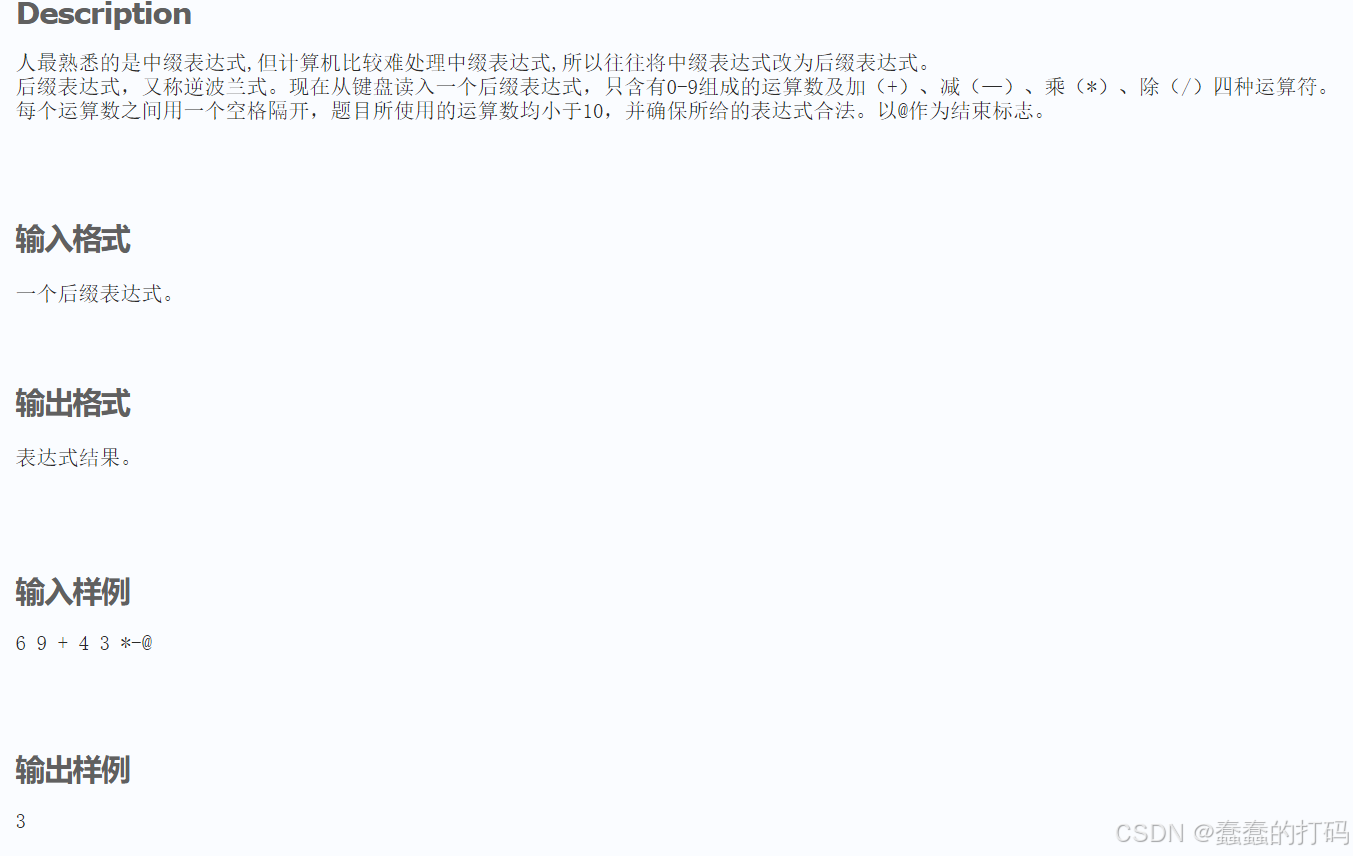
思路
-
**输入处理**:读取输入的后缀表达式,去掉末尾的`@`符号。
-
**使用栈计算后缀表达式**:
-
遍历表达式中的每个字符。
-
如果是数字,压入栈中。
-
如果是运算符,从栈中弹出两个数字进行运算,并将结果压入栈中。
- **输出结果**:栈中最后剩下的数字即为表达式的结果。
伪代码
```
function evaluate_postfix(expression):
stack = []
for token in expression.split():
if token is a digit:
stack.push(int(token))
else if token is an operator:
b = stack.pop()
a = stack.pop()
result = perform_operation(a, b, token)
stack.push(result)
return stack.pop()
function perform_operation(a, b, operator):
if operator == '+':
return a + b
if operator == '-':
return a - b
if operator == '*':
return a * b
if operator == '/':
return a / b
function main():
while input is not EOF:
expression = read_input().strip('@')
result = evaluate_postfix(expression)
print(result)
```
C++代码
cpp
#include <iostream>
#include <stack>
#include <sstream>
#include <string>
int main() {
std::string input;
std::getline(std::cin, input);
std::stack<int> s;
std::istringstream iss(input);
std::string token;
while (iss >> token) {
if (token == "@") {
break;
} else if (isdigit(token[0])) {
s.push(std::stoi(token));
} else {
for (char op : token) {
if (op == '@') {
break;
}
int b = s.top(); s.pop();
int a = s.top(); s.pop();
if (op == '+') {
s.push(a + b);
} else if (op == '-') {
s.push(a - b);
} else if (op == '*') {
s.push(a * b);
} else if (op == '/') {
s.push(a / b);
}
}
}
}
std::cout << s.top() << std::endl;
return 0;
}
总结
-
**输入处理**:读取并去掉末尾的`@`符号。
-
**使用栈计算后缀表达式**:遍历表达式,数字压栈,运算符弹出两个数字计算并压栈。
-
**输出结果**:栈中最后剩下的数字即为结果。