介绍
Redis 哨兵模式(Sentinel)是 Redis 集群的高可用解决方案,它主要用于监控 Redis 主从复制架构中的主节点和从节点的状态,并提供故障转移和通知功能。通过 Redis 哨兵模式,可以保证 Redis 服务的高可用性和自动故障恢复。
- 监控 (Monitoring):哨兵会监控主节点和从节点的健康状况。如果检测到某个节点故障(例如主节点不可达),哨兵会启动故障转移流程。
- 故障转移 (Failover):当主节点出现故障时,哨兵会自动选举新的主节点,并将一个从节点提升为新的主节点。此时,所有的写操作都会发送到新的主节点。
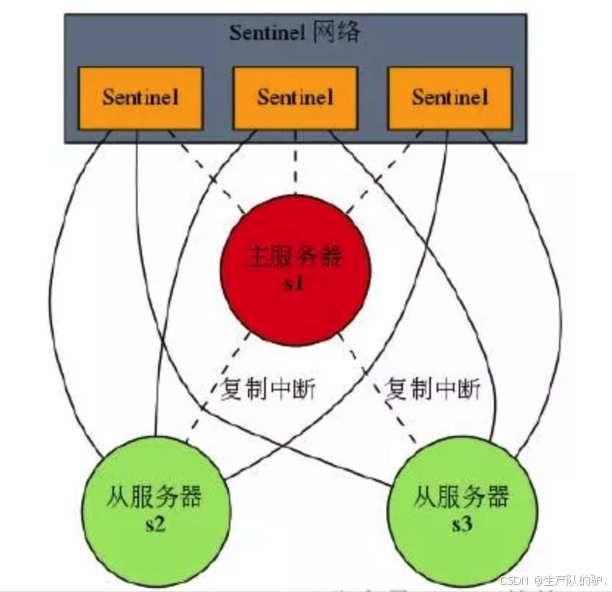
docker-compose.yml
yaml
version: "3.2"
services:
r1:
image: redis
container_name: r1
network_mode: "host"
entrypoint: ["redis-server", "--port", "7001", "--requirepass", "yourpassword","--masterauth", "yourpassword"]
r2:
image: redis
container_name: r2
network_mode: "host"
entrypoint: ["redis-server", "--port", "7002", "--slaveof", "103.116.247.215", "7001", "--requirepass", "yourpassword", "--masterauth", "yourpassword"]
r3:
image: redis
container_name: r3
network_mode: "host"
entrypoint: ["redis-server", "--port", "7003", "--slaveof", "103.116.247.215", "7001", "--requirepass", "yourpassword", "--masterauth", "yourpassword"]
s1:
image: redis
container_name: s1
volumes:
- /opt/s1:/etc/redis
network_mode: "host"
entrypoint: ["redis-sentinel", "/etc/redis/sentinel.conf", "--port", "27001"]
s2:
image: redis
container_name: s2
volumes:
- /opt/s2:/etc/redis
network_mode: "host"
entrypoint: ["redis-sentinel", "/etc/redis/sentinel.conf", "--port", "27002"]
s3:
image: redis
container_name: s3
volumes:
- /opt/s3:/etc/redis
network_mode: "host"
entrypoint: ["redis-sentinel", "/etc/redis/sentinel.conf", "--port", "27003"]
哨兵配置文件
powershell
# 设置 Sentinel 公网 IP,其他 Sentinel 会通过此 IP 来发现该 Sentinel 节点
sentinel announce-ip 192.168.1.100
# 监控 Redis 主节点,配置主节点的 IP 地址 (192.168.1.100) 和端口 (7002)
# "app-name" 是监控的主节点名称,2 表示至少需要 2 个 Sentinel 来判断主节点下线
sentinel monitor app-name 192.168.1.100 7002 2
# 设置 Sentinel 检测主节点是否宕机的超时时间 (单位:毫秒)
# 如果 Sentinel 在 5000 毫秒内未收到主节点的回复,将认为主节点宕机
sentinel down-after-milliseconds app-name 5000
# 设置故障转移的超时时间 (单位:毫秒)
# 如果在 60000 毫秒(即 60 秒)内没有完成故障转移,Sentinel 会中止当前操作
sentinel failover-timeout app-name 60000
# 设置 Sentinel 与 Redis 主节点之间的密码认证
# 如果 Redis 主节点启用了密码保护,则 Sentinel 需要使用这个密码来连接并与主节点进行通信
sentinel auth-pass app-name yourpassword
构建
powershell
docker-compose up -d
SpringBoot读写分离
yaml
yaml
spring:
redis:
sentinel:
master: "hmaster" # 主节点名称
nodes:
- "123.6.247.215:27001" # Sentinel 1 地址
- "123.6.247.215:27002" # Sentinel 2 地址
- "123.6.247.215:27003" # Sentinel 3 地址
password: "yourpassword" # 如果 Redis 配置了密码
#方便查询关于Redis的日志
logging:
level:
io.lettuce.core: debug
配置读写分离
java
@Configuration
public class RedisConfig {
@Bean
public LettuceClientConfigurationBuilderCustomizer lettuceclientConfigurationBuilderCustomizer(){
return clientConfigurationBuilder -> clientConfigurationBuilder.readFrom(ReadFrom.REPLICA_PREFERRED).commandTimeout(Duration.ofSeconds(5));
// 设置连接超时,避免连接问题;
}
}
- MASTER: 从主节点读取
- MASTER_PREFERRED: 优先从master节点读取,master不可用才读取replica
- REPLICA: 从slave(replica)节点读取
- REPLICA_PREFERRED: 优先从slave(replica)节点读取,所有的slave都不可用才读取master
接口
java
@RestController
@RequiredArgsConstructor
public class TestController
{
private final StringRedisTemplate stringRedisTemplate;
@GetMapping("/t")
public String test(){
stringRedisTemplate.opsForValue().set("name", "dpc", Duration.ofSeconds(10L));
return "成功";
}
@GetMapping("/g")
public String get(){
String str = stringRedisTemplate.opsForValue().get("name");
return str;
}
}
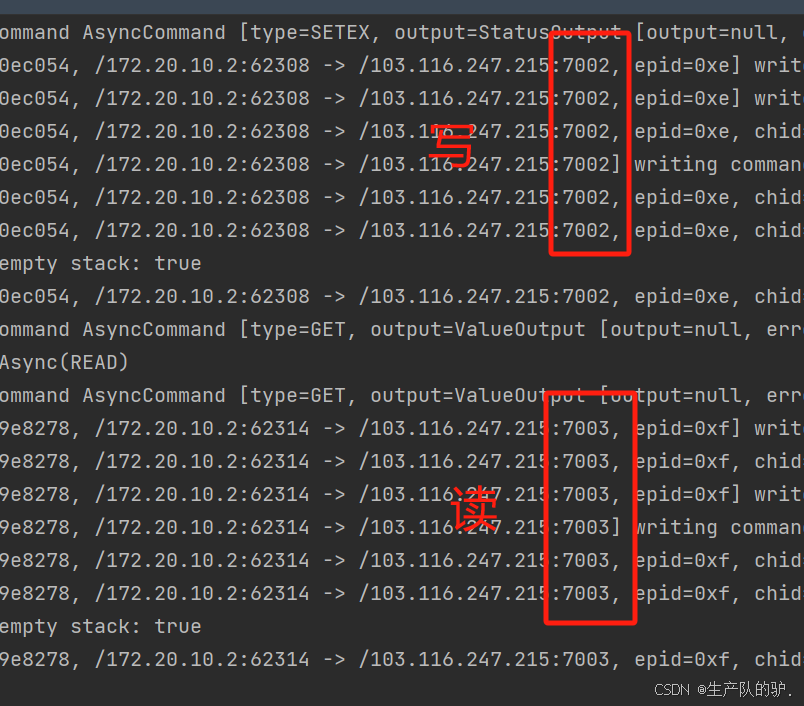