支持:
3.1 添加 Redis 依赖
<!-- Redis依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- 添加Jackson序列化依赖 -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
3.2 配置 Redis
在 config 包下新建一个 RedisConfig 类:
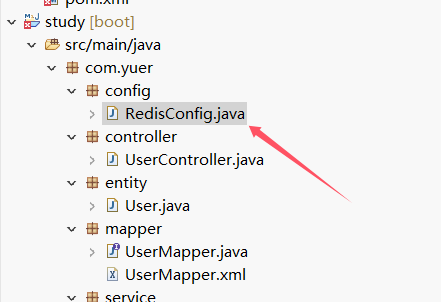
package com.yuer.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
public class RedisConfig {
@Bean
public RedisTemplateredisTemplate(RedisConnectionFactory connectionFactory) {
RedisTemplateredisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(connectionFactory);
// 使用StringRedisSerializer来序列化和反序列化redis的key值
redisTemplate.setKeySerializer(new StringRedisSerializer());
// 使用GenericJackson2JsonRedisSerializer来序列化和反序列化redis的value值
GenericJackson2JsonRedisSerializer jsonRedisSerializer = new GenericJackson2JsonRedisSerializer();
redisTemplate.setValueSerializer(jsonRedisSerializer);
// Hash的key也采用StringRedisSerializer的序列化方式
redisTemplate.setHashKeySerializer(new StringRedisSerializer());
// Hash的value也采用GenericJackson2JsonRedisSerializer的序列化方式
redisTemplate.setHashValueSerializer(jsonRedisSerializer);
redisTemplate.afterPropertiesSet();
return redisTemplate;
}
}
3.3 配置 application.properties
spring.redis.host=localhost
spring.redis.port=6379
# spring.redis.password=
spring.redis.database=0
spring.redis.timeout=3000
3.4 修改 UserService 实现类
修改 userservice.impl 中的登录方法:
@Override
public User login(String username, String password) {
// 根据用户名查询用户
User user = userMapper.selectByUsername(username);
// 从缓存中读取登录的数据
User cachedUser = (User) redisTemplate.opsForValue().get("user:" + user.getUid().toString());
if(cachedUser != null) {
System.out.print("缓存命中!登录成功!");
} else {
System.out.print("未寻找到缓存,准备使用mysql查询");
}
// 如果用户存在且密码匹配,则登录成功,通过数据库,并且缓存redis
if (user != null && password.equals(user.getPassword())) {
redisTemplate.opsForValue().set("user:" + user.getUid().toString(), user);
return user;
}
// 登录失败
return null;
}
4. 登录流程演示(测试Redis)
当用户首次登录时,需要从 MySQL 查询用户信息,之后可以缓存登录的数据。
4.1 第一次登录
第一次登录需要查询 MySQL:

4.2 第二次登录
第二次登录时,已缓存登录数据:

5. 清除 Redis 缓存
如果需要清除 Redis 缓存数据,可以使用以下命令:
# 连接到 Redis
redis-cli
# 清除当前数据库缓存
flushdb
# 清除所有缓存
FLUSHALL