一、leetcode 209. 长度最小的子数组
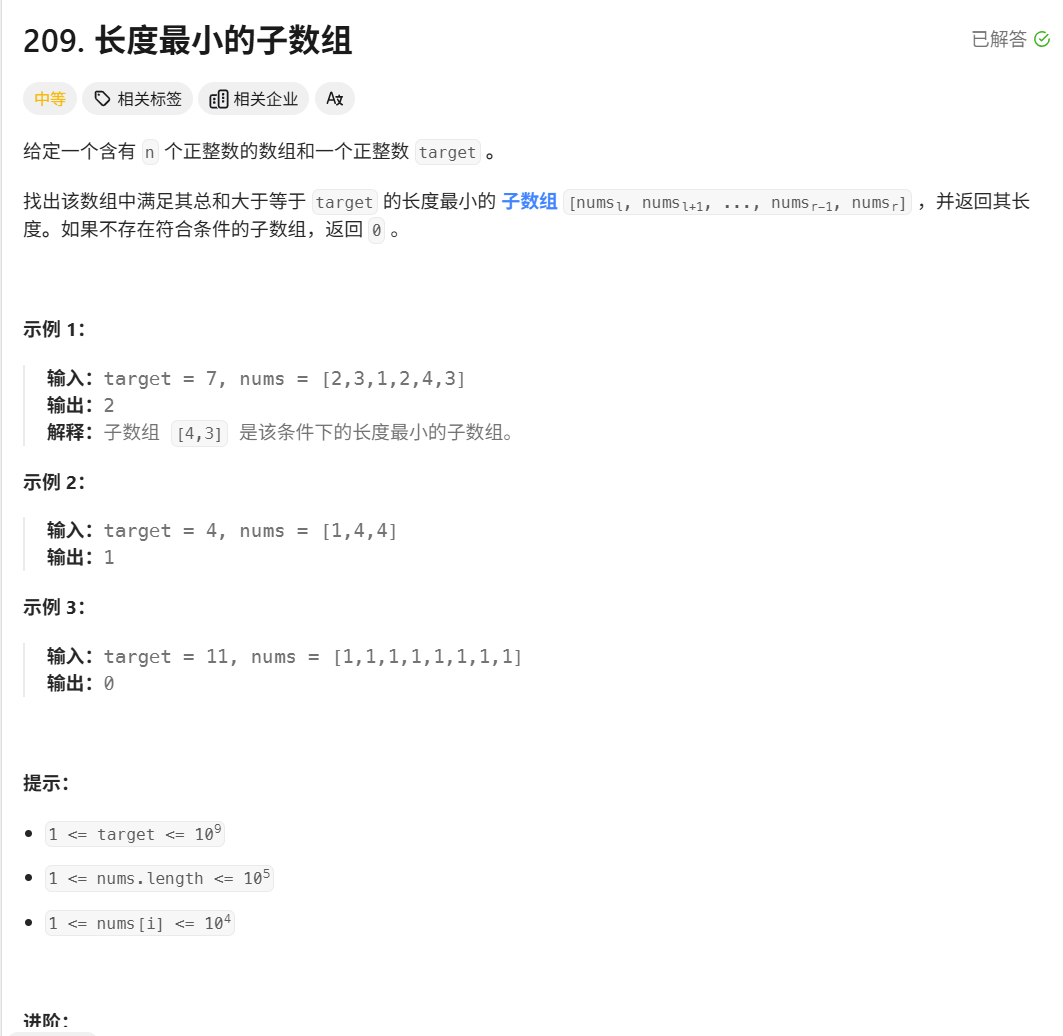
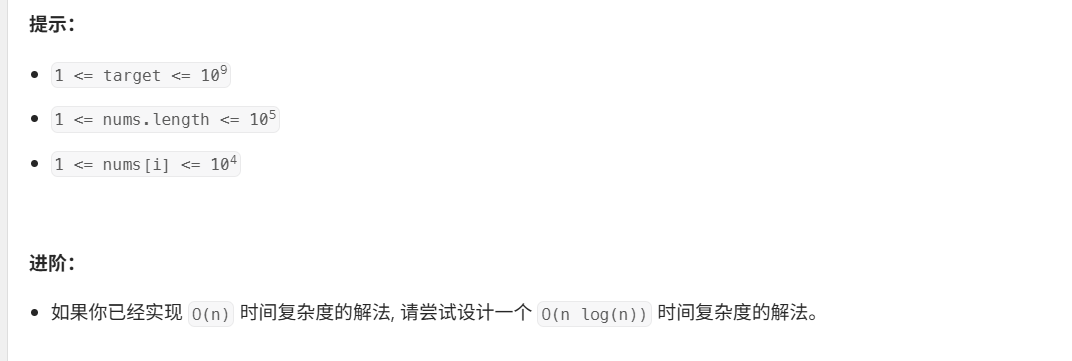
代码:
cpp
class Solution {
public:
int minSubArrayLen(int target, vector<int>& nums) {
int n = nums.size();
int left = 0;
int sum = 0;
int res = 100001;
for(int right = 0;right <n;right++){
sum += nums[right];
while(sum >= target){
res = min(res,right-left+1);
sum -= nums[left];
left++;
}
}
if(res == 100001)
res = 0;
return res;
}
};
二、leetcode76. 最小覆盖子串
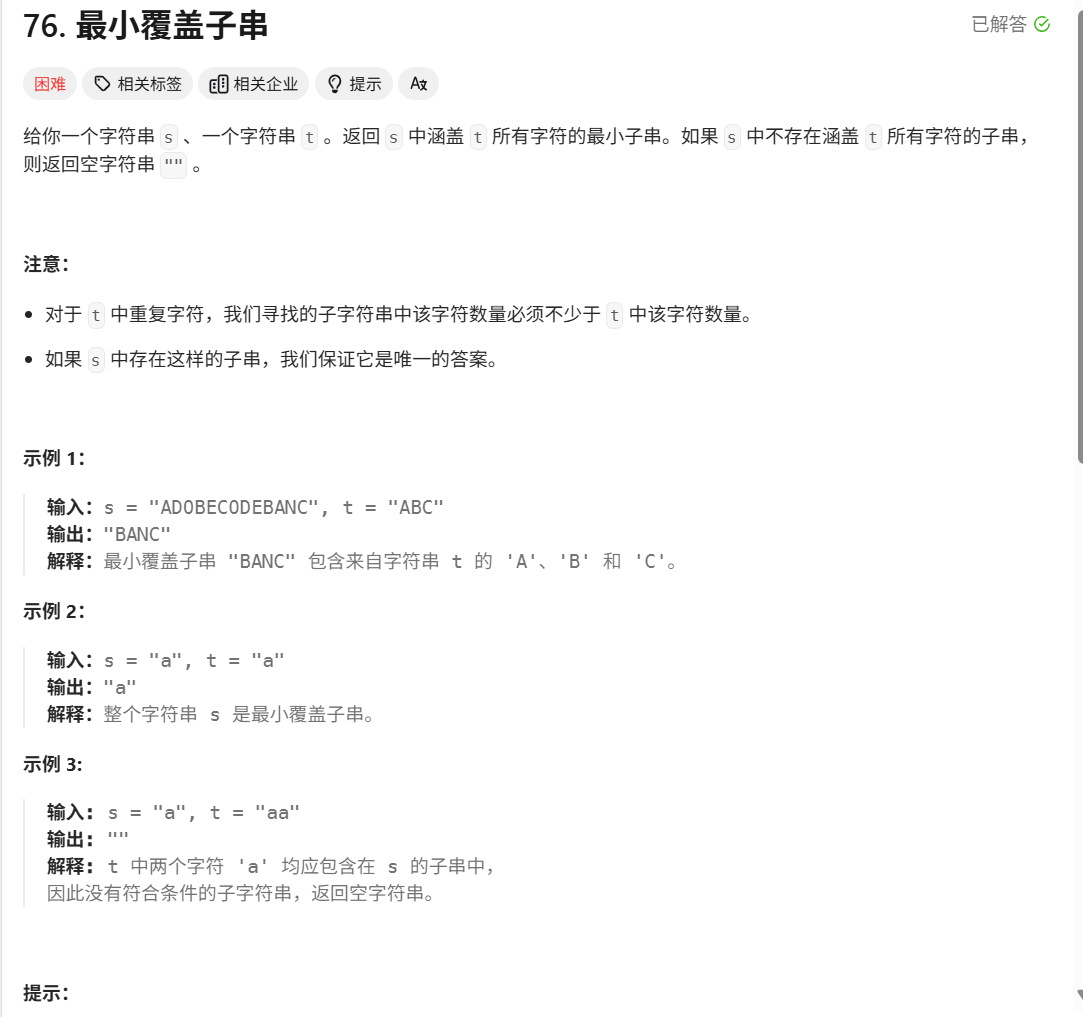
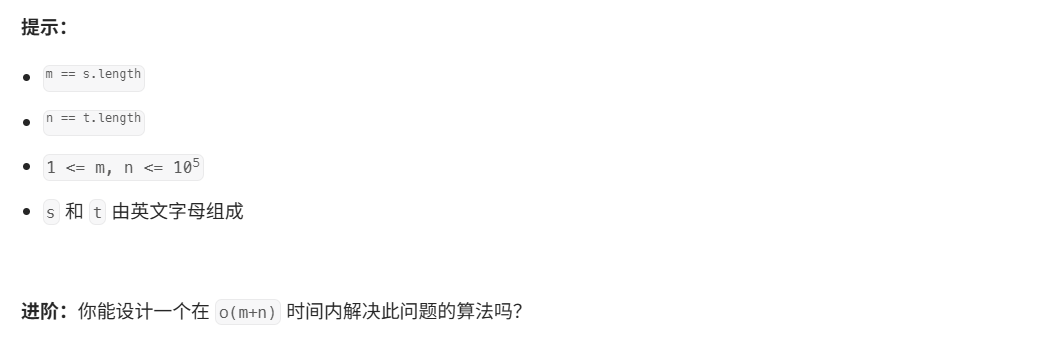
代码:
cpp
class Solution {
public:
string minWindow(string s, string t) {
unordered_map<char,int> t_map;
unordered_map<char,int> window_map;
for(char ch:t){
if(t_map.contains(ch)){
t_map[ch]++;
}else{
t_map[ch] = 1;
}
window_map[ch] = 0;
}
int s_len = s.size();
int left = 0;
int ans_left = -1;
int ans_len = INT_MAX;
for(int right = 0; right <s_len;right++){
if(!window_map.contains(s[right]))
continue;
window_map[s[right]]++;
while(check(t_map,window_map) && left <= right){
if(right -left+1 <ans_len){
ans_len = right -left +1;
ans_left = left;
}
if(t_map.contains(s[left]))
window_map[s[left]]--;
left++;
}
}
if(ans_left == -1) return "";
return s.substr(ans_left,ans_len);
}
bool check(unordered_map<char,int>& t_map,unordered_map<char,int>& window_map){
for(auto p:t_map){
if(window_map[p.first] < t_map[p.first])
return false;
}
return true;
}
};
cpp
class Solution {
public:
string minWindow(string s, string t) {
unordered_map<char,int> t_map;
unordered_map<char,int> window_map;
for(char ch:t){
if(t_map.contains(ch))
t_map[ch]++;
else
t_map[ch] = 1;
}
int s_len = s.size();
int left = 0;
int window_valid_char_cnt = 0;
string ans;
for(int right = 0; right <s_len;right++){
if(++window_map[s[right]] <= t_map[s[right]])
window_valid_char_cnt++;
while(window_map[s[left]] > t_map[s[left]]){
window_map[s[left]]--;
left++;
}
if(window_valid_char_cnt == t.size()){
if(ans.empty() || ans.size() > right-left+1)
ans = s.substr(left,right-left+1);
}
}
return ans;
}
};