Write an algorithm to determine if a number is "happy"
A happy number is a number defined by the following process: Starting with any positive integer, replace the number by the sum of thesquares of its digits,
and repeat the process until the number equals 1 (where it will stay, or it loops endlessly in a cycle which does notinclude 1. Those numbers for which this process ends in 1 are happy numbers.
Input:19
Output:true
Explanation
1^2 + 9^2 = 82
8^2 + 2^2 = 68
6^2 + 8^2 = 100
1^2 + 0^2 + 0^2 = 1
循环检测 是不是在1轮回 快慢指针~
cpp
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define DEBUG
int next_n(int n){
int r=0;
while(n != 0){
int d = n % 10;
n /= 10;
r += d*d;
}
return r;
}
bool contains(int *history,int size,int n){
for(int i=0; i<size ;++i){
if(history[i] == n){
return true;
}
}
return false;
}
bool ishappy(int n){
int slow=n;
int fast=n;
do{
slow = next_n(slow);
fast = next_n(next_n(fast));
#ifdef DEBUG
printf("%d %d \n",slow,fast);
#endif
} while (slow != fast);
return false;
}
int main()
{
ishappy(19);
if(ishappy)
printf("yes");
else printf("no");
return 0;
}
slow 和 fast 都初始化为输入的数字 n。
slow 每次移动一步,即计算一次数字的平方和;fast 每次移动两步,计算两次平方和。
在循环中,如果 slow == fast,则表示数字进入了循环,不会到达 1。
但是,在这段代码中,无论循环如何执行,最后的结果总是返回 false,这个逻辑需要进一步调整。
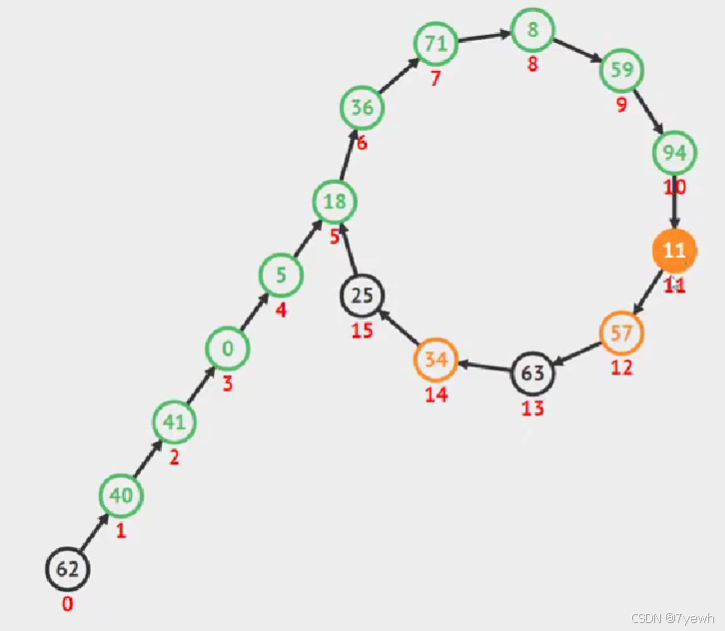