hi !
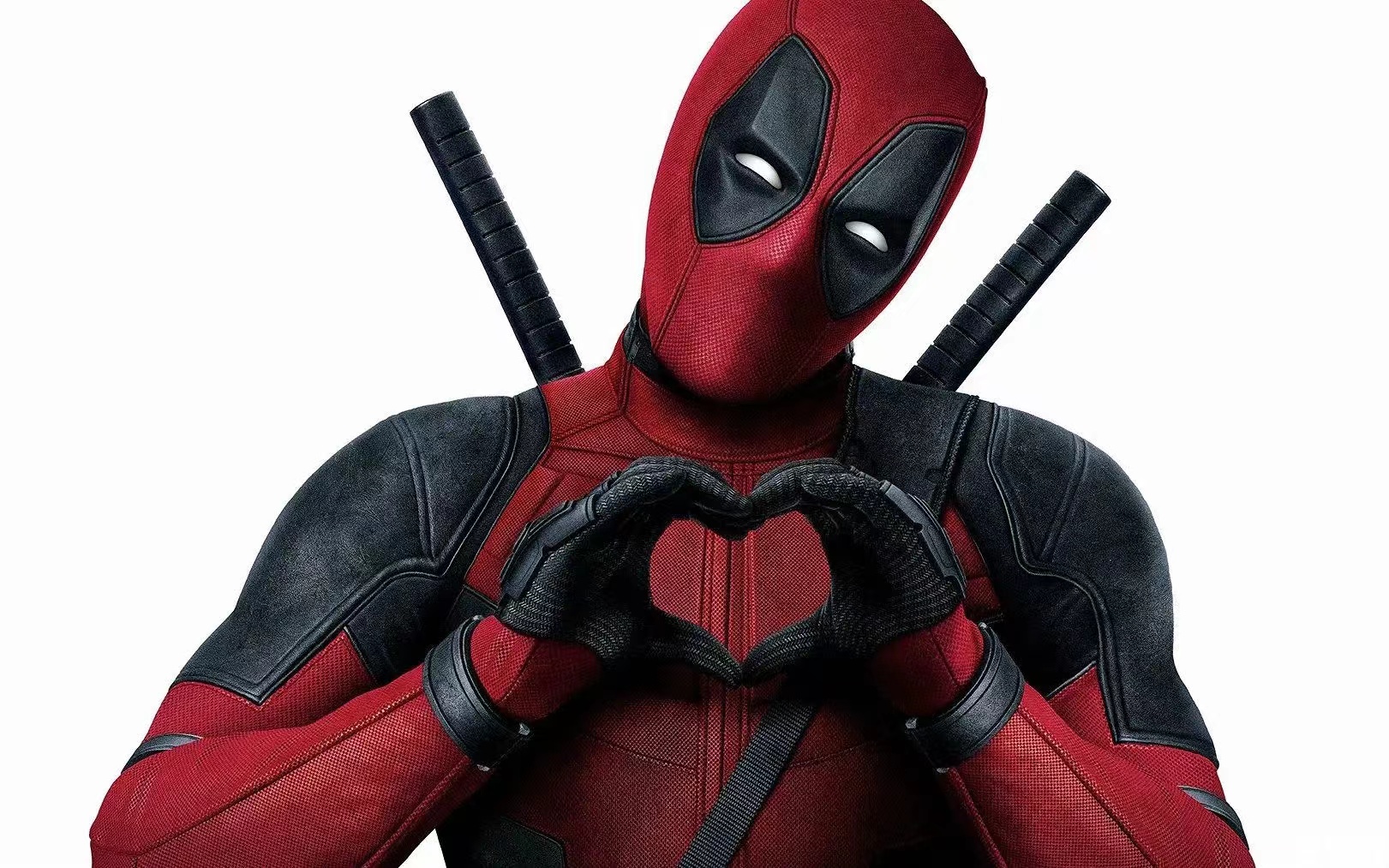
目录
[9、 环形链表 ||](#9、 环形链表 ||)
9、 环形链表 ||
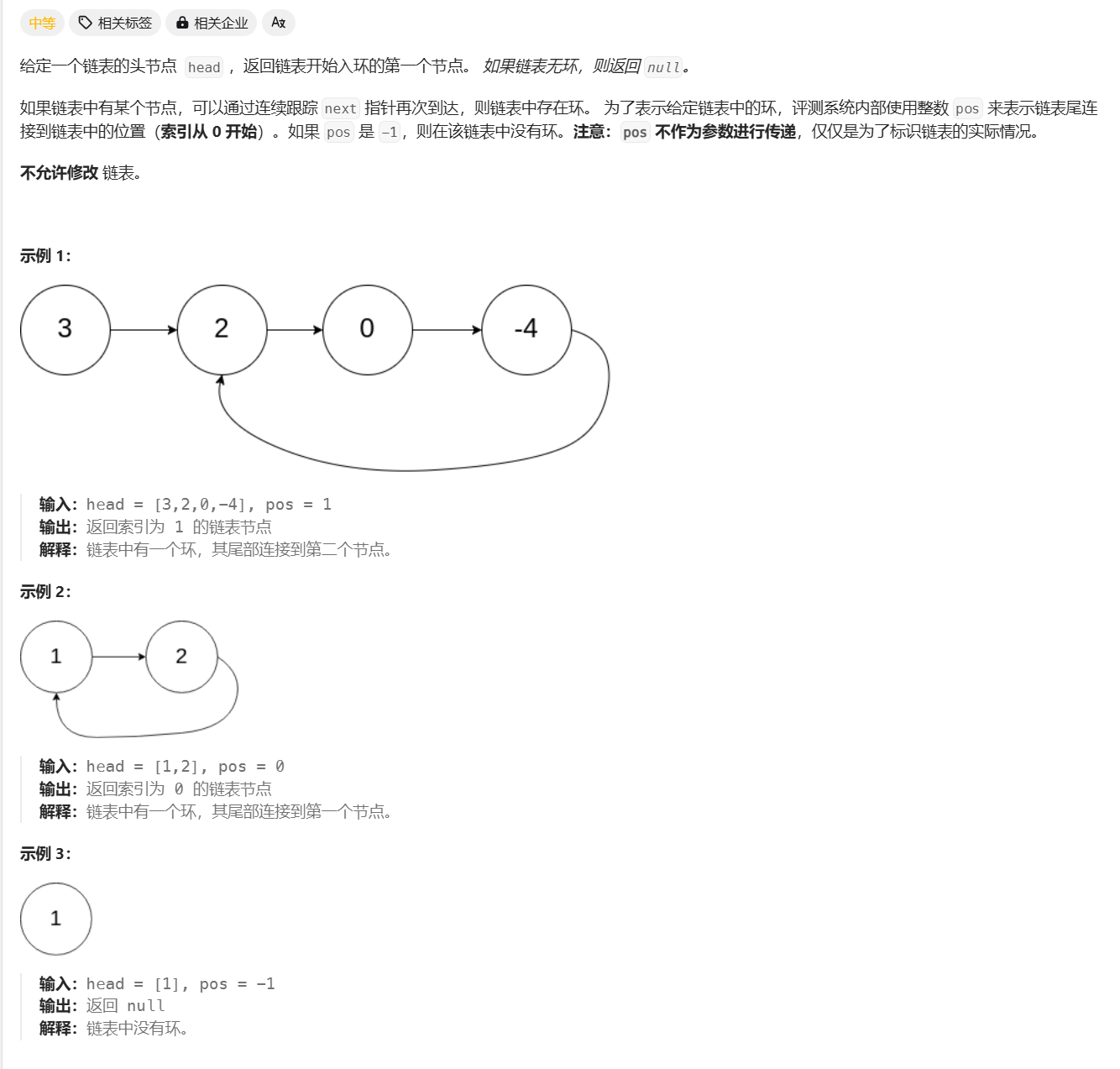
【图解】
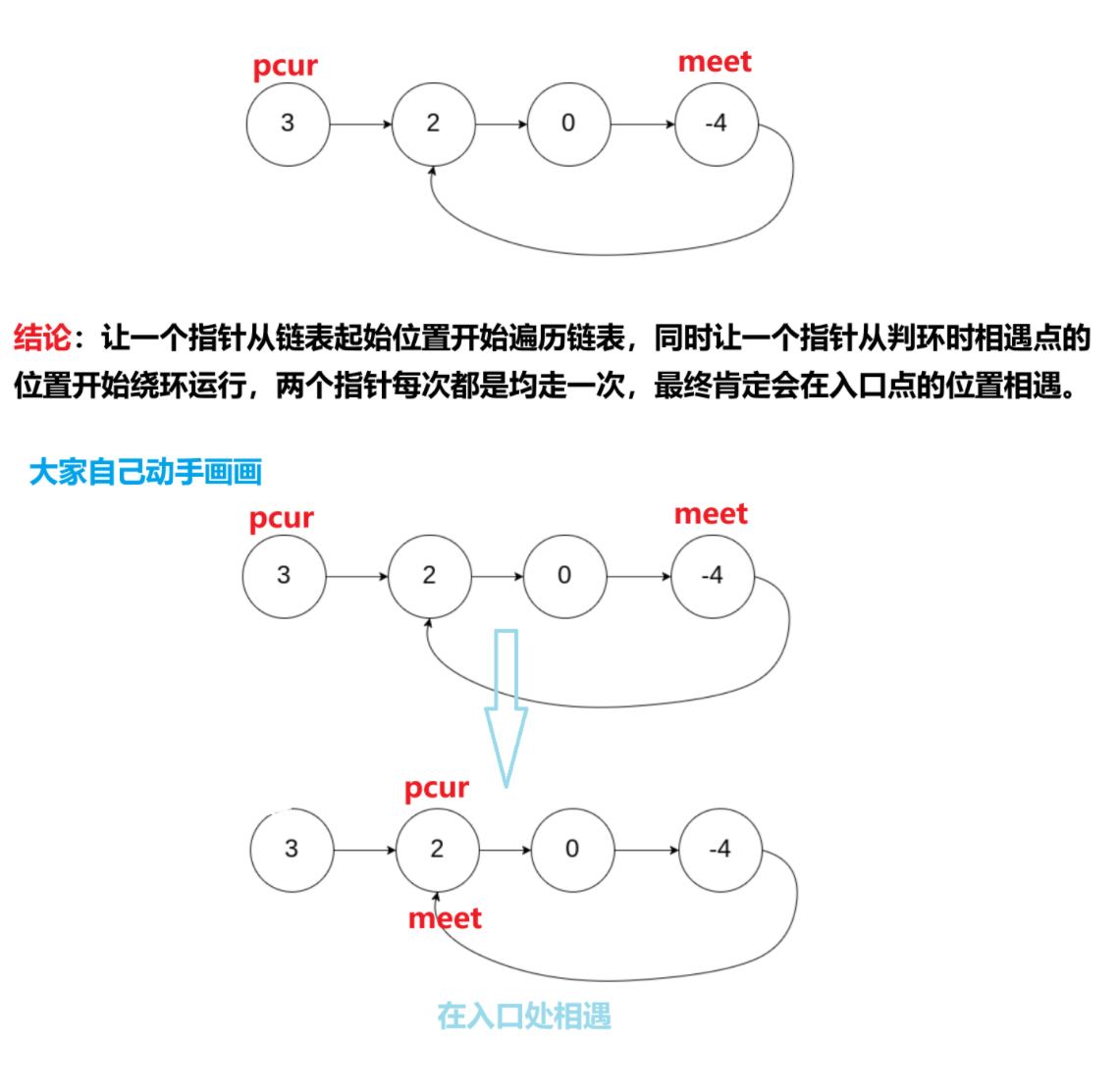
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
typedef struct ListNode ListNode;
struct ListNode *detectCycle(struct ListNode *head) {
//找相遇节点
ListNode* slow=head;
ListNode* fast=head;
while(fast&&fast->next)
{
slow=slow->next;
fast=fast->next->next;
if(slow==fast)
{
//相遇,开始遍历
ListNode* pcur=head;
while(slow!=pcur)
{
slow=slow->next;
pcur=pcur->next;
}
return slow;
}
}
return NULL;
}
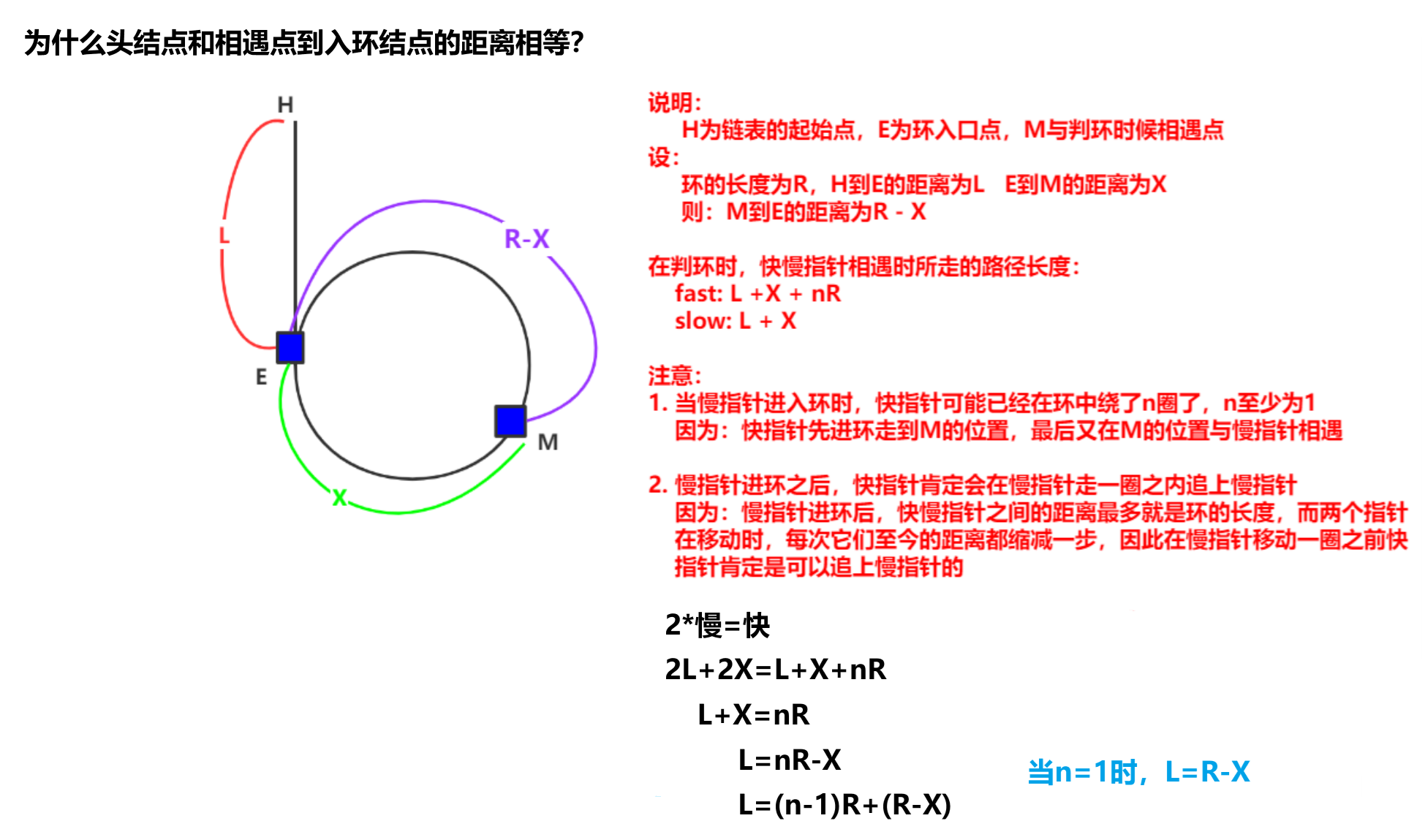
10、随机链表的复制
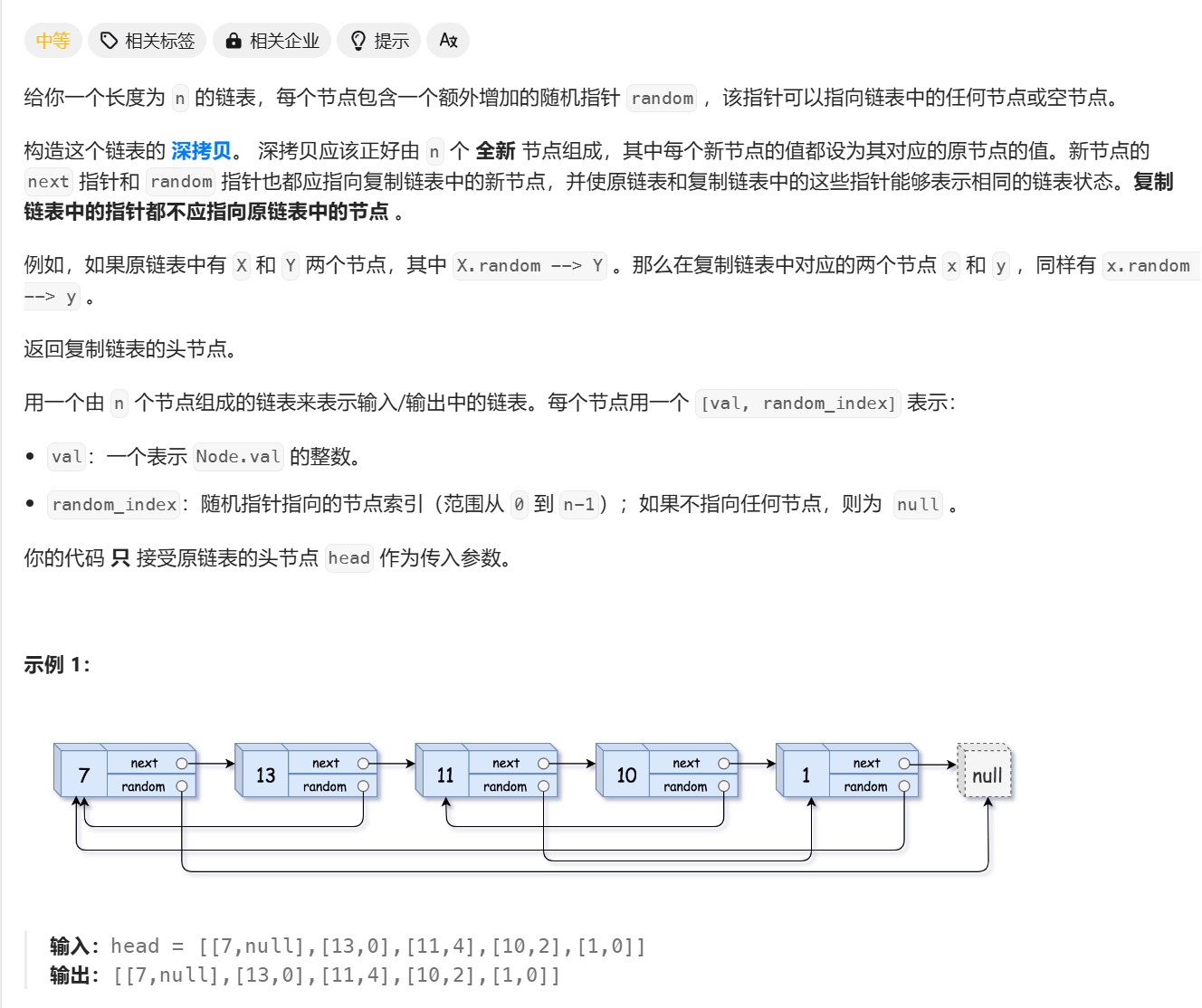
【图解】
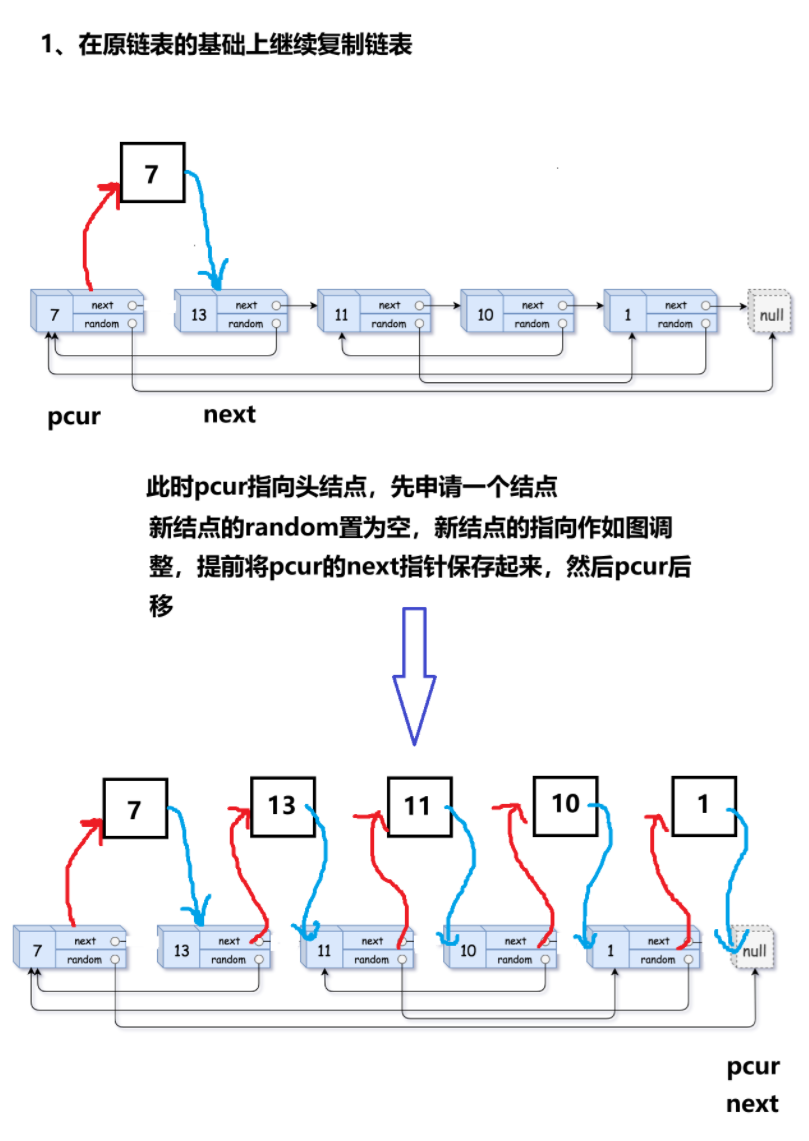
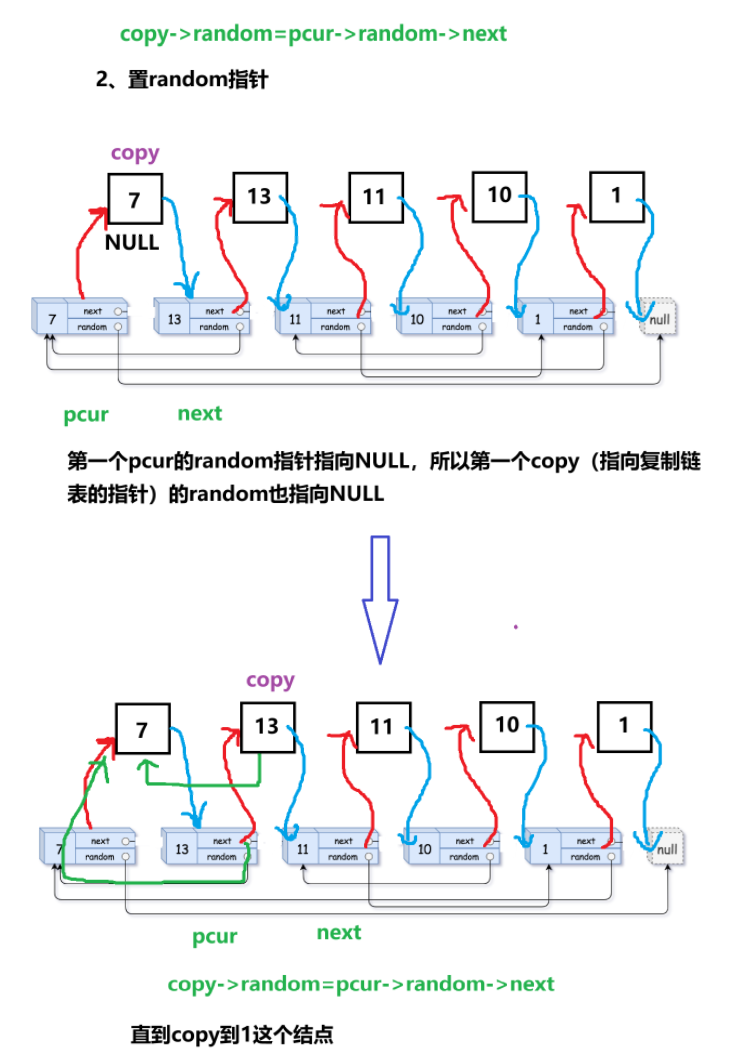
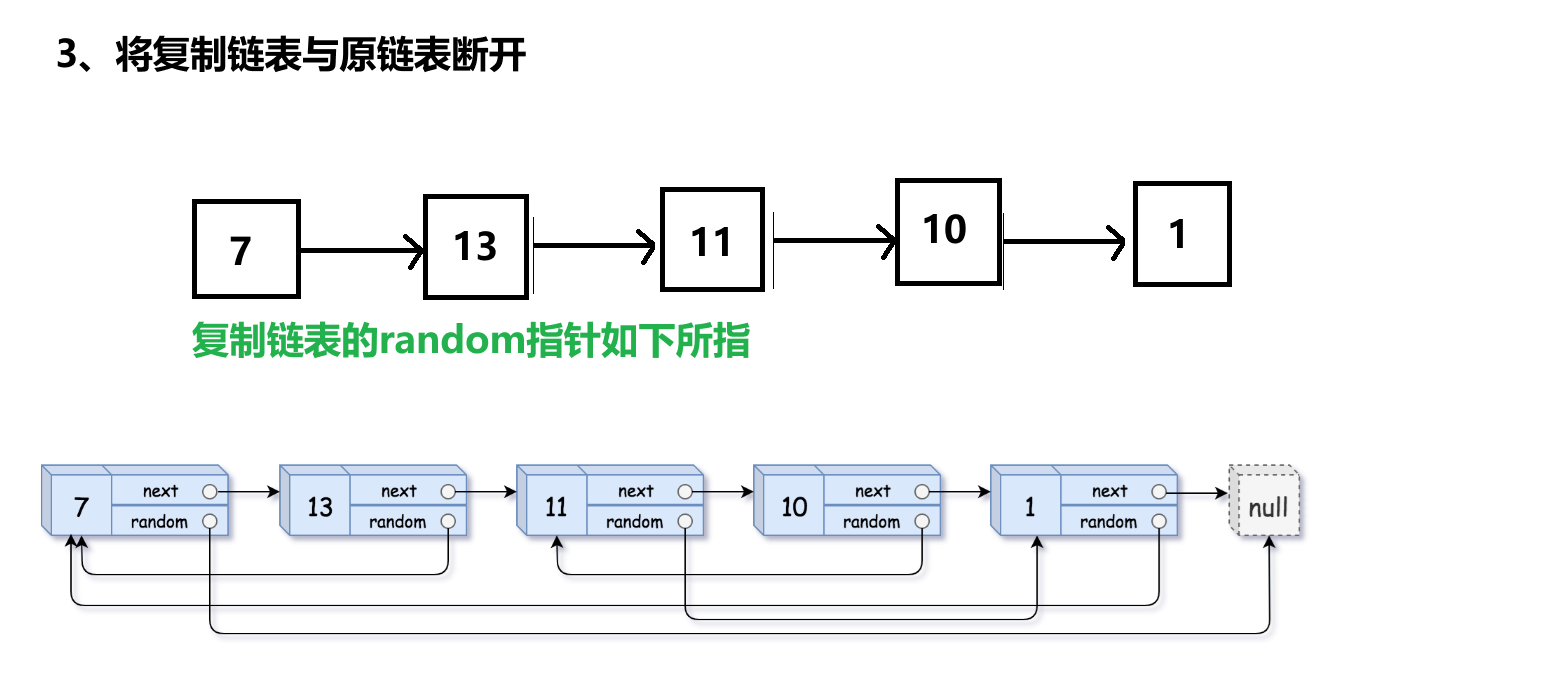
【思路】
1、在原链表的基础上复制新链表
2、置random指针
3、(改变next指针指向)将复制链表和原链表断开
cs
/**
* Definition for a Node.
* struct Node {
* int val;
* struct Node *next;
* struct Node *random;
* };
*/
typedef struct Node Node;
//创建新结点
Node* BuyNode(int x)
{
Node* newnode=(Node*)malloc(sizeof(Node));
newnode->val=x;
newnode->next=newnode->random=NULL;
return newnode;
}
//复制结点
void AddNode(Node* phead)
{
Node* pcur=phead;
while(pcur)
{
Node* Next=pcur->next;
//创建新结点
Node* newnode=BuyNode(pcur->val);
newnode->next=Next;
pcur->next=newnode;
pcur=Next;
}
}
struct Node* copyRandomList(struct Node* head) {
if(head==NULL)
{
return NULL;
}
//第一步:复制结点
AddNode(head);
//第二步:置random
Node* pcur=head;
while(pcur)
{
Node* copy=pcur->next;
if(pcur->random!=NULL)
{
copy->random=pcur->random->next;
}
pcur=copy->next;
}
//第三步:断开链表
pcur=head;
Node* newTail,*newHead;
newTail=newHead=pcur->next;
while(pcur->next->next)
{
pcur=pcur->next->next;
newTail->next=pcur->next;
newTail=newTail->next;
}
return newHead;
}
这十道题做完理解透,想不提升都难
完------
------------------------------------------------ 终章 ------------------------------------------------
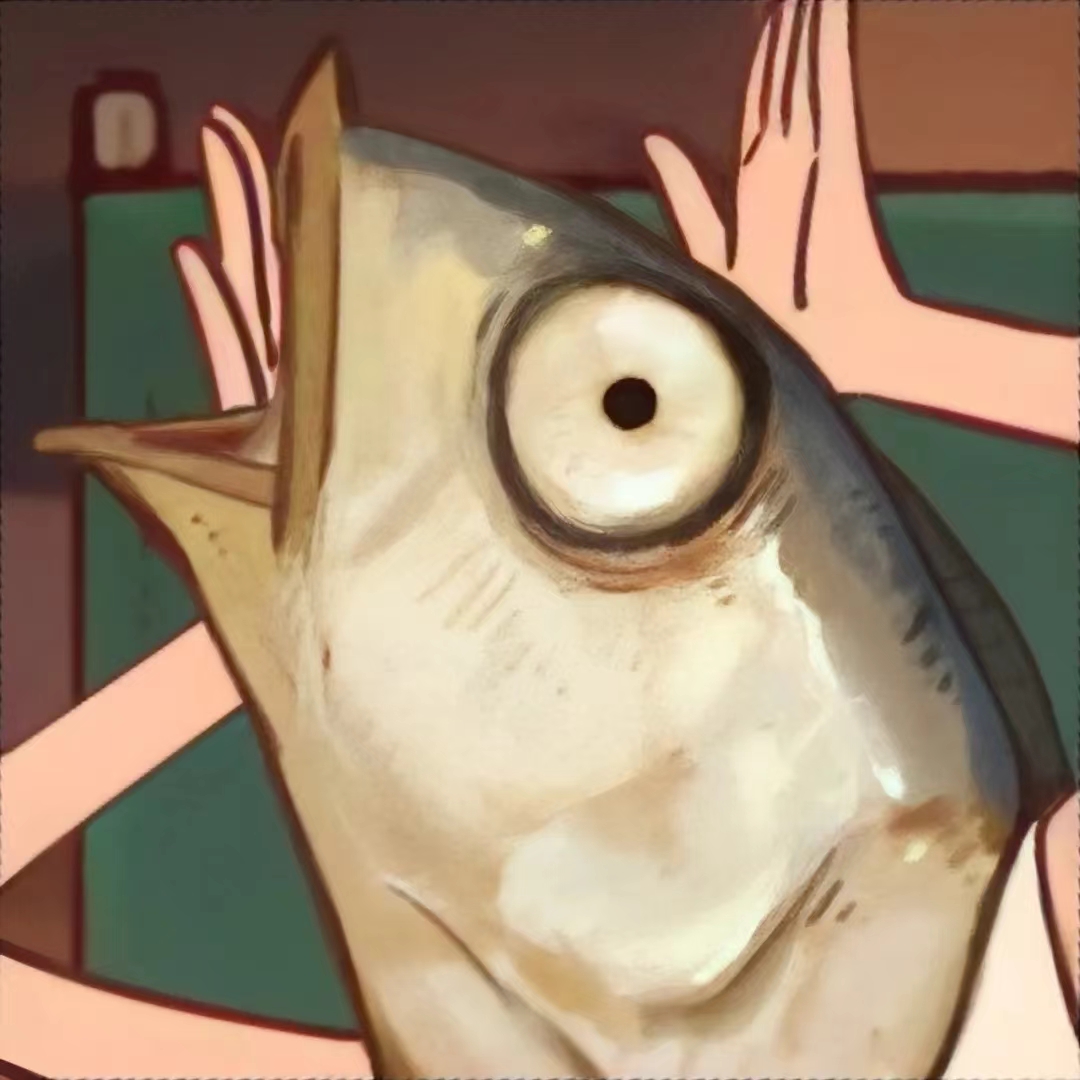
拜拜------