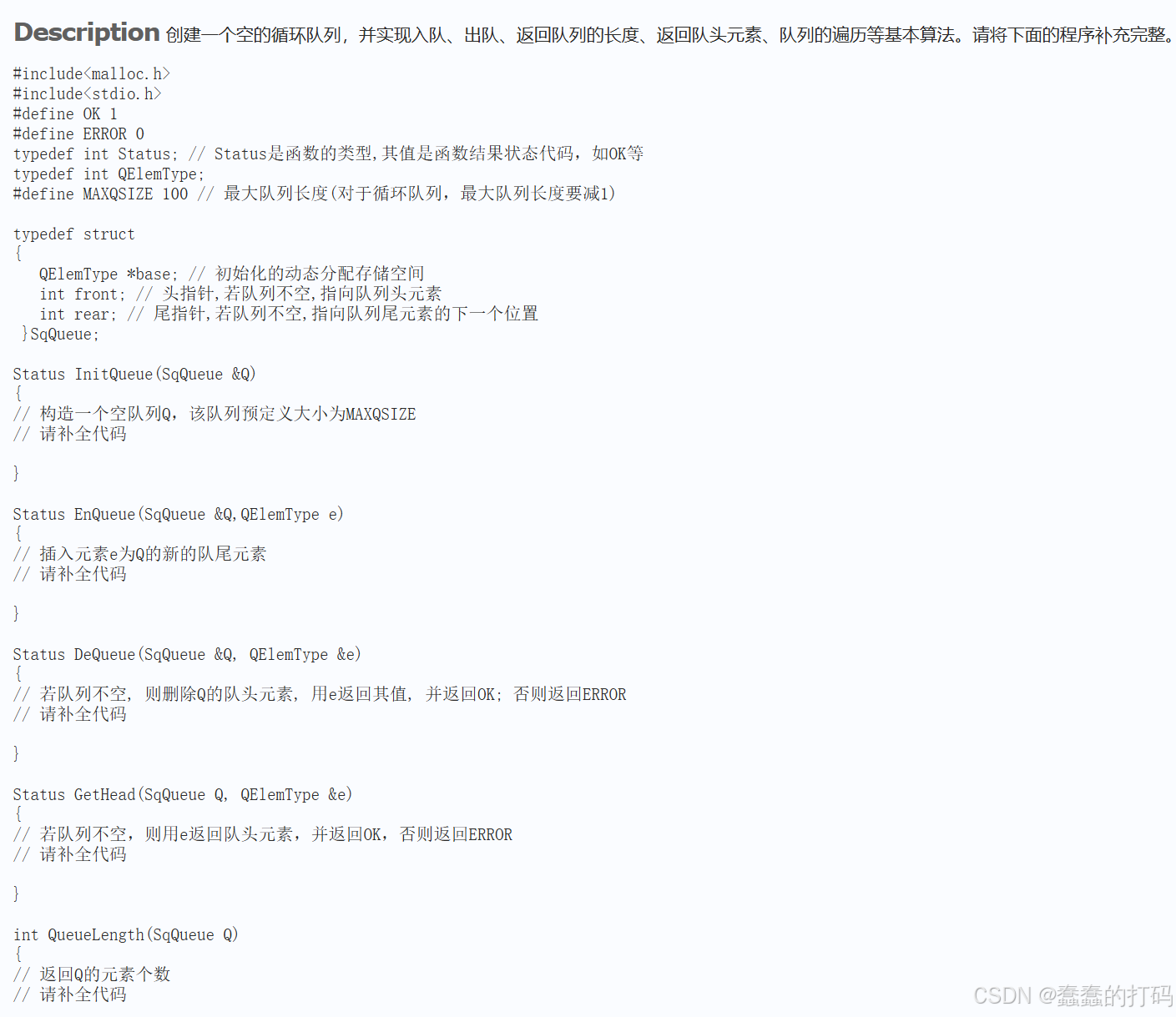
思路
-
**初始化队列**:分配初始大小为 `MAXQSIZE` 的内存空间,并将 `front` 和 `rear` 指针初始化为 0。
-
**入队**:检查队列是否已满,如果未满则将新元素插入队尾,并更新 `rear` 指针。
-
**出队**:检查队列是否为空,如果不为空则删除队头元素,并更新 `front` 指针。
-
**获取队头元素**:检查队列是否为空,如果不为空则返回队头元素。
-
**获取队列的长度**:计算并返回队列的元素个数。
-
**遍历队列**:从队头到队尾依次输出队列中的每个元素。
伪代码
```
function InitQueue(Q):
Q.base = allocate memory of size MAXQSIZE
Q.front = 0
Q.rear = 0
return OK
function EnQueue(Q, e):
if (Q.rear + 1) % MAXQSIZE == Q.front:
return ERROR
Q.base[Q.rear] = e
Q.rear = (Q.rear + 1) % MAXQSIZE
return OK
function DeQueue(Q, e):
if Q.front == Q.rear:
return ERROR
e = Q.base[Q.front]
Q.front = (Q.front + 1) % MAXQSIZE
return OK
function GetHead(Q, e):
if Q.front == Q.rear:
return ERROR
e = Q.base[Q.front]
return OK
function QueueLength(Q):
return (Q.rear - Q.front + MAXQSIZE) % MAXQSIZE
function QueueTraverse(Q):
if Q.front == Q.rear:
print "The Queue is Empty!"
else:
print "The Queue is: "
i = Q.front
while i != Q.rear:
print Q.base[i]
i = (i + 1) % MAXQSIZE
return OK
```
C++代码
cpp
#include <malloc.h>
#include <stdio.h>
#define OK 1
#define ERROR 0
typedef int Status; // Status是函数的类型,其值是函数结果状态代码,如OK等
typedef int QElemType;
#define MAXQSIZE 100 // 最大队列长度(对于循环队列,最大队列长度要减1)
typedef struct {
QElemType *base; // 初始化的动态分配存储空间
int front; // 头指针,若队列不空,指向队列头元素
int rear; // 尾指针,若队列不空,指向队列尾元素的下一个位置
} SqQueue;
Status InitQueue(SqQueue &Q) {
Q.base = (QElemType *)malloc(MAXQSIZE * sizeof(QElemType));
if (!Q.base) return ERROR;
Q.front = 0;
Q.rear = 0;
return OK;
}
Status EnQueue(SqQueue &Q, QElemType e) {
if ((Q.rear + 1) % MAXQSIZE == Q.front) return ERROR; // 队列满
Q.base[Q.rear] = e;
Q.rear = (Q.rear + 1) % MAXQSIZE;
return OK;
}
Status DeQueue(SqQueue &Q, QElemType &e) {
if (Q.front == Q.rear) return ERROR; // 队列空
e = Q.base[Q.front];
Q.front = (Q.front + 1) % MAXQSIZE;
return OK;
}
Status GetHead(SqQueue Q, QElemType &e) {
if (Q.front == Q.rear) return ERROR; // 队列空
e = Q.base[Q.front];
return OK;
}
int QueueLength(SqQueue Q) {
return (Q.rear - Q.front + MAXQSIZE) % MAXQSIZE;
}
Status QueueTraverse(SqQueue Q) {
int i = Q.front;
if (Q.front == Q.rear) {
printf("The Queue is Empty!\n");
} else {
printf("The Queue is: ");
while (i != Q.rear) {
printf("%d ", Q.base[i]);
i = (i + 1) % MAXQSIZE;
}
printf("\n");
}
return OK;
}
int main() {
int a;
SqQueue S;
QElemType x, e;
if (InitQueue(S) == OK) {
printf("A Queue Has Created.\n");
}
while (1) {
printf("1:Enter \n2:Delete \n3:Get the Front \n4:Return the Length of the Queue\n5:Load the Queue\n0:Exit\nPlease choose:\n");
scanf("%d", &a);
switch (a) {
case 1:
scanf("%d", &x);
if (EnQueue(S, x) == ERROR) printf("Enter Error!\n");
else printf("The Element %d is Successfully Entered!\n", x);
break;
case 2:
if (DeQueue(S, e) == ERROR) printf("Delete Error!\n");
else printf("The Element %d is Successfully Deleted!\n", e);
break;
case 3:
if (GetHead(S, e) == ERROR) printf("Get Head Error!\n");
else printf("The Head of the Queue is %d!\n", e);
break;
case 4:
printf("The Length of the Queue is %d!\n", QueueLength(S));
break;
case 5:
QueueTraverse(S);
break;
case 0:
return 1;
}
}
}