《深入浅出WPF》读书笔记.10资源
背景
这一章主要讲资源,包括StaticResource,DynamicResource,以及二进制资源等等
资源
ResourceDictionary
资源对象为object类型,需要自己进行类型转换
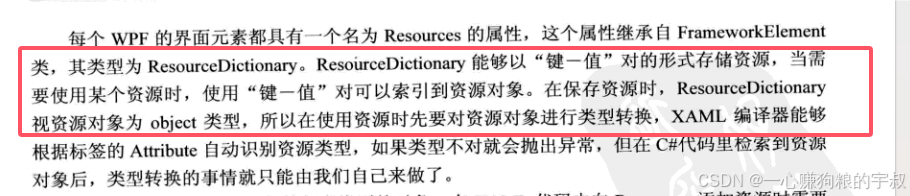
cs
<Window x:Class="ResourceDemo.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:ResourceDemo"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800" Loaded="Window_Loaded">
<Window.Resources>
<ResourceDictionary>
<sys:String x:Key="str1">沉舟侧畔千帆过</sys:String>
<sys:Double x:Key="db1">3.14</sys:Double>
</ResourceDictionary>
</Window.Resources>
<Grid>
<StackPanel>
<TextBox Height="40" Text="{StaticResource ResourceKey=str1}" ></TextBox>
<TextBox Height="40" x:Name="tb2"></TextBox>
<!--<TextBox Height="40" Text="{StaticResource ResourceKey=db1}"></TextBox>-->
</StackPanel>
</Grid>
</Window>
可以使用资源设置样式
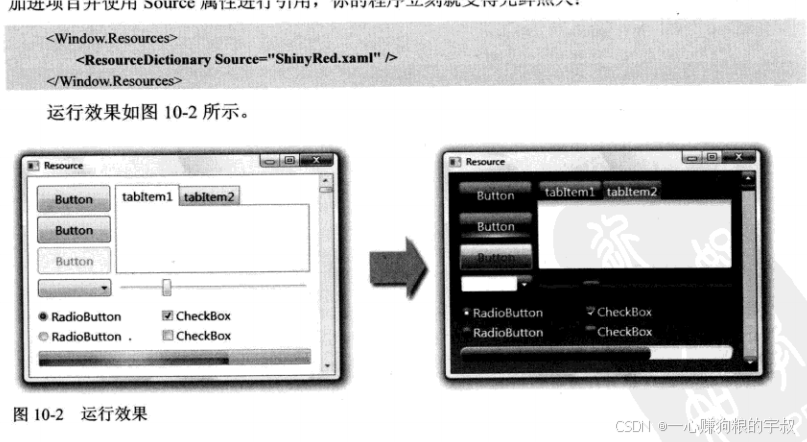
StaticResource和DynamicResource的区别
StaticResource:程序初始化只使用一次
DynamicResource:程序在运行中也在不断使用时
cs
<Window x:Class="ResourceDemo.DynamicAndStaticDemo"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:ResourceDemo"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
mc:Ignorable="d"
Title="DynamicAndStaticDemo" Height="300" Width="400">
<Window.Resources>
<ResourceDictionary>
<sys:String x:Key="str1">沉舟侧畔千帆过</sys:String>
<sys:String x:Key="str2">病树前头万木春</sys:String>
</ResourceDictionary>
</Window.Resources>
<Grid>
<StackPanel >
<Button x:Name="btn1" Content="{StaticResource str1}" Margin="5"></Button>
<Button x:Name="btn2" Content="{DynamicResource str2}" Margin="5"></Button>
<!--二进制文件资源-->
<Button x:Name="btn4" Content="{x:Static local:Resource1.str1}" Margin="5"></Button>
<Button x:Name="btn3" Content="Update" Margin="5" Click="btn3_Click"></Button>
<StackPanel Height="100">
<Image x:Name="img1" Stretch="Fill"></Image>
<!--<Image x:Name="img1" Source="pack://application:,,,/ResourceImage/screen1.png" Stretch="Fill"></Image>-->
<!--<Image Source="ResourceImage/screen1.png" Stretch="Fill"></Image>-->
</StackPanel>
</StackPanel>
</Grid>
</Window>
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
namespace ResourceDemo
{
/// <summary>
/// DynamicAndStaticDemo.xaml 的交互逻辑
/// </summary>
public partial class DynamicAndStaticDemo : Window
{
public DynamicAndStaticDemo()
{
InitializeComponent();
//使用uri加载资源文件
Uri uri = new Uri(@"pack://application:,,,/ResourceImage/screen1.png", UriKind.Absolute);
//Uri uri = new Uri(@"/ResourceImage/screen1.png", UriKind.Relative);
this.img1.Source=new BitmapImage(uri);
}
private void btn3_Click(object sender, RoutedEventArgs e)
{
this.Resources["str1"] = new TextBlock() { Text = "ABCD" };
this.Resources["str2"] = new TextBlock() { Text = "efgh" };
//代码中访问二进制资源
string str3 = Resource1.str1;
}
}
}
二进制资源
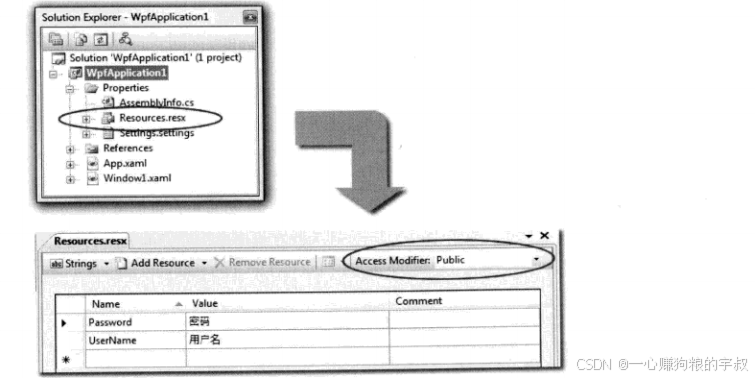
修改资源文件的权限
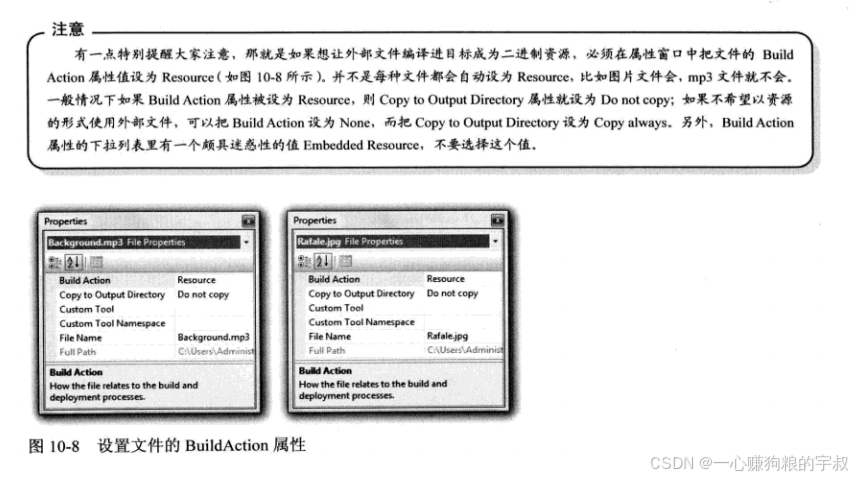
修改生成类型
使用Pack URI访问资源文件
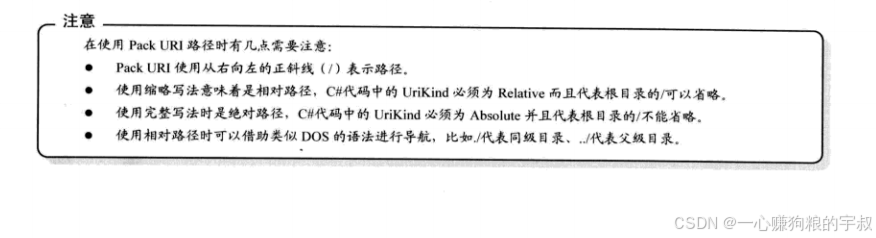
cs
Uri uri = new Uri(@"pack://application:,,,/ResourceImage/screen1.png", UriKind.Absolute);
//Uri uri = new Uri(@"/ResourceImage/screen1.png", UriKind.Relative);
this.img1.Source=new BitmapImage(uri);
cs
<!--<Image x:Name="img1" Source="pack://application:,,,/ResourceImage/screen1.png" Stretch="Fill"></Image>-->
<!--<Image Source="ResourceImage/screen1.png" Stretch="Fill"></Image>-->